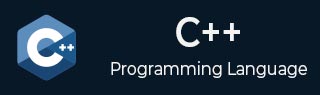
- C++ 基础
- C++ 首页
- C++ 概述
- C++ 环境设置
- C++ 基本语法
- C++ 注释
- C++ Hello World
- C++ 省略命名空间
- C++ 常量/字面量
- C++ 关键字
- C++ 标识符
- C++ 数据类型
- C++ 数值数据类型
- C++ 字符数据类型
- C++ 布尔数据类型
- C++ 变量类型
- C++ 变量作用域
- C++ 多个变量
- C++ 基本输入/输出
- C++ 修饰符类型
- C++ 存储类
- C++ 运算符
- C++ 数字
- C++ 枚举
- C++ 引用
- C++ 日期和时间
- C++ 控制语句
- C++ 决策制定
- C++ if 语句
- C++ if else 语句
- C++ 嵌套 if 语句
- C++ switch 语句
- C++ 嵌套 switch 语句
- C++ 循环类型
- C++ while 循环
- C++ for 循环
- C++ do while 循环
- C++ foreach 循环
- C++ 嵌套循环
- C++ break 语句
- C++ continue 语句
- C++ goto 语句
- C++ 构造函数
- C++ 构造函数和析构函数
- C++ 复制构造函数
C++ 中的返回语句
C++ 中的 **return** 语句用于退出函数并将值(可选)发送回函数的调用者,这取决于需求。它在控制程序流程和确保函数向代码的其他部分提供结果方面起着非常重要的作用。
语法
以下是 C++ 中使用 return 语句的语法:
return [expression];
其中,“**表达式**”是可选的,专门用于函数。如果提供,它指定要返回给调用者的值。
return 语句示例
以下是 return 语句的示例:
#include <iostream> using namespace std; int sum(int a, int b){ // Here, returning sum of a and b return a + b; } int main(){ // Calling the function int ans = sum(5, 2); cout << "The sum of two integers 5 and 2 is: " << ans << endl; // Returning from the main (), // 0 represents execution done without any error return 0; }
输出
The sum of two integers 5 and 2 is: 7
return 语句的关键方面
1. 函数终止
执行 return 语句时,函数会立即退出,并可选地将值返回给调用者。
2. 返回类型
按值返回
在此,return 语句中指定的值将发送回调用者。这对于执行计算或需要提供结果的函数至关重要。
int Add(int a, int b) { return a + b; // Returns the sum of a and b }
无返回值 (void)
对于声明为 void 的函数,可以使用不带表达式的 return 语句来提前退出函数。
void GetMessage() { cout << "Hello, TutorialsPoint Learner!"; return; // Exits the function }
3. 多个 return 语句
一个函数可能包含多个 return 语句,我们通常在条件语句中看到这些语句。
int max(int a, int b) { if (a > b) return a; else return b; }
4. 返回对象
函数可以返回对象,这对于返回封装在类或结构体中的多个值很有用。
struct point { int x, y; }; point getOrigin() { return {0, 0}; }
5. 提前退出
return 语句可以用来提前退出函数,这对于错误处理或特殊条件很有用。
int divideInteger(int a, int b) { if (b == 0) { cer << "Error: Division by zero!" << endl; return -1; // Shows an error } return a / b; }
C++ 中的返回类型和值处理
在 C++ 中,函数的返回类型决定了函数将返回给调用者的值类型(如果有)。正确处理返回类型和值对于确保函数按预期运行并与程序的其他部分平滑集成非常重要。
1. 原生数据类型
原生数据类型是 C++ 提供的基本内置类型。常见的示例包括 int、float、double、char 等。
示例
#include <iostream> using namespace std; // created a function which returns an integer int getSquare(int num) { return num * num; } int main() { int value = 5; int result = getSquare(value); // Calling the function and storing its result cout << "The square of " << value << " is " << result << endl; return 0; }
输出
The square of 5 is 25
2. 用户定义类型
用户定义类型包括结构体和类。这些类型允许您定义复杂的数据结构并根据您的特定需求对其进行自定义。
结构体示例
#include <iostream> using namespace std; struct Point { int x; int y; }; Point createPoint(int x, int y) { Point p; p.x = x; p.y = y; return p; // will returns a Point object } int main() { Point p = createPoint(10, 20); cout << "Point coordinates: (" << p.x << ", " << p.y << ")" << endl; return 0; }
输出
Point coordinates: (10, 20)
类示例
#include <iostream> using namespace std; class rectangle { public: rectangle(int w, int h) : width(w), height(h) {} int getArea() const { return width * height; } private: int width; int height; }; rectangle createRectangle(int width, int height) { return rectangle(width, height); // Returns a Rectangle object } int main() { rectangle rect = createRectangle(10, 5); cout << "Area of given Rectangle is: " << rect.getArea() << endl; return 0; }
输出
Area of given Rectangle is: 50
3. 引用和指针
引用和指针用于引用变量或对象而无需进行任何复制。这对于效率和在需要时轻松修改原始数据很有用。
按引用返回
#include <iostream> using namespace std; int globalValue = 100; int& getGlobalValue() { return globalValue; // Returns a reference to the global variable } int main() { int& ref = getGlobalValue(); ref = 200; // Modifies the global variable cout << "Global Value: " << globalValue << endl; return 0; }
输出
Global Value: 200
按指针返回
#include <iostream> using namespace std; int* createArray(int size) { int* array = new int[size]; // Allocating memory dynamically for (int i = 0; i < size; ++i) { array[i] = i * 10; } return array; // Returning a pointer to the allocated array } int main() { int* myArray = createArray(5); for (int i = 0; i < 5; ++i) { cout << myArray[i] << " "; } delete[] myArray; // Free dynamically allocated memory return 0; }
输出
0 10 20 30 40
广告