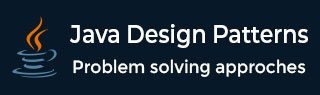
- 设计模式教程
- 设计模式 - 首页
- 设计模式 - 概述
- 设计模式 - 工厂模式
- 抽象工厂模式
- 设计模式 - 单例模式
- 设计模式 - 建造者模式
- 设计模式 - 原型模式
- 设计模式 - 适配器模式
- 设计模式 - 桥接模式
- 设计模式 - 过滤器模式
- 设计模式 - 组合模式
- 设计模式 - 装饰器模式
- 设计模式 - 外观模式
- 设计模式 - 享元模式
- 设计模式 - 代理模式
- 责任链模式
- 设计模式 - 命令模式
- 设计模式 - 解释器模式
- 设计模式 - 迭代器模式
- 设计模式 - 中介者模式
- 设计模式 - 备忘录模式
- 设计模式 - 观察者模式
- 设计模式 - 状态模式
- 设计模式 - 空对象模式
- 设计模式 - 策略模式
- 设计模式 - 模板模式
- 设计模式 - 访问者模式
- 设计模式 - MVC模式
- 业务代表模式
- 组合实体模式
- 数据访问对象模式
- 前端控制器模式
- 拦截过滤器模式
- 服务定位器模式
- 传输对象模式
- 设计模式资源
- 设计模式 - 问答
- 设计模式 - 快速指南
- 设计模式 - 有用资源
- 设计模式 - 讨论
设计模式 - 桥接模式
当我们需要将抽象与其实现解耦,以便两者可以独立变化时,可以使用桥接模式。这种设计模式属于结构型模式,因为它通过在实现类和抽象类之间提供桥接结构来解耦实现类和抽象类。
此模式包含一个充当桥接的接口,它使具体类的功能独立于接口实现类。两种类型的类都可以进行结构更改,而不会相互影响。
我们通过以下示例演示桥接模式的使用,其中可以使用相同的抽象类方法但在不同的桥接实现类中绘制不同颜色的圆圈。
实现
我们有一个DrawAPI接口,它充当桥接实现者,而具体类RedCircle、GreenCircle实现了DrawAPI接口。Shape是一个抽象类,并将使用DrawAPI的对象。BridgePatternDemo,我们的演示类将使用Shape类来绘制不同颜色的圆圈。
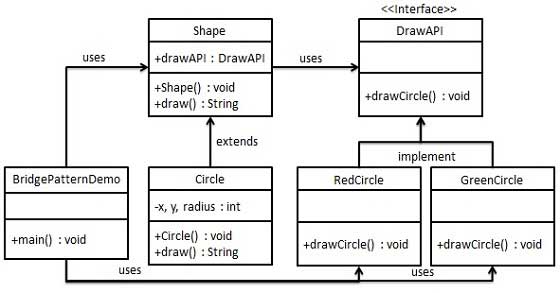
步骤 1
创建桥接实现者接口。
DrawAPI.java
public interface DrawAPI { public void drawCircle(int radius, int x, int y); }
步骤 2
创建实现DrawAPI接口的具体桥接实现类。
RedCircle.java
public class RedCircle implements DrawAPI { @Override public void drawCircle(int radius, int x, int y) { System.out.println("Drawing Circle[ color: red, radius: " + radius + ", x: " + x + ", " + y + "]"); } }
GreenCircle.java
public class GreenCircle implements DrawAPI { @Override public void drawCircle(int radius, int x, int y) { System.out.println("Drawing Circle[ color: green, radius: " + radius + ", x: " + x + ", " + y + "]"); } }
步骤 3
使用DrawAPI接口创建一个抽象类Shape。
Shape.java
public abstract class Shape { protected DrawAPI drawAPI; protected Shape(DrawAPI drawAPI){ this.drawAPI = drawAPI; } public abstract void draw(); }
步骤 4
创建实现Shape接口的具体类。
Circle.java
public class Circle extends Shape { private int x, y, radius; public Circle(int x, int y, int radius, DrawAPI drawAPI) { super(drawAPI); this.x = x; this.y = y; this.radius = radius; } public void draw() { drawAPI.drawCircle(radius,x,y); } }
步骤 5
使用Shape和DrawAPI类绘制不同颜色的圆圈。
BridgePatternDemo.java
public class BridgePatternDemo { public static void main(String[] args) { Shape redCircle = new Circle(100,100, 10, new RedCircle()); Shape greenCircle = new Circle(100,100, 10, new GreenCircle()); redCircle.draw(); greenCircle.draw(); } }
步骤 6
验证输出。
Drawing Circle[ color: red, radius: 10, x: 100, 100] Drawing Circle[ color: green, radius: 10, x: 100, 100]
广告