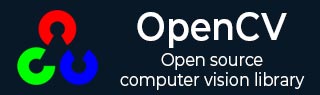
- OpenCV 教程
- OpenCV - 首页
- OpenCV - 概述
- OpenCV - 环境配置
- OpenCV - 图片存储
- OpenCV - 图片读取
- OpenCV - 图片写入
- OpenCV - 图形用户界面 (GUI)
- 绘图函数
- OpenCV - 绘制圆形
- OpenCV - 绘制直线
- OpenCV - 绘制矩形
- OpenCV - 绘制椭圆
- OpenCV - 绘制多边形
- OpenCV - 绘制凸多边形
- OpenCV - 绘制带箭头的直线
- OpenCV - 添加文本
- 滤波
- OpenCV - 双边滤波
- OpenCV - 方框滤波
- OpenCV - 平方盒滤波
- OpenCV - Filter2D
- OpenCV - 膨胀
- OpenCV - 腐蚀
- OpenCV - 形态学操作
- OpenCV - 图像金字塔
- Sobel 算子
- OpenCV - Sobel 算子
- OpenCV - Scharr 算子
- 摄像头和人脸检测
- OpenCV - 使用摄像头
- OpenCV - 图片中的人脸检测
- 使用摄像头进行人脸检测
- OpenCV 有用资源
- OpenCV - 快速指南
- OpenCV - 有用资源
- OpenCV - 讨论
OpenCV - 自适应阈值
在**简单阈值化**中,阈值是全局的,即图像中所有像素的阈值相同。**自适应阈值化**是一种针对较小区域计算阈值的方法,因此不同区域将具有不同的阈值。
在 OpenCV 中,可以使用 `Imgproc` 类的 `adaptiveThreshold()` 方法对图像执行自适应阈值操作。以下是此方法的语法。
adaptiveThreshold(src, dst, maxValue, adaptiveMethod, thresholdType, blockSize, C)
此方法接受以下参数:
**src** - `Mat` 类对象,表示源(输入)图像。
**dst** - `Mat` 类对象,表示目标(输出)图像。
**maxValue** - 双精度型变量,表示如果像素值大于阈值则要赋予的值。
**adaptiveMethod** - 整型变量,表示要使用的自适应方法。这将是以下两个值之一:
**ADAPTIVE_THRESH_MEAN_C** - 阈值是邻域区域的均值。
**ADAPTIVE_THRESH_GAUSSIAN_C** - 阈值是邻域值的加权和,权重为高斯窗口。
**thresholdType** - 整型变量,表示要使用的阈值类型。
**blockSize** - 整型变量,表示用于计算阈值的像素邻域的大小。
**C** - 双精度型变量,表示两种方法中使用的常数(从均值或加权均值中减去)。
示例
以下程序演示如何在 OpenCV 中对图像执行自适应阈值操作。这里我们选择类型为**二值化**的`adaptiveThreshold`以及`ADAPTIVE_THRESH_MEAN_C`作为阈值方法。
import org.opencv.core.Core; import org.opencv.core.Mat; import org.opencv.imgcodecs.Imgcodecs; import org.opencv.imgproc.Imgproc; public class AdaptiveThresh { public static void main(String args[]) throws Exception { // Loading the OpenCV core library System.loadLibrary( Core.NATIVE_LIBRARY_NAME ); // Reading the Image from the file and storing it in to a Matrix object String file ="E:/OpenCV/chap14/thresh_input.jpg"; // Reading the image Mat src = Imgcodecs.imread(file,0); // Creating an empty matrix to store the result Mat dst = new Mat(); Imgproc.adaptiveThreshold(src, dst, 125, Imgproc.ADAPTIVE_THRESH_MEAN_C, Imgproc.THRESH_BINARY, 11, 12); // Writing the image Imgcodecs.imwrite("E:/OpenCV/chap14/Adaptivemean_thresh_binary.jpg", dst); System.out.println("Image Processed"); } }
假设上面程序中指定的是输入图像 `thresh_input.jpg`。
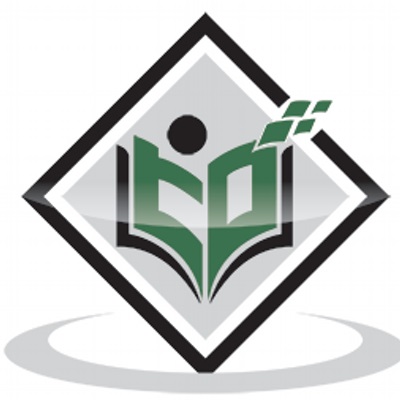
输出
执行程序后,您将获得以下输出:
Image Processed
如果您打开指定的路径,您可以观察到输出图像如下:
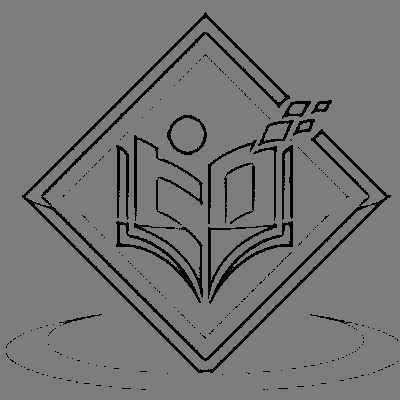
其他类型的自适应阈值化
除了前面示例中演示的 `ADAPTIVE_THRESH_MEAN_C` 作为自适应方法和 `THRESH_BINARY` 作为阈值类型之外,我们还可以选择这两种值的更多组合。
Imgproc.adaptiveThreshold(src, dst, 125, Imgproc.ADAPTIVE_THRESH_MEAN_C, Imgproc.THRESH_BINARY, 11, 12);
以下是表示 `adaptiveMethod` 和 `thresholdType` 参数各种值组合及其各自输出的值。
adaptiveMethod / thresholdType | ADAPTIVE_THRESH_MEAN_C | ADAPTIVE_THRESH_GAUSSIAN_C |
---|---|---|
THRESH_BINARY | ![]() |
![]() |
THRESH_BINARY_INV | ![]() |
![]() |