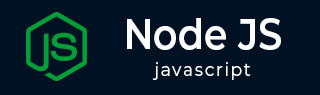
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL 条件查询
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 连接查询
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 数据限制
- Node.js - MongoDB 连接查询
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - 控制台
控制台是一个基于文本的用户界面,允许您与计算机的操作系统交互或运行代码。 使用控制台,您可以编写和执行命令或程序。 它也用于调试和故障排除。 Node.js 的内置库包含 Console 模块。它提供将消息打印到 IO 流的功能,该功能类似于 Web 浏览器提供的 JavaScript 控制台机制。
Console 模块的功能主要分为两部分:
Console 类:Console 类的常用方法有 console.log()、console.error() 和 console.warn(),用于显示 Node.js 流。
全局 console 对象:一个预定义的对象,将日志、错误和警告消息回显到标准输出流。 使用时无需调用 require('console')。
全局 console 对象的默认用法很简单。 下面的代码展示了全局 console 对象的典型用法,用于显示日志、错误和警告消息。
示例
// Welcome message console.log('Welcome to Tutorialspoint!'); // Hello world message console.log('hello world'); // printing to error stream console.error(new Error('Oops... something went wrong')); // print warning const name = 'NodeJS Tutorial'; console.warn(`Warning ${name}! Warning!`);
输出
Welcome to Tutorialspoint! hello world Error: Oops... something went wrong at Object.<anonymous> (D:\nodejs\emailapp\main.js:11:15) at Module._compile (node:internal/modules/cjs/loader:1241:14) at Module._extensions..js (node:internal/modules/cjs/loader:1295:10) at Module.load (node:internal/modules/cjs/loader:1091:32) at Module._load (node:internal/modules/cjs/loader:938:12) at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:83:12) at node:internal/main/run_main_module:23:47 Warning NodeJS Tutorial! Warning!
您还可以通过指定用于反映输出和错误日志的流来自定义 console 对象。声明 Console 类的对象并将流对象作为参数传递。
下面的程序将日志和错误重定向到两个磁盘文件。
const fs = require('fs'); const out = fs.createWriteStream('./stdout.log'); const err = fs.createWriteStream('./stderr.log'); const logger = new console.Console(out, err); // Welcome message logger.log('Welcome to Tutorialspoint!'); // Hello world message logger.log('hello world'); // printing to error stream logger.error(new Error('Oops... something went wrong')); // print warning const name = 'NodeJS Tutorial'; logger.warn(`Warning ${name}! Warning!`);
这将在当前目录中创建两个文件 stdout.log 和 stderr.log,其中保存了 console 对象的 log()、error() 和 warn() 方法的结果。
Console 方法
以下是 console 对象可用的方法列表。
序号 | 方法及描述 |
---|---|
1 |
console.log([data][, ...]) 带换行符打印到 stdout。此函数可以像 printf() 一样接受多个参数。 |
2 |
console.info([data][, ...]) 带换行符打印到 stdout。此函数可以像 printf() 一样接受多个参数。 |
3 |
console.error([data][, ...]) 带换行符打印到 stderr。此函数可以像 printf() 一样接受多个参数。 |
4 |
console.warn([data][, ...]) 带换行符打印到 stderr。此函数可以像 printf() 一样接受多个参数。 |
5 |
console.dir(obj[, options]) 对 obj 使用 util.inspect 并将结果字符串打印到 stdout。 |
6 |
console.time(label) 标记时间。 |
7 |
console.timeEnd(label) 结束计时器,记录输出。 |
8 |
console.trace(message[, ...]) 打印到 stderr 'Trace :', 后跟格式化的消息和到当前位置的堆栈跟踪。 |
9 |
console.assert(value[, message][, ...]) 类似于 assert.ok(),但错误消息格式化为 util.format(message...)。 |