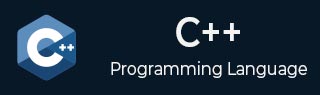
- C++ 基础
- C++ 首页
- C++ 概述
- C++ 环境设置
- C++ 基本语法
- C++ 注释
- C++ Hello World
- C++ 省略命名空间
- C++ 常量/字面量
- C++ 关键字
- C++ 标识符
- C++ 数据类型
- C++ 数值数据类型
- C++ 字符数据类型
- C++ 布尔数据类型
- C++ 变量类型
- C++ 变量作用域
- C++ 多个变量
- C++ 基本输入/输出
- C++ 修饰符类型
- C++ 存储类
- C++ 运算符
- C++ 数字
- C++ 枚举
- C++ 引用
- C++ 日期和时间
- C++ 控制语句
- C++ 决策制定
- C++ if 语句
- C++ if else 语句
- C++ 嵌套 if 语句
- C++ switch 语句
- C++ 嵌套 switch 语句
- C++ 循环类型
- C++ while 循环
- C++ for 循环
- C++ do while 循环
- C++ foreach 循环
- C++ 嵌套循环
- C++ break 语句
- C++ continue 语句
- C++ goto 语句
- C++ 构造函数
- C++ 构造函数和析构函数
- C++ 复制构造函数
C++ 注释
C++ 注释
程序注释是可以在 C++ 代码中包含的解释性语句。这些注释可以帮助任何阅读源代码的人。所有编程语言都允许某种形式的注释。
C++ 注释的类型
C++ 支持两种类型的注释:**单行注释**和**多行注释**。任何注释中可用的所有字符都被 C++ 编译器 忽略。
C++ 注释的类型将在下一节中详细解释
1. C++ 单行注释
单行注释以**//**开头,一直延伸到行尾。这些注释只能持续到行尾,下一行将开始一个新的注释。
语法
以下语法显示了如何在 C++ 中使用单行注释
// Text to be commented
示例
在以下示例中,我们正在创建单行注释 -
#include <iostream> using namespace std; int main() { // this is a single line comment cout << "Hello world!" << endl; // for a new line, we have to use new comment sections cout << "This is second line."; return 0; }
输出
Hello world! This is second line.
2. C++ 多行注释
多行注释以**/***开头,以***/**结尾。这些符号之间的任何文本都被视为注释。
语法
以下语法显示了如何在 C++ 中使用多行注释
/* This is a comment */ /* C++ comments can also span multiple lines */
示例
在以下示例中,我们正在创建多行注释 -
#include <iostream> using namespace std; int main() { /* Printing hello world!*/ cout << "Hello World!" << endl; /* This is a multi-line comment Printing another message Using cout */ cout << "Tutorials Point"; return 0; }
输出
Hello World! Tutorials Point
语句中的注释
我们也可以在 C++ 程序中的代码块内注释掉特定的语句。这两种类型的注释都可以做到这一点。
示例
以下示例说明了在语句中使用多行注释 -
#include <iostream> using namespace std; int main() { cout << "This line" /*what is this*/ << " contains a comment" << endl; return 0; }
输出
This line contains a comment
示例
以下示例说明了在语句中使用单行注释 -
#include <iostream> using namespace std; int main() { cout << "This line" // what is this << " contains a comment" << endl; return 0; }
输出
This line contains a comment
嵌套注释
在 /* 和 */ 注释中,// 字符没有特殊含义。在 // 注释中,/* 和 */ 没有特殊含义。因此,您可以将一种注释“嵌套”在另一种注释中。
示例
以下示例说明了使用嵌套在注释中的注释 -
#include <iostream> using namespace std; int main() { /* Comment out printing of Hello World: cout << "Hello World"; // prints Hello World */ cout << "New, Hello World!"; return 0; }
输出
New, Hello World!
单行或多行注释 - 何时使用?
单行注释通常用于一般的短行注释。这在我们需要在代码中为算法提供一个小提示时很常见。
多行注释通常用于较长的注释行,在需要整个注释行的可见性时。注释越长,多行注释所需的语句就越多。
注释的目的
注释在 C++ 中用于各种目的。注释的一些主要应用领域如下所示
- 表示程序中的一个简短且简洁的步骤,以便用户更好地理解。
- 详细解释代码中未明确表达的步骤。
- 为用户在代码本身中提供不同的提示。
- 为了娱乐或消遣留下注释。
- 为了调试目的暂时禁用代码的一部分。
- 为代码添加元数据以备将来使用。
- 为代码创建文档,例如在 Github 页面中。
广告