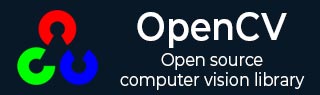
- OpenCV 教程
- OpenCV - 首页
- OpenCV - 概述
- OpenCV - 环境配置
- OpenCV - 图像存储
- OpenCV - 读取图像
- OpenCV - 写入图像
- OpenCV - 图形用户界面 (GUI)
- 绘图函数
- OpenCV - 绘制圆形
- OpenCV - 绘制直线
- OpenCV - 绘制矩形
- OpenCV - 绘制椭圆
- OpenCV - 绘制多边形
- OpenCV - 绘制凸多边形
- OpenCV - 绘制带箭头的直线
- OpenCV - 添加文本
- 滤波
- OpenCV - 双边滤波
- OpenCV - 方框滤波
- OpenCV - 平方盒滤波器
- OpenCV - Filter2D
- OpenCV - 膨胀
- OpenCV - 腐蚀
- OpenCV - 形态学操作
- OpenCV - 图像金字塔
- Sobel 算子
- OpenCV - Sobel 算子
- OpenCV - Scharr 算子
- 图像变换操作
- OpenCV - 拉普拉斯变换
- OpenCV - 距离变换
- 相机和人脸检测
- OpenCV - 使用相机
- OpenCV - 图片中的人脸检测
- 使用相机进行人脸检测
- OpenCV 有用资源
- OpenCV - 快速指南
- OpenCV - 有用资源
- OpenCV - 讨论
OpenCV - 图像金字塔
金字塔是对图像的一种操作,其中:
输入图像首先使用特定的平滑滤波器(例如:高斯滤波器、拉普拉斯滤波器)进行平滑处理,然后对平滑后的图像进行子采样。
此过程重复多次。
在金字塔操作过程中,图像的平滑度增加,而分辨率(大小)减小。
向上金字塔
在向上金字塔中,图像首先进行上采样,然后进行模糊处理。您可以使用`imgproc`类的`pyrUP()`方法对图像执行向上金字塔操作。以下是此方法的语法:
pyrUp(src, dst, dstsize, borderType)
此方法接受以下参数:
src - `Mat`类的一个对象,表示源(输入)图像。
dst - `Mat`类的一个对象,表示目标(输出)图像。
size - `Size`类的一个对象,表示图像要增加或减小的尺寸。
borderType - 整型变量,表示要使用的边界类型。
示例
以下程序演示了如何在图像上执行向上金字塔操作。
import org.opencv.core.Core; import org.opencv.core.Mat; import org.opencv.core.Size; import org.opencv.imgcodecs.Imgcodecs; import org.opencv.imgproc.Imgproc; public class PyramidUp { public static void main( String[] args ) { // Loading the OpenCV core library System.loadLibrary( Core.NATIVE_LIBRARY_NAME ); // Reading the Image from the file and storing it in to a Matrix object String file ="E:/OpenCV/chap13/pyramid_input.jpg"; Mat src = Imgcodecs.imread(file); // Creating an empty matrix to store the result Mat dst = new Mat(); // Applying pyrUp on the Image Imgproc.pyrUp(src, dst, new Size(src.cols()*2, src.rows()*2), Core.BORDER_DEFAULT); // Writing the image Imgcodecs.imwrite("E:/OpenCV/chap13/pyrUp_output.jpg", dst); System.out.println("Image Processed"); } }
假设上述程序中指定了以下输入图像`pyramid_input.jpg`。
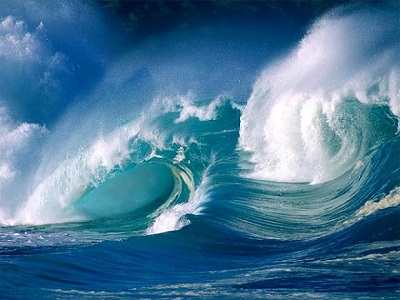
输出
执行程序后,您将获得以下输出:
Image Processed
如果打开指定的路径,您可以观察到输出图像如下:
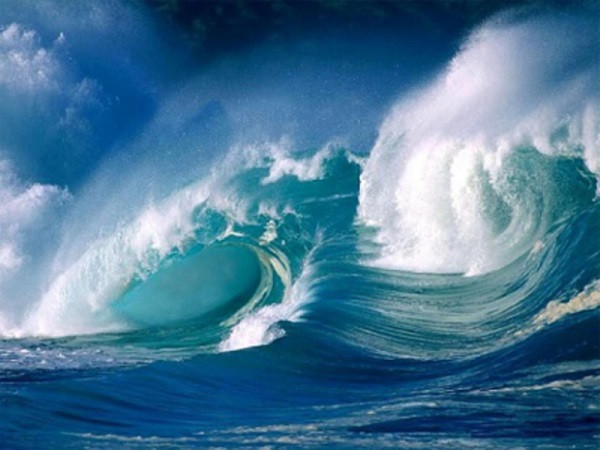
向下金字塔
在向下金字塔中,图像首先进行模糊处理,然后进行下采样。您可以使用`imgproc`类的`pyrDown()`方法对图像执行向下金字塔操作。以下是此方法的语法:
pyrDown(src, dst, dstsize, borderType)
此方法接受以下参数:
src - `Mat`类的一个对象,表示源(输入)图像。
dst - `Mat`类的一个对象,表示目标(输出)图像。
