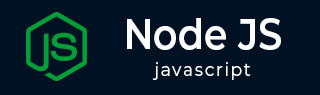
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL WHERE 子句
- Node.js - MySQL ORDER BY 子句
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL JOIN 操作
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查询数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 限制
- Node.js - MongoDB JOIN 操作
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - 命令行选项
任何 JavaScript 文件(扩展名为 .js)都可以使用它作为 node 可执行文件的命令行选项从命令提示符执行。
PS D:\nodejs> node hello.js
您可以通过运行 node 而不带任何选项来调用 Node.js REPL。
PS D:\nodejs> node >
此外,node 命令行中可以使用许多选项。要获取可用的命令行选项,请使用 --help
PS D:\nodejs> node --help
一些常用的命令行选项(有时也称为开关)如下所示:
显示版本
PS D:\nodejs> node -v v20.9.0 PS D:\nodejs> node --version v20.9.0
评估脚本
PS D:\nodejs> node --eval "console.log(123)" 123 PS D:\nodejs> node -e "console.log(123)" 123
显示帮助
PS D:\nodejs> node -h PS D:\nodejs> node –help
启动 REPL
PS D:\nodejs> node -i PS D:\nodejs> node –interactive
加载模块
PS D:\nodejs> node -r "http" PS D:\nodejs> node –require "http"
您可以将参数传递给要从命令行执行的脚本。这些参数存储在一个数组 process.argv 中。数组中的第 0 个元素是 nide 可执行文件,第一个元素是 javascript 文件,后面是传递的参数。
将以下脚本保存为 hello.js 并从命令行运行它,从命令行向其传递字符串参数。
const args = process.argv; console.log(args); const name = args[2]; console.log("Hello,", name);
在终端中输入
PS D:\nodejs> node hello.js TutorialsPoint [ 'C:\\nodejs\\node.exe', 'D:\\nodejs\\a.js', 'TutorialsPoint' ] Hello, TutorialsPoint
您还可以从 Node.js 的命令行接受输入。从 Node.js 7 版本开始,为此提供 readline 模块。createInterface() 方法有助于从可读流(例如 process.stdin 流)设置输入,在 Node.js 程序执行期间,该流是终端输入,一次一行。
将以下代码保存为 hello.js
const readline = require('readline').createInterface({ input: process.stdin, output: process.stdout, }); readline.question(`What's your name?`, name => { console.log(`Hi ${name}!`); readline.close(); });
question() 方法显示第一个参数(一个问题)并等待用户输入。按下 Enter 键后,它会调用回调函数。
从命令行运行。Node 运行时等待用户输入,然后在控制台上回显输出。
PS D:\nodejs> node a.js What's your name?TutorialsPoint Hi TutorialsPoint!
您还可以从命令行设置环境变量。在 node 可执行文件名之前为一个或多个变量赋值。
USER_ID=101 USER_NAME=admin node app.js
在脚本中,环境变量作为 process.env 对象的属性可用。
process.env.USER_ID; // "101" process.env.USER_NAME; // "admin"
广告