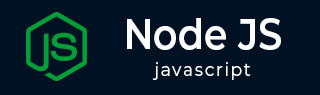
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL 条件查询
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 连接查询
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 数据限制
- Node.js - MongoDB 连接查询
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - 文件系统
Node.js API 是一种服务器端编程技术。因此,Node.js 应用程序可能需要与服务器的物理文件系统交互。Node.js API 包含 fs 模块,使开发人员能够对磁盘文件执行读/写操作。Node.js 中的 fs 模块提供同步和异步方法来处理文件。
要使用文件系统函数,您需要使用以下语法导入 fs 模块:
var fs = require("fs")
同步与异步
fs 模块中的每个方法都有同步和异步版本。异步方法将最后一个参数作为完成函数回调,回调函数的第一个参数作为错误。
例如,在文件中写入数据的同步方法是:
fs.writeFileSync(file, data[, options])
另一方面,它的异步版本具有以下语法:
fs.writeFile(file, data[, options], callback)
与同步方法相比,异步方法是非阻塞的。
对于本章中的示例代码,我们将使用一个名为 input.txt 的文本文件,其内容如下:
Tutorials Point is giving self learning content to teach the world in simple and easy way!!!!!
写入文件
以下程序演示了如何使用同步和异步方法在文件中写入数据。
const fs = require('fs'); var text = `Tutorials Point is giving self learning content to teach the world in simple and easy way!!!!! `; console.log("Writing synchronously"); fs.writeFileSync("input.txt", text); console.log("Writing asynchronously"); fs.writeFile('input.txt', text, function (err) { if (err) console.log(err); else console.log('Write operation complete.'); });
输出
Writing synchronously Writing asynchronously Write operation complete.
读取文件
fs 模块中的 ReadFile() 方法异步读取文件。它具有以下语法:
fs.readFile(fileName [,options], callback)
另一方面,ReadFileSync() 方法是其同步版本,具有以下语法:
fs.readFileSync(fileName [,options])
示例
以下程序同步和异步地读取 input.txt 文件。
const fs = require('fs'); console.log("Reading synchronously"); data = fs.readFileSync("input.txt"); console.log(data.toString()); console.log("Reading asynchronously"); fs.readFile('input.txt', function (err, data) { if (err) { return console.error(err); } console.log("Asynchronous read: " + data.toString()); }); console.log('Read operation complete.');
输出
Reading synchronously Tutorials Point is giving self learning content to teach the world in simple and easy way!!!!! Reading asynchronously Read operation complete. Asynchronous read: Tutorials Point is giving self learning content to teach the world in simple and easy way!!!!!
打开文件
readFile() 和 writeFile() 方法隐式地打开和关闭要读取/写入的文件。相反,您可以显式打开文件,设置文件打开模式以指示是用于读取还是写入,然后关闭文件。
open() 方法具有以下签名:
fs.open(path, flags[, mode], callback)
参数
path − 包含路径的文件名字符串。
flags − 标志指示要打开的文件的行为。所有可能的值如下所示。
mode − 它设置文件模式(权限和粘滞位),但仅当创建文件时才设置。默认为 0666,可读和可写。
callback − 这是回调函数,它接收两个参数 (err, fd)。
flag 参数的值为:
序号 | 标志 & 描述 |
---|---|
1 |
r 打开文件以进行读取。如果文件不存在,则会发生异常。 |
2 |
r+ 打开文件以进行读写。如果文件不存在,则会发生异常。 |
3 |
rs 以同步模式打开文件以进行读取。 |
4 |
rs+ 打开文件以进行读写,要求操作系统以同步方式打开它。有关谨慎使用此方法,请参阅“rs”的说明。 |
5 |
w 打开文件以进行写入。如果文件不存在,则创建文件;如果文件存在,则截断文件。 |
6 |
wx 类似于“w”,但如果路径存在则失败。 |
7 |
w+ 打开文件以进行读写。如果文件不存在,则创建文件;如果文件存在,则截断文件。 |
8 |
wx+ 类似于“w+”,但如果路径存在则失败。 |
