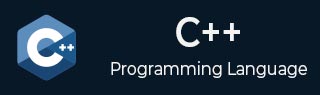
- C++ 基础
- C++ 首页
- C++ 概述
- C++ 环境设置
- C++ 基本语法
- C++ 注释
- C++ Hello World
- C++ 省略命名空间
- C++ 常量/字面量
- C++ 关键字
- C++ 标识符
- C++ 数据类型
- C++ 数值数据类型
- C++ 字符数据类型
- C++ 布尔数据类型
- C++ 变量类型
- C++ 变量作用域
- C++ 多个变量
- C++ 基本输入/输出
- C++ 修饰符类型
- C++ 存储类
- C++ 运算符
- C++ 数字
- C++ 枚举
- C++ 引用
- C++ 日期和时间
- C++ 控制语句
- C++ 决策
- C++ if 语句
- C++ if else 语句
- C++ 嵌套 if 语句
- C++ switch 语句
- C++ 嵌套 switch 语句
- C++ 循环类型
- C++ while 循环
- C++ for 循环
- C++ do while 循环
- C++ foreach 循环
- C++ 嵌套循环
- C++ break 语句
- C++ continue 语句
- C++ goto 语句
- C++ 构造函数
- C++ 构造函数和析构函数
- C++ 复制构造函数
C++ if...else 语句
一个if语句可以后跟一个可选的else语句,当布尔表达式为假时执行。
语法
C++ 中 if...else 语句的语法为:
if(boolean_expression) { // statement(s) will execute if the boolean expression is true } else { // statement(s) will execute if the boolean expression is false }
如果布尔表达式计算结果为true,则将执行if 块代码,否则将执行else 块代码。
流程图
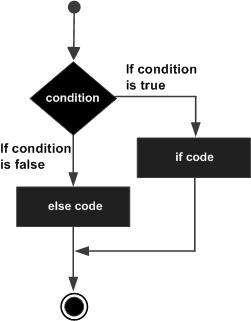
示例
#include <iostream> using namespace std; int main () { // local variable declaration: int a = 100; // check the boolean condition if( a < 20 ) { // if condition is true then print the following cout << "a is less than 20;" << endl; } else { // if condition is false then print the following cout << "a is not less than 20;" << endl; } cout << "value of a is : " << a << endl; return 0; }
编译并运行上述代码后,将产生以下结果:
a is not less than 20; value of a is : 100
if...else if...else 语句
一个if语句可以后跟一个可选的else if...else语句,这对于使用单个 if...else if 语句测试各种条件非常有用。
使用 if、else if、else 语句时,需要注意几点。
一个 if 语句可以有零个或一个 else,并且它必须位于任何 else if 之后。
一个 if 语句可以有零个到多个 else if,并且它们必须位于 else 之前。
一旦 else if 成功,就不会测试任何剩余的 else if 或 else。
语法
C++ 中 if...else if...else 语句的语法为:
if(boolean_expression 1) { // Executes when the boolean expression 1 is true } else if( boolean_expression 2) { // Executes when the boolean expression 2 is true } else if( boolean_expression 3) { // Executes when the boolean expression 3 is true } else { // executes when the none of the above condition is true. }
示例
#include <iostream> using namespace std; int main () { // local variable declaration: int a = 100; // check the boolean condition if( a == 10 ) { // if condition is true then print the following cout << "Value of a is 10" << endl; } else if( a == 20 ) { // if else if condition is true cout << "Value of a is 20" << endl; } else if( a == 30 ) { // if else if condition is true cout << "Value of a is 30" << endl; } else { // if none of the conditions is true cout << "Value of a is not matching" << endl; } cout << "Exact value of a is : " << a << endl; return 0; }
编译并运行上述代码后,将产生以下结果:
Value of a is not matching Exact value of a is : 100
广告