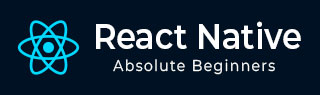
- React Native 教程
- React Native - 主页
- 核心概念
- React Native - 概述
- React Native - 环境设置
- React Native - 应用
- React Native - 状态
- React Native - 属性
- React Native - 样式
- React Native - Flexbox
- React Native - ListView
- React Native - 文本输入
- React Native - ScrollView
- React Native - 图片
- React Native - HTTP
- React Native - 按钮
- React Native - 动画
- React Native - 调试
- React Native - 路由
- React Native - 运行 IOS
- React Native - 运行 Android
- 组件和 API
- React Native - View
- React Native - WebView
- React Native - Modal
- React Native - ActivityIndicator
- React Native - Picker
- React Native - 状态栏
- React Native - Switch
- React Native - Text
- React Native - Alert
- React Native - 地理位置
- React Native - AsyncStorage
- React Native 实用资源
- React Native - 快速指南
- React Native - 实用资源
- React Native - 讨论
React Native - 按钮
在本文档中,我们将向您展示 react Native 中的可触摸组件。我们将它们称为“可触摸”,因为它们可提供内置动画效果,并且我们可以使用 onPress 属性来处理触摸事件。
Facebook 提供了 Button 组件,可用作通用按钮。考虑以下示例来理解这一点。
App.js
import React, { Component } from 'react' import { Button } from 'react-native' const App = () => { const handlePress = () => false return ( <Button onPress = {handlePress} title = "Red button!" color = "red" /> ) } export default App
如果默认 Button 组件无法满足您的需求,您可以使用以下组件之一。
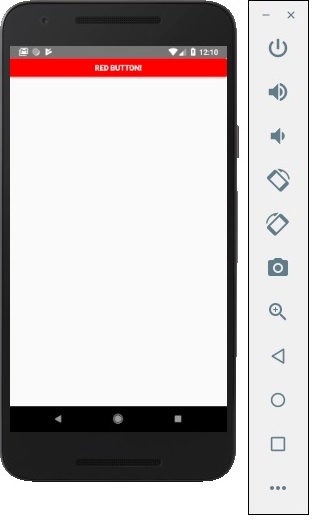
Touchable Opacity
当触摸该元素时,此元素将更改元素的不透明度。
App.js
import React from 'react' import { TouchableOpacity, StyleSheet, View, Text } from 'react-native' const App = () => { return ( <View style = {styles.container}> <TouchableOpacity> <Text style = {styles.text}> Button </Text> </TouchableOpacity> </View> ) } export default App const styles = StyleSheet.create ({ container: { alignItems: 'center', }, text: { borderWidth: 1, padding: 25, borderColor: 'black', backgroundColor: 'red' } })
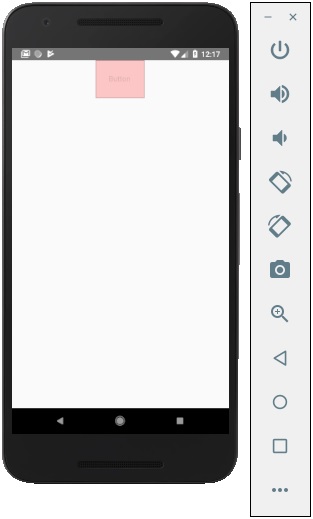
Touchable Highlight
当用户按下元素时,它将变暗并且底色将显现。
App.js
import React from 'react' import { View, TouchableHighlight, Text, StyleSheet } from 'react-native' const App = (props) => { return ( <View style = {styles.container}> <TouchableHighlight> <Text style = {styles.text}> Button </Text> </TouchableHighlight> </View> ) } export default App const styles = StyleSheet.create ({ container: { alignItems: 'center', }, text: { borderWidth: 1, padding: 25, borderColor: 'black', backgroundColor: 'red' } })
Touchable Native Feedback
当按下元素时,这将模拟墨水动画效果。
App.js
import React from 'react' import { View, TouchableNativeFeedback, Text, StyleSheet } from 'react-native' const Home = (props) => { return ( <View style = {styles.container}> <TouchableNativeFeedback> <Text style = {styles.text}> Button </Text> </TouchableNativeFeedback> </View> ) } export default Home const styles = StyleSheet.create ({ container: { alignItems: 'center', }, text: { borderWidth: 1, padding: 25, borderColor: 'black', backgroundColor: 'red' } })
Touchable Without Feedback
当您想在没有任何动画效果的情况下处理触摸事件时,应使用此元素。通常,此组件使用的并不多。
<TouchableWithoutFeedback> <Text> Button </Text> </TouchableWithoutFeedback>
广告