9 |
a 打开文件以进行追加。如果文件不存在,则创建文件。 |
10 |
ax 类似于“a”,但如果路径存在则失败。 |
11 |
a+ 打开文件以进行读取和追加。如果文件不存在,则创建文件。 |
12 |
ax+ 类似于“a+”,但如果路径存在则失败。 |
让我们打开一个名为“input.txt”的文件,以便向其中写入数据。
const fd = fs.open('input.txt', 'w', (err, fd) => { if (err) { console.log(err); return; } });
open() 方法返回对文件的引用,称为文件描述符。文件描述符是一个唯一的整数,用作执行写入和读取操作的方法的参数。
fs 模块中的 write() 方法将数据保存到 open() 方法返回的文件描述符引用的文件中。
write(fd, string[, position[, encoding]], callback)
参数
fd − 文件描述符
string − 要写入的数据
position − 从哪里开始写入 默认值:null
encoding − 字符编码字符串 默认值:'utf8'
callback − 要调用的回调函数。
以下代码片段将给定的文本写入我们上面打开的文件中。
var data = `Tutorials Point is giving self learning content to teach the world in simple and easy way!!!!! `; if (err) { console.log(err); return; }
始终建议关闭打开的文件,尤其是在以可写模式打开时。
// Close the file fs.close(fd, (err) => { if (err) { console.log(err); return; } });
让我们将所有这些代码片段组合成一个程序,以可写模式打开文件,向其中放入一些数据,然后关闭它。
示例
const fs = require('fs'); // Open a file for writing const fd = fs.open('input.txt', 'w', (err, fd) => { if (err) { console.log(err); return; } // Write some data to the file var data = `Tutorials Point is giving self learning content to teach the world in simple and easy way!!!!! `; fs.write(fd, data, (err) => { if (err) { console.log(err); return; } // Close the file fs.close(fd, (err) => { if (err) { console.log(err); return; } console.log('The file was written successfully!'); }); }); });
执行后,上述程序会在当前目录中创建 input.txt。
要读回文件,必须以读取模式打开它。fs 模块中的 read() 方法使用文件描述符并在缓冲区对象中检索其中的数据。
read(fd, buffer[, options], callback)
参数
fd − 文件描述符
buffer − 数据将写入到的缓冲区。
options − 偏移量、长度和位置
callback − 要调用的函数。
要读回 input.txt 中的数据,请按如下方式使用 read() 方法:
示例
var fs = require('fs'); fs.open('test.txt', 'r', function (err, fd) { if (err) { return console.error(err); } var buffr = Buffer.alloc(1024); fs.read(fd, buffr, function (err) { if (err) throw err; else console.log(buffr.toString()); }); // Close the opened file. fs.close(fd, function (err) { if (err) throw err; }); });
运行上述程序。input.txt 的内容将被检索并显示在控制台上。
Promise API
在 Node.js 应用程序中,如果需要异步执行许多不同的活动,则可能会有大量的嵌套回调。但是,代码随后可能会变得杂乱无章,通常称为回调地狱。为了克服这个问题,JavaScript 引入了 Promise 的概念。
Promise 本质上是对执行异步任务的回调的改进。它表示将完成或拒绝的活动。如果 Promise 已完成,则将其解析;否则,将其拒绝。与典型的回调不同,Promise 可以链接。
在 Node.js 10 版之后的版本中,fs 模块包含实现 Promise 概念的文件管理方法。(回调和同步方法也存在)。
新的异步函数使用 async/await 语法。该函数以 async 关键字为前缀,并且始终返回一个 Promise。await 关键字使 JavaScript 等待该 Promise 完成并返回其结果。
fs 模块中 writeFile() 方法的 Promise API 版本如下:
fsPromises.writeFile(file, data[, options])
参数
file − 文件名或 FileHandle
data − 字符串或缓冲区。该方法返回一个 Promise 对象。它异步地将数据写入文件,如果文件已存在则替换该文件。data 可以是字符串或缓冲区。
以下程序使用 Promise 化的 writeFile() 方法。
示例
const fs = require("fs"); var data = `Tutorials Point is giving self learning content to teach the world in simple and easy way!!!!! `; async function write_file() { await fs.promises.writeFile("input.txt", data); console.log("Data written successfully"); } write_file();
