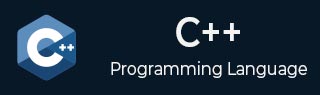
- C++基础
- C++主页
- C++概述
- C++环境设置
- C++基本语法
- C++注释
- C++ Hello World
- C++省略命名空间
- C++常量/字面量
- C++关键字
- C++标识符
- C++数据类型
- C++数值数据类型
- C++字符数据类型
- C++布尔数据类型
- C++变量类型
- C++变量作用域
- C++多个变量
- C++基本输入/输出
- C++修饰符类型
- C++存储类
- C++运算符
- C++数字
- C++枚举
- C++引用
- C++日期和时间
- C++控制语句
- C++决策制定
- C++ if 语句
- C++ if else 语句
- C++嵌套 if 语句
- C++ switch 语句
- C++嵌套 switch 语句
- C++循环类型
- C++ while 循环
- C++ for 循环
- C++ do while 循环
- C++ foreach 循环
- C++嵌套循环
- C++ break 语句
- C++ continue 语句
- C++ goto 语句
- C++构造函数
- C++构造函数和析构函数
- C++复制构造函数
C++数值数据类型
C++中的数值数据类型用于处理数值数据,例如整数(有符号和无符号)、浮点值和精度值。
数值数据类型主要包含三种基本类型的数值数据,如下所示:
- int
- int
- short int
- long int
- long long int
- unsigned int
- unsigned short int
- unsigned long int
- unsigned long long int
- float
- double
- long double
在本文中,我们将详细介绍所有数值数据类型及其子类型,并提供示例。
int 数据类型
**int** 数据类型是 integer(整数)的缩写,它接受从 -231 到 (231-1) 的数值。它在内存中占用 4 字节(即 32 位)。
语法
int variable_name;
它进一步分为各种派生子类型,如下所示:
(a) short int
**short int** 用于较小的数字,因为它在内存中只占用 2 字节(即 16 位)。其值范围为 -215 到 (215-1)。
语法
short int varianle_name;
(b) long int
**long int** 用于较大的数字,内存空间为 4 字节(即 32 位),根据编译器不同,最多可达 8 字节(即 64 位)。
语法
long int variable_name;
(c) long long int
**long long int** 用于更大的数字,内存空间为 8 字节(即 64 位),根据编译器不同,最多可达 16 字节(即 128 位)。其值范围为 -263 到 (263-1)。
语法
long long int variable_name;
(d) unsigned int
**unsigned int** 用于存储非负值,占用与 **int** 数据类型相同的内存空间,即 4 字节(即 32 位)。其值范围为 0 到 (232-1)。
语法
unsigned int variable_name;
(e) unsigned short int
**unsigned short int** 用于存储非负值,占用与 short int 数据类型相同的内存空间,即 2 字节(即 16 位)。其值范围为 0 到 (216-1)。
语法
unsigned short int variable_name;
(f) unsigned long int
**unsigned long int** 用于存储非负值,占用与 **long int** 数据类型相同的内存空间,范围为 4 字节(即 32 位)到 8 字节(即 64 位)。
语法
unsigned long int variable_name;
(g) unsigned long long int
**unsigned long long int** 用于存储非负值,占用与 **long long int** 数据类型相同的内存空间,范围为 8 字节(即 64 位)到 16 字节(128 位)。其值范围为 0 到 (264-1)。
语法
unsigned long long int variable_name;
int 数据类型示例
以下代码显示了所有派生 int 数据类型的尺寸和用法:
#include <iostream> using namespace std; int main() { int a=16; short int b=3; long int c= -32; long long int d= 555; unsigned short int e=22; unsigned int f=33; unsigned long int g=888; unsigned long long int h=444444; cout << "sizeof int datatype is: " << sizeof(a) <<" and the number is: "<<a<< endl; cout << "sizeof short int datatype is: " << sizeof(unsigned int) <<" and the number is: "<<b<< endl; cout << "sizeof long int datatype is: " << sizeof(short int) <<" and the number is: "<<c<< endl; cout << "sizeof long long int datatype is: " << sizeof(unsigned short int) <<" and the number is: "<<d<< endl; cout << "sizeof unsigned short int datatype is: " << sizeof(long int) <<" and the number is: "<<e<< endl; cout << "sizeof unsigned int datatype is: " << sizeof(unsigned long int) <<" and the number is: "<<f<< endl; cout << "sizeof unsigned long int datatype is: " << sizeof(long long int) <<" and the number is: "<<g<< endl; cout << "sizeof unsigned long long int datatype is: " << sizeof(unsigned long long int) <<" and the number is: "<<h<< endl; return 0; }
输出
sizeof int datatype is: 4 and the number is: 16 sizeof short int datatype is: 4 and the number is: 3 sizeof long int datatype is: 2 and the number is: -32 sizeof long long int datatype is: 2 and the number is: 555 sizeof unsigned short int datatype is: 8 and the number is: 22 sizeof unsigned int datatype is: 8 and the number is: 33 sizeof unsigned long int datatype is: 8 and the number is: 888 sizeof unsigned long long int datatype is: 8 and the number is: 444444
float 数据类型
**float** 数据类型用于浮点元素,即带有小数部分的数字。此数据类型占用 4 字节(即 32 位)的内存。
语法
float elem_name;
float 数据类型示例
以下代码显示了所有派生 int 数据类型的尺寸和用法:
#include <bits/stdc++.h> using namespace std; int main() { float k=1.120123; cout << "sizeof float datatype is: "<<sizeof(k)<<" and the element is: "<<k<<endl; return 0; }
输出
sizeof float datatype is: 4 and the element is: 1.12012
double 数据类型
**double** 数据类型用于存储与 **float** 相比具有双精度浮点元素。此数据类型占用 8 字节(即 64 位)的内存。
语法
double elem_name;
double 数据类型还有进一步的分类,它占用更多内存并存储更精确的元素。
long double
从 double 派生的另一种数据类型是 long double,它占用 16 字节(即 128 位)的内存空间。
语法
double elem_name;
示例
以下代码显示了所有派生 int 数据类型的尺寸和用法:
#include <bits/stdc++.h> using namespace std; int main() { double m=1.34000123; long double n=1.21312312421; cout << "sizeof double datatype is: "<<sizeof(m)<<" and the element is: "<<m<<endl; cout << "sizeof long double datatype is: "<<sizeof(n)<<" and the element is: "<<n<<endl; return 0; }
输出
sizeof double datatype is: 8 and the element is: 1.34 sizeof long double datatype is: 16 and the element is: 1.21312
数值数据类型的显式转换
在 C++ 中,显式类型转换不会自动进行,需要手动将目标数据类型放在括号 () 中进行转换。它告诉编译器将变量或表达式的值转换为特定(目标)数据类型。
示例
以下程序显示了数值数据类型从一种类型到另一种类型的显式转换:
#include <bits/stdc++.h> using namespace std; int main() { double a=6.551555; cout<<"value of a (int) is "<<(int)a<<endl; cout<<"value of a (float) is "<<(float)a<<endl; cout<<"value of a (double) is "<<a<<endl; cout<<"Value of a (long double) is "<<(long double)a; return 0; }
输出
value of a (int) is 6 value of a (float) is 6.55156 value of a (double) is 6.55155 Value of a (long double) is 6.55155