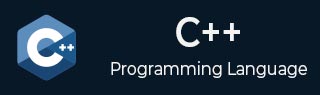
- C++ 基础
- C++ 首页
- C++ 概述
- C++ 环境设置
- C++ 基本语法
- C++ 注释
- C++ Hello World
- C++ 省略命名空间
- C++ 常量/字面量
- C++ 关键字
- C++ 标识符
- C++ 数据类型
- C++ 数值数据类型
- C++ 字符数据类型
- C++ 布尔数据类型
- C++ 变量类型
- C++ 变量作用域
- C++ 多个变量
- C++ 基本输入/输出
- C++ 修饰符类型
- C++ 存储类
- C++ 运算符
- C++ 数字
- C++ 枚举
- C++ 引用
- C++ 日期和时间
- C++ 控制语句
- C++ 决策
- C++ if 语句
- C++ if else 语句
- C++ 嵌套 if 语句
- C++ switch 语句
- C++ 嵌套 switch 语句
- C++ 循环类型
- C++ while 循环
- C++ for 循环
- C++ do while 循环
- C++ foreach 循环
- C++ 嵌套循环
- C++ break 语句
- C++ continue 语句
- C++ goto 语句
- C++ 构造函数
- C++ 构造函数和析构函数
- C++ 复制构造函数
C++ 文件读取
C++ 中的文件 I/O(输入/输出)是读取和写入文件的过程。C++ 通过标准库和 <fstream> 头文件提供了用于处理文件的功能。 文件 I/O 允许程序持久化数据,与外部资源交互,以及存储/检索超出程序执行范围的信息。
文件流类型
有三种主要类型的文件流,每种与不同的操作相关联:
- ifstream(输入文件流) - 用于从文件读取数据。
- ofstream(输出文件流) - 用于将数据写入文件。
- fstream(文件流) - 用于对文件进行输入和输出操作。它结合了 ifstream 和 ofstream 的功能。
文件 I/O 的基本步骤
以下是文件 I/O 的基本步骤:
打开文件
在读取或写入文件之前,必须使用其中一个文件流类打开它。如果文件成功打开,程序将继续进行 I/O 操作。
执行文件操作
可以使用适当的方法读取或写入文件。
关闭文件
文件操作完成后,应关闭文件以确保所有数据都被刷新并且文件被正确释放。
使用 ifstream 读取(流提取运算符)
使用流提取运算符(>>)是从文件中读取数据的最简单方法。此运算符从文件读取格式化数据,类似于从标准输入读取数据的方式。
示例
以下是使用 ifstream 读取的示例:
#include <fstream> #include <iostream> #include <string> int main() { std::ifstream inputFile("example.txt"); // Open the file for reading if (!inputFile) { std::cerr << "Error opening file!" << std::endl; return 1; } std::string word; while (inputFile >> word) { // Reads until a whitespace is encountered std::cout << word << std::endl; // Print each word from the file } inputFile.close(); // Close the file when done return 0; }
这里,>> 运算符逐个读取单词,在空白字符(空格、换行符等)处停止。
但它不处理读取文本行(因为它在空白字符处停止),并且对于读取复杂的数据格式(如包含空格的行)可能很棘手。
使用 getline() 读取行
如果要读取整行或文本,包括步骤,可以使用 getline() 函数。此函数将从文件中读取字符,直到遇到换行符('\n')。
示例
以下是使用 getline() 读取行的示例:
#include <fstream> #include <iostream> #include <string> int main() { std::ifstream inputFile("example.txt"); // Open the file for reading if (!inputFile) { std::cerr << "Error opening file!" << std::endl; return 1; } std::string line; while (std::getline(inputFile, line)) { // Read a full line of text std::cout << line << std::endl; // Output the line to the console } inputFile.close(); // Close the file when done return 0; }
读取二进制文件(使用 read())
上面讨论的方法适用于文本文件,对于二进制文件,可以使用 read() 函数从文件中读取原始二进制数据。
示例
以下是读取二进制文件(使用 read())的示例:
#include <iostream> #include <fstream> int main() { std::ifstream file("example.bin", std::ios::binary); // Check if the file was successfully opened if (!file) { std::cerr << "Error opening file!" << std::endl; return 1; } // Get the length of the file file.seekg(0, std::ios::end); std::streamsize size = file.tellg(); file.seekg(0, std::ios::beg); // Read the entire file content into a buffer char* buffer = new char[size]; file.read(buffer, size); // Print raw data (optional, for demonstration) for (std::streamsize i = 0; i < size; ++i) { std::cout << std::hex << (0xFF & buffer[i]) << " "; // Print byte in hex } delete[] buffer; file.close(); return 0; }
处理文件 I/O 中的错误
处理错误对于确保程序在文件不可用、无法打开或在读取/写入过程中发生意外情况时能够正确运行非常重要。
使用 fail()
、eof()
和 good()
检查错误。
C++ 提供了 ifstream/ofstream 对象的几个成员函数来检查各种条件。
- fail():检查 I/O 期间是否发生不可恢复的错误。
- eof():检查是否到达文件末尾 (EOF)。
- good():如果未发生错误,则返回 true。
处理文件末尾 (EOF)
从文件读取时,务必处理到达文件末尾的情况。标准方法是使用 eof() 或 std::getline() 运算符,它们会在到达文件末尾时自动停止。
优雅地处理 I/O 错误
在文件操作期间,应检查 fail() 或 bad() 状态以检测意外文件末尾或数据损坏等错误。