另一方面,Promise API 中的 readFile() 方法具有以下语法:
fsPromises.readFile(path[, options])#
参数
path − 文件名或 FileHandle
options 包括 encoding、flag、signal
该方法返回 − 一个 Promise。它异步地读取文件的全部内容。
示例
const fs = require("fs"); async function read_file() { const secret = await fs.promises.readFile("input.txt"); console.log(secret.toString()); } read_file();
要检索文件 (input.txt) 的内容,请保存上述代码并从命令行运行。
获取文件信息
语法
以下是获取有关文件信息的方法的语法:
fs.stat(path, callback)
参数
以下是使用的参数说明:
path − 包含路径的文件名字符串。
callback − 这是回调函数,它接收两个参数 (err, stats),其中stats 是 fs.Stats 类型的对象,如下面的示例中所示。
除了下面示例中打印的重要属性外,fs.Stats 类中还提供了一些有用的方法来检查文件类型。这些方法在下面的表中给出。
序号 | 方法 & 描述 |
---|---|
1 |
stats.isFile() 如果文件类型是简单文件,则返回 true。 |
2 |
stats.isDirectory() 如果文件类型是目录,则返回 true。 |
3 |
stats.isBlockDevice() 如果文件类型是块设备,则返回 true。 |
4 |
stats.isCharacterDevice() 如果文件类型是字符设备,则返回 true。 |
5 |
stats.isSymbolicLink() 如果文件类型是符号链接,则返回 true。 |
6 |
stats.isFIFO() 如果文件类型是 FIFO,则返回 true。 |
7 |
stats.isSocket() 如果文件类型是套接字,则返回 true。 |
示例
让我们创建一个名为main.js的 js 文件,其中包含以下代码:
var fs = require("fs"); console.log("Going to get file info!"); fs.stat('input.txt', function (err, stats) { if (err) { return console.error(err); } console.log(stats); console.log("Got file info successfully!"); // Check file type console.log("isFile ? " + stats.isFile()); console.log("isDirectory ? " + stats.isDirectory()); });
现在运行 main.js 以查看结果:
$ node main.js
验证输出。
Going to get file info! { dev: 1792, mode: 33188, nlink: 1, uid: 48, gid: 48, rdev: 0, blksize: 4096, ino: 4318127, size: 97, blocks: 8, atime: Sun Mar 22 2015 13:40:00 GMT-0500 (CDT), mtime: Sun Mar 22 2015 13:40:57 GMT-0500 (CDT), ctime: Sun Mar 22 2015 13:40:57 GMT-0500 (CDT) } Got file info successfully! isFile ? true isDirectory ? false
关闭文件
语法
以下是关闭打开文件的语法:
fs.close(fd, callback)
参数
以下是使用的参数说明:
fd − 这是 fs.open() 方法返回的文件描述符。
callback − 这是回调函数,除了可能的异常之外,没有其他参数传递给完成回调。
示例
让我们创建一个名为main.js的 js 文件,其中包含以下代码:
var fs = require("fs"); var buf = new Buffer(1024); console.log("Going to open an existing file"); fs.open('input.txt', 'r+', function(err, fd) { if (err) { return console.error(err); } console.log("File opened successfully!"); console.log("Going to read the file"); fs.read(fd, buf, 0, buf.length, 0, function(err, bytes) { if (err) { console.log(err); } // Print only read bytes to avoid junk. if(bytes > 0) { console.log(buf.slice(0, bytes).toString()); } // Close the opened file. fs.close(fd, function(err) { if (err) { console.log(err); } console.log("File closed successfully."); }); }); });
现在运行 main.js 以查看结果:
$ node main.js
验证输出。
Going to open an existing file File opened successfully! Going to read the file Tutorials Point is giving self learning content to teach the world in simple and easy way!!!!! File closed successfully.
