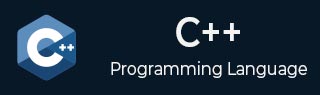
- C++ 基础
- C++ 首页
- C++ 概述
- C++ 环境设置
- C++ 基本语法
- C++ 注释
- C++ Hello World
- C++ 省略命名空间
- C++ 常量/字面量
- C++ 关键字
- C++ 标识符
- C++ 数据类型
- C++ 数值数据类型
- C++ 字符数据类型
- C++ 布尔数据类型
- C++ 变量类型
- C++ 变量作用域
- C++ 多个变量
- C++ 基本输入/输出
- C++ 修饰符类型
- C++ 存储类
- C++ 运算符
- C++ 数字
- C++ 枚举
- C++ 引用
- C++ 日期和时间
- C++ 控制语句
- C++ 决策
- C++ if 语句
- C++ if else 语句
- C++ 嵌套 if 语句
- C++ switch 语句
- C++ 嵌套 switch 语句
- C++ 循环类型
- C++ while 循环
- C++ for 循环
- C++ do while 循环
- C++ foreach 循环
- C++ 嵌套循环
- C++ break 语句
- C++ continue 语句
- C++ goto 语句
- C++ 构造函数
- C++ 构造函数和析构函数
- C++ 复制构造函数
C++ 字符串
C++ 提供以下两种类型的字符串表示:
- C 风格字符串。
- 标准 C++ 中引入的 string 类类型。
C 风格字符串
C 风格字符串起源于 C 语言,并在 C++ 中继续得到支持。这个字符串实际上是一个字符的一维数组,以空字符 '\0' 结尾。因此,一个空终止字符串包含构成字符串的字符,后面跟着一个空字符。
以下声明和初始化创建一个包含单词“Hello”的字符串。为了在数组末尾保存空字符,包含字符串的字符数组的大小比单词“Hello”中的字符数多一个。
char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
如果您遵循数组初始化规则,则可以按如下方式编写上述语句:
char greeting[] = "Hello";
以下是 C/C++ 中上述定义的字符串的内存表示:
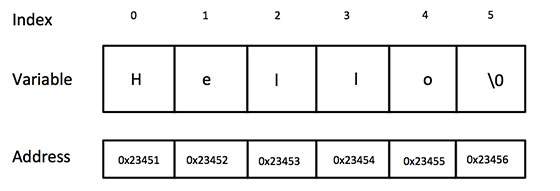
实际上,您不会在字符串常量的末尾放置空字符。C++ 编译器在初始化数组时会自动在字符串末尾放置 '\0'。让我们尝试打印上述字符串:
示例
#include <iostream> using namespace std; int main () { char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'}; cout << "Greeting message: "; cout << greeting << endl; return 0; }
编译并执行上述代码时,将产生以下结果:
Greeting message: Hello
C 风格字符串函数
C++ 支持广泛的函数来操作以 null 结尾的字符串。这些函数在<string.h> 头文件中定义。
序号 | 函数和用途 |
---|---|
1 |
将字符串 s2 复制到字符串 s1。 |
2 |
将字符串 s2 连接到字符串 s1 的末尾。 |
3 |
返回字符串 s1 的长度。 |
4 |
如果 s1 和 s2 相同,则返回 0;如果 s1<s2,则返回小于 0 的值;如果 s1>s2,则返回大于 0 的值。 |
5 |
返回指向字符串 s1 中字符 ch 的第一次出现的指针。 |
6 |
返回指向字符串 s1 中字符串 s2 的第一次出现的指针。 |
示例
以下示例使用了上述几个函数:
#include <iostream> #include <cstring> using namespace std; int main () { char str1[10] = "Hello"; char str2[10] = "World"; char str3[10]; int len ; // copy str1 into str3 strcpy( str3, str1); cout << "strcpy( str3, str1) : " << str3 << endl; // concatenates str1 and str2 strcat( str1, str2); cout << "strcat( str1, str2): " << str1 << endl; // total lenghth of str1 after concatenation len = strlen(str1); cout << "strlen(str1) : " << len << endl; return 0; }
编译并执行上述代码时,将产生如下结果:
strcpy( str3, str1) : Hello strcat( str1, str2): HelloWorld strlen(str1) : 10
C++ 中的 String 类
标准 C++ 库提供一个string 类类型,它支持上面提到的所有操作,以及更多功能。
String 类的示例
让我们检查以下示例:
#include <iostream> #include <string> using namespace std; int main () { string str1 = "Hello"; string str2 = "World"; string str3; int len ; // copy str1 into str3 str3 = str1; cout << "str3 : " << str3 << endl; // concatenates str1 and str2 str3 = str1 + str2; cout << "str1 + str2 : " << str3 << endl; // total length of str3 after concatenation len = str3.size(); cout << "str3.size() : " << len << endl; return 0; }
编译并执行上述代码时,将产生如下结果:
str3 : Hello str1 + str2 : HelloWorld str3.size() : 10
创建字符串
我们可以通过以下两种方法之一创建字符串变量:
使用字符数组创建字符串
我们可以使用C 类型数组以字符格式声明字符串。这可以通过以下语法完成:
语法
char variable_name[len_value] ;
这里,len_value 是字符数组的长度。
使用字符数组创建字符串的示例
在下面的示例中,我们声明一个字符数组,并为其赋值。
#include <iostream> using namespace std; int main() { char s[5]={'h','e','l','l','o'}; cout<<s<<endl; return 0; }
输出
hello
使用<string>创建字符串
我们可以使用 'string' 关键字声明字符串变量。这包含在<string> 头文件中。声明字符串的语法解释如下:
语法
string variable_name = [value];
这里,[value] 是可选的,可以在声明期间使用它来赋值。
示例
在下面的示例中,我们声明一个字符串变量,并为其赋值。
#include <iostream> using namespace std; int main() { string s="a merry tale"; cout<<s; return 0; }
输出
a merry tale
遍历字符串(迭代字符串)
我们可以通过两种方式迭代字符串:
使用循环语句
我们可以使用 for 循环、while 循环和 do while 循环,使用指向字符串中第一个和最后一个索引的指针来遍历字符串。
使用迭代器
使用基于范围的循环,我们可以使用迭代器迭代字符串。这是通过在运行基于范围的循环时使用 ":" 运算符实现的。
迭代字符串的示例
以下示例代码展示了使用这两种方法遍历字符串:
#include <iostream> using namespace std; int main() { string s="Hey, I am at TP."; for(int i=0;i<s.length();i++){ cout<<s[i]<<" "; } cout<<endl; for(char c:s){ cout<<c<<" "; } return 0; }
输出
H e y , I a m a t T P . H e y , I a m a t T P .
