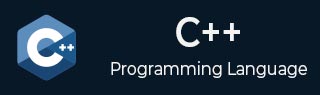
- C++ 基础
- C++ 首页
- C++ 概述
- C++ 环境设置
- C++ 基本语法
- C++ 注释
- C++ Hello World
- C++ 省略命名空间
- C++ 常量/字面量
- C++ 关键字
- C++ 标识符
- C++ 数据类型
- C++ 数值数据类型
- C++ 字符数据类型
- C++ 布尔数据类型
- C++ 变量类型
- C++ 变量作用域
- C++ 多个变量
- C++ 基本输入/输出
- C++ 修饰符类型
- C++ 存储类
- C++ 运算符
- C++ 数字
- C++ 枚举
- C++ 引用
- C++ 日期和时间
- C++ 控制语句
- C++ 决策
- C++ if 语句
- C++ if else 语句
- C++ 嵌套 if 语句
- C++ switch 语句
- C++ 嵌套 switch 语句
- C++ 循环类型
- C++ while 循环
- C++ for 循环
- C++ do while 循环
- C++ foreach 循环
- C++ 嵌套循环
- C++ break 语句
- C++ continue 语句
- C++ goto 语句
- C++ 构造函数
- C++ 构造函数和析构函数
- C++ 复制构造函数
C++ 自增和自减运算符
自增运算符 ++ 将 1 加到其操作数,自减运算符 -- 将 1 减去其操作数。因此:
x = x+1; is the same as x++;
同样:
x = x-1; is the same as x--;
自增和自减运算符都可以位于操作数之前(前缀)或之后(后缀)。例如:
x = x+1; can be written as ++x; // prefix form
或者:
x++; // postfix form
当自增或自减用作表达式的一部分时,前缀和后缀形式之间存在重要区别。如果使用前缀形式,则会在表达式其余部分之前进行自增或自减;如果使用后缀形式,则会在计算完整个表达式后进行自增或自减。
示例
以下示例说明了这种区别:
#include <iostream> using namespace std; main() { int a = 21; int c ; // Value of a will not be increased before assignment. c = a++; cout << "Line 1 - Value of a++ is :" << c << endl ; // After expression value of a is increased cout << "Line 2 - Value of a is :" << a << endl ; // Value of a will be increased before assignment. c = ++a; cout << "Line 3 - Value of ++a is :" << c << endl ; return 0; }
编译并执行上述代码后,将产生以下结果:
Line 1 - Value of a++ is :21 Line 2 - Value of a is :22 Line 3 - Value of ++a is :23
cpp_operators.htm
广告