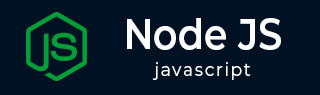
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL 条件查询
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 连接查询
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 数据限制
- Node.js - MongoDB 连接查询
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用工具模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - MongoDB 删除
Node.js 的 mongodb 驱动程序在 Collection 类中包含两种方法。deleteOne() 方法删除一个文档,而 deleteMany() 方法用于一次删除多个文档。这两种方法都需要一个 filter 参数。
collection.deleteOne(filter);
请注意,如果有多个文档满足给定的过滤器条件,则只删除第一个文档。
deleteOne()
在下面的例子中,deleteOne() 方法从 products 集合中删除一个 name 字段匹配 TV 的文档。
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://127.0.0.1:27017/"; const client = new MongoClient(uri); try { await client.connect(); await deldocs(client, "mydb", "products"); } finally { await client.close(); } } main().catch(console.error); async function deldocs(client, dbname, colname){ var myqry = { Name: "TV" }; const result = await client.db(dbname).collection(colname).deleteOne(myqry); console.log("Document Deleted"); }
执行上述代码后,使用 MongoCompass(或 MongoShell)验证预期的文档是否已删除。
deleteMany()
deleteMany() 方法也使用 filter 参数。但是,它会导致所有满足指定条件的文档都被删除。
在上面的代码中,将 deldocs() 函数更改如下。这将导致所有 price>10000 的文档被删除。
async function deldocs(client, dbname, colname){ var myqry = {"price":{$gt:10000}}; const result = await client.db(dbname).collection(colname).deleteMany(myqry); console.log("Documents Deleted"); }
删除集合
您可以使用 drop() 方法从数据库中删除集合。
示例
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://127.0.0.1:27017/"; const client = new MongoClient(uri); try { await client.connect(); await dropcol(client, "mydb", "products"); } finally { await client.close(); } } main().catch(console.error); async function dropcol(client, dbname, colname){ const result = await client.db(dbname).collection(colname).drop(); console.log("Collection dropped "); }
db 对象中还有一个可用的 **dropCollection()** 方法。
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://127.0.0.1:27017/"; const client = new MongoClient(uri); try { await client.connect(); await dropcol(client, "mydb", "orders"); } finally { await client.close(); } } main().catch(console.error); async function dropcol(client, dbname, colname){ const result = await client.db(dbname).dropCollection(colname); console.log("Collection dropped"); }
广告