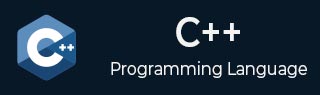
- C++ 基础
- C++ 首页
- C++ 概述
- C++ 环境设置
- C++ 基本语法
- C++ 注释
- C++ Hello World
- C++ 省略命名空间
- C++ 常量/字面量
- C++ 关键字
- C++ 标识符
- C++ 数据类型
- C++ 数值数据类型
- C++ 字符数据类型
- C++ 布尔数据类型
- C++ 变量类型
- C++ 变量作用域
- C++ 多个变量
- C++ 基本输入/输出
- C++ 修饰符类型
- C++ 存储类
- C++ 运算符
- C++ 数字
- C++ 枚举
- C++ 引用
- C++ 日期和时间
- C++ 控制语句
- C++ 决策
- C++ if 语句
- C++ if else 语句
- C++ 嵌套 if 语句
- C++ switch 语句
- C++ 嵌套 switch 语句
- C++ 循环类型
- C++ while 循环
- C++ for 循环
- C++ do while 循环
- C++ foreach 循环
- C++ 嵌套循环
- C++ break 语句
- C++ continue 语句
- C++ goto 语句
- C++ 构造函数
- C++ 构造函数和析构函数
- C++ 复制构造函数
C++ this 指针
this 指针
C++ 中的每个对象都可以通过一个称为this指针的重要指针访问其自身的地址。this指针是所有成员函数的隐式参数。因此,在成员函数内部,this 可以用来引用调用该函数的对象。
友元函数没有this指针,因为友元不是类的成员。只有成员函数才有this指针。
this 指针示例
让我们尝试以下示例来理解 this 指针的概念:
#include <iostream> using namespace std; class Box { public: // Constructor definition Box(double l = 2.0, double b = 2.0, double h = 2.0) { cout <<"Constructor called." << endl; length = l; breadth = b; height = h; } double Volume() { return length * breadth * height; } int compare(Box box) { return this->Volume() > box.Volume(); } private: double length; // Length of a box double breadth; // Breadth of a box double height; // Height of a box }; int main(void) { Box Box1(3.3, 1.2, 1.5); // Declare box1 Box Box2(8.5, 6.0, 2.0); // Declare box2 if(Box1.compare(Box2)) { cout << "Box2 is smaller than Box1" <<endl; } else { cout << "Box2 is equal to or larger than Box1" <<endl; } return 0; }
当以上代码编译并执行时,会产生以下结果:
Constructor called. Constructor called. Box2 is equal to or larger than Box1
使用 this 指针返回调用对象的引用
要实现链式函数调用,您需要调用对象的引用。您可以使用“this”指针返回调用对象的引用。
语法
以下是语法:
Test& Test::func () { return *this; }
示例
以下示例演示了如何返回对调用对象的引用
#include <iostream> using namespace std; class Coordinates { private: int latitude; int longitude; public: Coordinates(int lat = 0, int lon = 0) { this->latitude = lat; this->longitude = lon; } Coordinates& setLatitude(int lat) { latitude = lat; return *this; } Coordinates& setLongitude(int lon) { longitude = lon; return *this; } void display() const { cout << "Latitude = " << latitude << ", Longitude = " << longitude << endl; } }; int main() { Coordinates location(15, 30); // Chained function calls modifying the same object location.setLatitude(40).setLongitude(70); location.display(); return 0; }
当以上代码编译并执行时,会产生以下结果:
Latitude = 40, Longitude = 70
广告