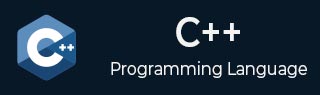
- C++ 基础
- C++ 首页
- C++ 概述
- C++ 环境设置
- C++ 基本语法
- C++ 注释
- C++ Hello World
- C++ 省略命名空间
- C++ 常量/字面量
- C++ 关键字
- C++ 标识符
- C++ 数据类型
- C++ 数值数据类型
- C++ 字符数据类型
- C++ 布尔数据类型
- C++ 变量类型
- C++ 变量作用域
- C++ 多个变量
- C++ 基本输入/输出
- C++ 修饰符类型
- C++ 存储类
- C++ 运算符
- C++ 数字
- C++ 枚举
- C++ 引用
- C++ 日期和时间
- C++ 控制语句
- C++ 决策
- C++ if 语句
- C++ if else 语句
- C++ 嵌套 if 语句
- C++ switch 语句
- C++ 嵌套 switch 语句
- C++ 循环类型
- C++ while 循环
- C++ for 循环
- C++ do while 循环
- C++ foreach 循环
- C++ 嵌套循环
- C++ break 语句
- C++ continue 语句
- C++ goto 语句
- C++ 构造函数
- C++ 构造函数和析构函数
- C++ 复制构造函数
C++ 文件和流
到目前为止,我们一直在使用iostream标准库,它提供cin和cout方法分别用于从标准输入读取和写入标准输出。
本教程将教你如何从文件读取和写入文件。这需要另一个名为fstream的标准C++库,它定义了三种新的数据类型:
序号 | 数据类型和描述 |
---|---|
1 | ofstream 此数据类型表示输出文件流,用于创建文件并将信息写入文件。 |
2 | ifstream 此数据类型表示输入文件流,用于从文件读取信息。 |
3 |
此数据类型通常表示文件流,并且具有ofstream和ifstream的功能,这意味着它可以创建文件,将信息写入文件以及从文件读取信息。 |
要在C++中执行文件处理,必须在C++源文件中包含头文件<iostream>和<fstream>。
打开文件
在读取或写入文件之前必须先打开文件。可以使用ofstream或fstream对象打开要写入的文件。而ifstream对象仅用于打开要读取的文件。
以下是open()函数的标准语法,它是fstream、ifstream和ofstream对象的成员。
void open(const char *filename, ios::openmode mode);
这里,第一个参数指定要打开的文件的名称和位置,open()成员函数的第二个参数定义应打开文件的模式。
序号 | 模式标志和描述 |
---|---|
1 | ios::app 追加模式。所有写入该文件的内容都将追加到末尾。 |
2 | ios::ate 打开一个输出文件并将读/写控制移动到文件的末尾。 |
3 | ios::in 打开一个用于读取的文件。 |
4 | ios::out 打开一个用于写入的文件。 |
5 | ios::trunc 如果文件已存在,则在打开文件之前将截断其内容。 |
您可以通过将它们一起OR来组合两个或多个这些值。例如,如果您想以写入模式打开文件,并且如果文件已存在则要截断它,则语法如下:
ofstream outfile; outfile.open("file.dat", ios::out | ios::trunc );
类似地,您可以按如下方式打开一个用于读写目的的文件:
fstream afile; afile.open("file.dat", ios::out | ios::in );
关闭文件
当C++程序终止时,它会自动刷新所有流,释放所有已分配的内存并关闭所有打开的文件。但是,程序员在程序终止前关闭所有打开的文件始终是一个好习惯。
以下是close()函数的标准语法,它是fstream、ifstream和ofstream对象的成员。
void close();
写入文件
在进行C++编程时,您可以使用流插入运算符(<<)将信息从程序写入文件,就像使用该运算符将信息输出到屏幕一样。唯一的区别是您使用ofstream或fstream对象而不是cout对象。
从文件读取
您可以使用流提取运算符(>>)将信息从文件读取到程序中,就像使用该运算符从键盘输入信息一样。唯一的区别是您使用ifstream或fstream对象而不是cin对象。
读写示例
以下是C++程序,它以读写模式打开文件。在将用户输入的信息写入名为afile.dat的文件后,程序从文件中读取信息并将其输出到屏幕上:
#include <fstream> #include <iostream> using namespace std; int main () { char data[100]; // open a file in write mode. ofstream outfile; outfile.open("afile.dat"); cout << "Writing to the file" << endl; cout << "Enter your name: "; cin.getline(data, 100); // write inputted data into the file. outfile << data << endl; cout << "Enter your age: "; cin >> data; cin.ignore(); // again write inputted data into the file. outfile << data << endl; // close the opened file. outfile.close(); // open a file in read mode. ifstream infile; infile.open("afile.dat"); cout << "Reading from the file" << endl; infile >> data; // write the data at the screen. cout << data << endl; // again read the data from the file and display it. infile >> data; cout << data << endl; // close the opened file. infile.close(); return 0; }
编译并执行上述代码时,它会产生以下示例输入和输出:
$./a.out Writing to the file Enter your name: Zara Enter your age: 9 Reading from the file Zara 9
上面的示例使用了cin对象的附加函数,例如getline()函数从外部读取行,以及ignore()函数忽略先前读取语句留下的额外字符。
文件位置指针
istream和ostream都提供用于重新定位文件位置指针的成员函数。这些成员函数对于istream是seekg(“seek get”),对于ostream是seekp(“seek put”)。
seekg和seekp的参数通常是长整数。可以指定第二个参数来指示搜索方向。搜索方向可以是ios::beg(默认值),用于相对于流的开头定位;ios::cur,用于相对于流中的当前位置定位;或者ios::end,用于相对于流的末尾定位。
文件位置指针是一个整数值,它指定文件中距文件起始位置的字节数。
// position to the nth byte of fileObject (assumes ios::beg) fileObject.seekg( n ); // position n bytes forward in fileObject fileObject.seekg( n, ios::cur ); // position n bytes back from end of fileObject fileObject.seekg( n, ios::end ); // position at end of fileObject fileObject.seekg( 0, ios::end );