截断文件
语法
以下是截断打开文件的方法的语法:
fs.ftruncate(fd, len, callback)
参数
以下是使用的参数说明:
fd − 这是 fs.open() 返回的文件描述符。
len − 这是文件在截断后的长度。
callback − 这是回调函数,除了可能的异常之外,没有其他参数传递给完成回调。
示例
让我们创建一个名为main.js的 js 文件,其中包含以下代码:
var fs = require("fs"); var buf = new Buffer(1024); console.log("Going to open an existing file"); fs.open('input.txt', 'r+', function(err, fd) { if (err) { return console.error(err); } console.log("File opened successfully!"); console.log("Going to truncate the file after 10 bytes"); // Truncate the opened file. fs.ftruncate(fd, 10, function(err) { if (err) { console.log(err); } console.log("File truncated successfully."); console.log("Going to read the same file"); fs.read(fd, buf, 0, buf.length, 0, function(err, bytes){ if (err) { console.log(err); } // Print only read bytes to avoid junk. if(bytes > 0) { console.log(buf.slice(0, bytes).toString()); } // Close the opened file. fs.close(fd, function(err) { if (err) { console.log(err); } console.log("File closed successfully."); }); }); }); });
现在运行 main.js 以查看结果:
$ node main.js
验证输出。
Going to open an existing file File opened successfully! Going to truncate the file after 10 bytes File truncated successfully. Going to read the same file Tutorials File closed successfully.
删除文件
语法
以下是删除文件的方法的语法:
fs.unlink(path, callback)
参数
以下是使用的参数说明:
path − 包含路径的文件名。
callback − 这是回调函数,除了可能的异常之外,没有其他参数传递给完成回调。
示例
让我们创建一个名为main.js的 js 文件,其中包含以下代码:
var fs = require("fs"); console.log("Going to delete an existing file"); fs.unlink('input.txt', function(err) { if (err) { return console.error(err); } console.log("File deleted successfully!"); });
现在运行 main.js 以查看结果:
$ node main.js
验证输出。
Going to delete an existing file File deleted successfully!
创建目录
语法
以下是创建目录的方法的语法:
fs.mkdir(path[, mode], callback)
参数
以下是使用的参数说明:
path − 这是包含路径的目录名称。
mode − 这是要设置的目录权限。默认为 0777。
callback − 这是回调函数,除了可能的异常之外,没有其他参数传递给完成回调。
示例
让我们创建一个名为main.js的 js 文件,其中包含以下代码:
var fs = require("fs"); console.log("Going to create directory /tmp/test"); fs.mkdir('/tmp/test',function(err) { if (err) { return console.error(err); } console.log("Directory created successfully!"); });
现在运行 main.js 以查看结果:
$ node main.js
验证输出。
Going to create directory /tmp/test Directory created successfully!
读取目录
语法
以下是读取目录的方法语法:
fs.readdir(path, callback)
参数
以下是使用的参数说明:
path − 这是包含路径的目录名称。
callback − 这是一个回调函数,它接收两个参数 (err, files),其中 files 是一个数组,包含目录中文件的名称,不包括 '.' 和 '..'。
示例
让我们创建一个名为main.js的 js 文件,其中包含以下代码:
var fs = require("fs"); console.log("Going to read directory /tmp"); fs.readdir("/tmp/",function(err, files) { if (err) { return console.error(err); } files.forEach( function (file) { console.log( file ); }); });
现在运行 main.js 以查看结果:
$ node main.js
验证输出。
Going to read directory /tmp ccmzx99o.out ccyCSbkF.out employee.ser hsperfdata_apache test test.txt
删除目录
语法
以下是删除目录的方法语法:
fs.rmdir(path, callback)
参数
以下是使用的参数说明:
path − 这是包含路径的目录名称。
callback − 这是回调函数,除了可能的异常之外,没有其他参数传递给完成回调。
示例
让我们创建一个名为main.js的 js 文件,其中包含以下代码:
var fs = require("fs"); console.log("Going to delete directory /tmp/test"); fs.rmdir("/tmp/test",function(err) { if (err) { return console.error(err); } console.log("Going to read directory /tmp"); fs.readdir("/tmp/",function(err, files) { if (err) { return console.error(err); } files.forEach( function (file) { console.log( file ); }); }); });
现在运行 main.js 以查看结果:
$ node main.js
验证输出。
Going to read directory /tmp ccmzx99o.out ccyCSbkF.out employee.ser hsperfdata_apache test.txt