访问字符串的字符
我们可以使用迭代器和指向字符串索引的指针来访问字符串的字符。
示例
以下示例代码展示了如何访问字符串中的字符:
#include <iostream> using namespace std; int main() { string s="Hey, I am at TP."; cout<<s<<endl; for(int i=0;i<s.length();i++){ s[i]='A'; } cout<<s<<endl; for(char &c:s){ c='B'; } cout<<s<<endl; return 0; }
输出
Hey, I am at TP. AAAAAAAAAAAAAAAA BBBBBBBBBBBBBBBB
字符串函数
String 是<string>类的对象,因此它具有各种函数,用户可以利用这些函数执行各种操作。其中一些函数如下:
函数 | 描述 |
---|---|
length() | 此函数返回字符串的长度。 |
swap() | 此函数用于交换两个字符串的值。 |
size() | 用于查找字符串的大小 |
resize() | 此函数用于将字符串的长度调整到给定数量的字符。 |
find() | 用于查找参数中传递的字符串 |
push_back() | 此函数用于将字符推送到字符串的末尾 |
pop_back() | 此函数用于从字符串中弹出最后一个字符 |
clear() | 此函数用于删除字符串的所有元素。 |
find() | 此函数用于搜索字符串内的某个子字符串,并返回子字符串的第一个字符的位置。 |
replace() | 此函数用于将范围 [first, last) 中等于旧值的每个元素替换为新值。 |
substr() | 此函数用于从给定字符串创建子字符串。 |
compare() | 此函数用于比较两个字符串,并以整数的形式返回结果。 |
erase() | 此函数用于删除字符串的某个部分。 |
字符串长度
字符串的长度是字符串中存在的字符数。因此,字符串“apple”的长度为 5 个字符,字符串“hello son”的长度为 9 个字符(包括空格)。这可以使用<string> 头文件中的 length() 方法访问。
语法
语法解释如下:
int len = string_1.length();
示例
#include <iostream> #include <string> using namespace std; int main() { string x="hey boy"; cout<<x.length()<<endl; return 0; }
输出
7
字符串连接
字符串连接是将两个字符串组合在一起的一种方法。这可以通过两种方式完成:
加法运算符
加法运算符用于添加两个元素。对于字符串,加法运算符会连接两个字符串。这在以下示例中清楚地解释:
示例
#include <iostream> #include <string> using namespace std; int main() { string x = "10"; string y = "20"; cout<<x+y<<endl; return 0; }
输出
1020
这与整数加法不同。当我们取两个整数并使用加法运算符将它们相加时,我们得到这两个数字的和。
这在以下示例中清楚地解释:
示例
#include <iostream> #include <string> using namespace std; int main() { int x = 10; int y = 20; cout<<x+y<<endl; return 0; }
输出
30
使用 string append() 方法
C++ 是一种面向对象的编程语言,因此字符串实际上是一个对象,它包含可以对字符串执行某些操作的函数。我们可以使用string append() 方法将一个字符串附加到另一个字符串。
此操作的语法如下:
语法
string_1.append(string_2);
此方法的使用在以下示例中清晰地显示:
示例
#include <iostream> #include <string> using namespace std; int main() { string x="hey boy"; string y=" now"; x.append(y); cout<<x<<endl; return 0; }
输出
hey boy now
C++ 中的字符串输入
字符串可以作为程序的输入,最常见的方法是使用 cin 方法。
有三种方法可以将字符串作为输入,这些方法如下所示:
- cin
- getline()
- stringstream
使用 cin 方法
这是将字符串作为输入的最简单方法。语法如下:
语法
cin>>string_name;
示例
#include <iostream> using namespace std; int main() { string s; cout << "Enter custom string : "<<endl; //enter the string here cin>>s; cout<<"The string is : "<<s; }
使用 getline() 方法
getline() 方法可用于从输入流读取字符串。这在 <string> 头文件中定义。上述方法的语法如下:
语法
getline(cin, string_name);
示例
#include <iostream> using namespace std; int main() { string s; cout << "Enter String as input : " << endl; getline(cin, s); //enter the string here cout << "Printed string is : " << s << endl; return 0; }
使用 stringstream
stringstream 类用于一次性接收多个字符串作为输入。上述方法的语法如下:
语法
Stringstream object_name(string_name);
示例
#include <iostream> #include <sstream> #include <string> using namespace std; int main() { string s = "Hey, I am at TP"; stringstream object(s); string newstr; // >> operator will read from the stringstream object while (object >> newstr) { cout << newstr << " "; } return 0; }
输出
Hey, I am at TP