size - `Size`类的一个对象,表示图像要增加或减小的尺寸。
borderType - 整型变量,表示要使用的边界类型。
示例
以下程序演示了如何在图像上执行向下金字塔操作。
import org.opencv.core.Core; import org.opencv.core.Mat; import org.opencv.core.Size; import org.opencv.imgcodecs.Imgcodecs; import org.opencv.imgproc.Imgproc; public class PyramidDown { public static void main( String[] args ) { // Loading the OpenCV core library System.loadLibrary( Core.NATIVE_LIBRARY_NAME ); // Reading the Image from the file and storing it in to a Matrix object String file ="E:/OpenCV/chap13/pyramid_input.jpg"; Mat src = Imgcodecs.imread(file); // Creating an empty matrix to store the result Mat dst = new Mat(); // Applying pyrDown on the Image Imgproc.pyrDown(src, dst, new Size(src.cols()/2, src.rows()/2), Core.BORDER_DEFAULT); // Writing the image Imgcodecs.imwrite("E:/OpenCV/chap13/pyrDown_output.jpg", dst); System.out.println("Image Processed"); } }
假设上述程序中指定了以下输入图像`pyramid_input.jpg`。
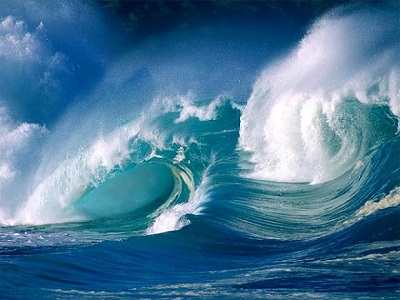
输出
执行程序后,您将获得以下输出:
Image Processed
如果打开指定的路径,您可以观察到输出图像如下:
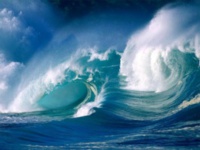
均值漂移滤波
在均值漂移金字塔操作中,首先对图像进行均值漂移分割。
您可以使用`imgproc`类的`pyrMeanShiftFiltering()`方法对图像执行均值漂移滤波操作。以下是此方法的语法。
pyrMeanShiftFiltering(src, dst, sp, sr)
此方法接受以下参数:
src - `Mat`类的一个对象,表示源(输入)图像。
dst - `Mat`类的一个对象,表示目标(输出)图像。
sp - 双精度型变量,表示空间窗口半径。
sr - 双精度型变量,表示颜色窗口半径。
示例
以下程序演示了如何在给定图像上执行均值漂移滤波操作。
import org.opencv.core.Core; import org.opencv.core.Mat; import org.opencv.imgcodecs.Imgcodecs; import org.opencv.imgproc.Imgproc; public class PyramidMeanShift { public static void main( String[] args ) { // Loading the OpenCV core library System.loadLibrary( Core.NATIVE_LIBRARY_NAME ); // Reading the Image from the file and storing it in to a Matrix object String file ="E:/OpenCV/chap13/pyramid_input.jpg"; Mat src = Imgcodecs.imread(file); // Creating an empty matrix to store the result Mat dst = new Mat(); // Applying meanShifting on the Image Imgproc.pyrMeanShiftFiltering(src, dst, 200, 300); // Writing the image Imgcodecs.imwrite("E:/OpenCV/chap13/meanShift_output.jpg", dst); System.out.println("Image Processed"); } }
假设上述程序中指定了以下输入图像`pyramid_input.jpg`。
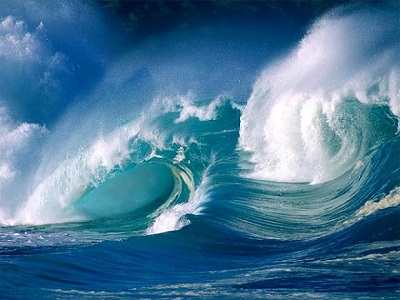
输出
执行程序后,您将获得以下输出:
Image Processed
如果打开指定的路径,您可以观察到输出图像如下:
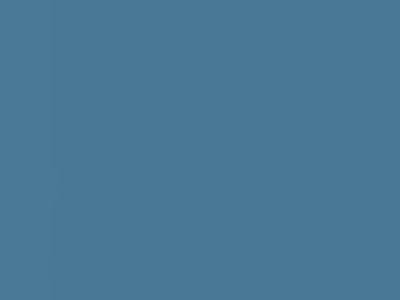