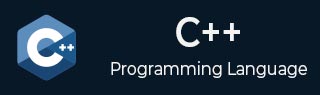
- C++ 基础
- C++ 首页
- C++ 概述
- C++ 环境设置
- C++ 基本语法
- C++ 注释
- C++ Hello World
- C++ 省略命名空间
- C++ 常量/字面量
- C++ 关键字
- C++ 标识符
- C++ 数据类型
- C++ 数值数据类型
- C++ 字符数据类型
- C++ 布尔数据类型
- C++ 变量类型
- C++ 变量作用域
- C++ 多个变量
- C++ 基本输入/输出
- C++ 修饰符类型
- C++ 存储类
- C++ 运算符
- C++ 数字
- C++ 枚举
- C++ 引用
- C++ 日期和时间
- C++ 控制语句
- C++ 决策
- C++ if 语句
- C++ if else 语句
- C++ 嵌套 if 语句
- C++ switch 语句
- C++ 嵌套 switch 语句
- C++ 循环类型
- C++ while 循环
- C++ for 循环
- C++ do while 循环
- C++ foreach 循环
- C++ 嵌套循环
- C++ break 语句
- C++ continue 语句
- C++ goto 语句
- C++ 构造函数
- C++ 构造函数和析构函数
- C++ 复制构造函数
C++ 类中的静态成员
类的静态成员
我们可以使用static关键字定义类的静态成员。当我们将类的成员声明为静态时,这意味着无论创建了多少个类的对象,静态成员都只有一份拷贝。
静态成员由类的所有对象共享。如果不存在其他初始化,则所有静态数据在创建第一个对象时初始化为零。我们不能将它放在类定义中,但可以在类外初始化它,如下例所示,通过重新声明静态变量,使用作用域解析运算符::来标识它属于哪个类。
示例
让我们尝试以下示例来理解静态数据成员的概念:
#include <iostream> using namespace std; class Box { public: static int objectCount; // Constructor definition Box(double l = 2.0, double b = 2.0, double h = 2.0) { cout <<"Constructor called." << endl; length = l; breadth = b; height = h; // Increase every time object is created objectCount++; } double Volume() { return length * breadth * height; } private: double length; // Length of a box double breadth; // Breadth of a box double height; // Height of a box }; // Initialize static member of class Box int Box::objectCount = 0; int main(void) { Box Box1(3.3, 1.2, 1.5); // Declare box1 Box Box2(8.5, 6.0, 2.0); // Declare box2 // Print total number of objects. cout << "Total objects: " << Box::objectCount << endl; return 0; }
编译并执行上述代码后,将产生以下结果:
Constructor called. Constructor called. Total objects: 2
静态函数成员
通过将函数成员声明为静态,使其独立于类的任何特定对象。即使不存在类的任何对象,也可以调用静态成员函数,并且static函数仅使用类名和作用域解析运算符::访问。
静态成员函数只能访问静态数据成员、其他静态成员函数以及类外部的任何其他函数。
静态成员函数具有类作用域,它们无法访问类的this指针。可以使用静态成员函数来确定是否已创建类的某些对象。
示例
让我们尝试以下示例来理解静态函数成员的概念:
#include <iostream> using namespace std; class Box { public: static int objectCount; // Constructor definition Box(double l = 2.0, double b = 2.0, double h = 2.0) { cout <<"Constructor called." << endl; length = l; breadth = b; height = h; // Increase every time object is created objectCount++; } double Volume() { return length * breadth * height; } static int getCount() { return objectCount; } private: double length; // Length of a box double breadth; // Breadth of a box double height; // Height of a box }; // Initialize static member of class Box int Box::objectCount = 0; int main(void) { // Print total number of objects before creating object. cout << "Inital Stage Count: " << Box::getCount() << endl; Box Box1(3.3, 1.2, 1.5); // Declare box1 Box Box2(8.5, 6.0, 2.0); // Declare box2 // Print total number of objects after creating object. cout << "Final Stage Count: " << Box::getCount() << endl; return 0; }
编译并执行上述代码后,将产生以下结果:
Inital Stage Count: 0 Constructor called. Constructor called. Final Stage Count: 2
静态成员的用例
以下是几个用例:
- 单例模式是一种设计模式,它确保类只有一个实例,并提供对其的全局访问点。这里的静态成员非常适合实现这种模式,因为它们允许维护类的单个共享实例。
- 静态成员通常用于管理跨类的所有实例共享的共享资源或计数器。
- 静态成员可用于存储全局配置设置或常量,这对于管理资源池(例如缓存、数据库连接池等)和实现跨实例共享的日志系统非常有用。
- 静态成员可用于跟踪方法调用。
广告