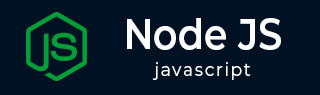
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTFul API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 从表中选择数据
- Node.js - MySQL Where 条件
- Node.js - MySQL Order By 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 联接
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 限制结果
- Node.js - MongoDB 联接
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - MongoDB排序
在 MongoDB 数据库中的集合上执行 find() 查询的结果,可以按照文档中某个特定字段的升序或降序排列。Node.js 的 MongoDB 驱动程序在 Collection 对象中定义了 sort() 方法。
要对 Node.js 中 MongoDB 查询的结果进行排序,可以使用 sort() 方法。此方法允许您按一个或多个字段的值以特定方向对返回的文档进行排序。要按字段的升序(从小到大)排序返回的文档,请使用值 1。要改为按降序(从大到小)排序,请使用 -1。
升序排序的语法如下:
result = col.find(query).sort(field:1);
降序排序的语法如下:
result = col.find(query).sort(field:-1);
升序排序
以下示例显示了 products 集合中的文档,按价格升序排列。
示例
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://127.0.0.1:27017/"; const client = new MongoClient(uri); try { await client.connect(); await sortdocs(client, "mydb", "products"); } finally { await client.close(); } } main().catch(console.error); async function sortdocs(client, dbname, colname){ var mysort = { price: 1 }; const result = await client.db(dbname).collection(colname).find({}).sort(mysort).toArray(); result.forEach(element => { console.log(element); }); }
输出
{ _id: new ObjectId('6580964f20f979d2e9a72ae9'), ProductID: 3, Name: 'Router', price: 2000 } { _id: new ObjectId('6580964f20f979d2e9a72aea'), ProductID: 4, Name: 'Scanner', price: 5000 } { _id: new ObjectId('6580964f20f979d2e9a72aeb'), ProductID: 5, Name: 'Printer', price: 9000 } { _id: new ObjectId('65809214693bd4622484dce3'), ProductID: 1, Name: 'Laptop', Price: 25000 } { _id: new ObjectId('6580964f20f979d2e9a72ae8'), ProductID: 2, Name: 'TV', price: 40000 }
降序排序
要从 products 集合中生成按名称字段降序排列的文档列表,请将 sortdocs() 函数更改为以下内容:
示例
async function sortdocs(client, dbname, colname){ var mysort = { Name: -1 }; const result = await client.db(dbname).collection(colname).find({}).sort(mysort).toArray(); result.forEach(element => { console.log(element); }); });
输出
{ _id: new ObjectId('6580964f20f979d2e9a72ae8'), ProductID: 2, Name: 'TV', price: 40000 } { _id: new ObjectId('6580964f20f979d2e9a72aea'), ProductID: 4, Name: 'Scanner', price: 5000 } { _id: new ObjectId('6580964f20f979d2e9a72ae9'), ProductID: 3, Name: 'Router', price: 2000 } { _id: new ObjectId('6580964f20f979d2e9a72aeb'), ProductID: 5, Name: 'Printer', price: 9000 } { _id: new ObjectId('65809214693bd4622484dce3'), ProductID: 1, Name: 'Laptop', Price: 25000 }
广告