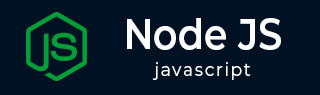
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL 条件查询
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 连接查询
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入
- Node.js - MongoDB 查询
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除
- Node.js - MongoDB 更新
- Node.js - MongoDB 限制
- Node.js - MongoDB 连接查询
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - MongoDB 插入
MongoDB是一个面向文档的数据库,可以通过名为mongodb的NPM模块与Node.js交互。文档是MongoDB数据库的核心。它是一组键值对的集合。我们也可以将其视为类似于SQL基于关系数据库的表中的一行,就像MongoDB中的集合类似于关系数据库中的表一样。NPM mongodb包提供了insertOne()和insertMany()方法,可以使用这些方法将一个或多个文档从Node.js应用程序添加到集合中。
MongoDB将数据记录存储为BSON文档。BSON是JSON文档的二进制表示。MongoDB文档由字段和值对组成,具有以下结构:
{ field1: value1, field2: value2, field3: value3, ... fieldN: valueN }
字段的值可以是任何BSON数据类型,包括其他文档、数组和文档数组。
每个文档都以一个名为“_id”的特殊键为特征,该键具有唯一值,类似于关系数据库实体表中的主键。键也称为字段。如果插入的文档省略了_id字段,则MongoDB驱动程序会自动为_id字段生成一个ObjectId。
insertOne() 方法
Collection对象具有insertOne()方法,该方法将单个文档插入到集合中。
Collection.insertOne(doc)
要插入的文档作为参数传递。它将单个文档插入到MongoDB中。如果传入的文档不包含_id字段,驱动程序将向每个缺少该字段的文档添加一个_id字段,从而修改文档。
示例
使用以下代码,我们将单个文档插入数据库中的products集合中。
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://127.0.0.1:27017"; const client = new MongoClient(uri); try { // Connect to the MongoDB cluster await client.connect(); // Make the appropriate DB calls // Create a single new document await createdoc(client, "mydatabase", "products", { "ProductID":1, "Name":"Laptop", "Price":25000 }); } finally { // Close the connection to the MongoDB cluster await client.close(); } } main().catch(console.error); async function createdoc(client, dbname, colname, doc){ const dbobj = await client.db(dbname); const col = dbobj.collection(colname); const result = await col.insertOne(doc); console.log(`New document created with the following id: ${result.insertedId}`); }
输出
创建新的列表,ID为:65809214693bd4622484dce3
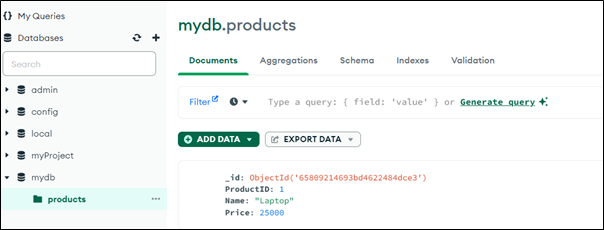
Mongo shell也可以用来查看插入的文档。
> use mydb; < switched to db mydb > products=db['products'] < mydb.products > docs=products.find() { _id: ObjectId("65809214693bd4622484dce3"), ProductID: 1, Name: 'Laptop', Price: 25000 }
insertMany() 方法
Collection对象的insertMany()方法使得可以插入多个文档。JSON文档数组用作参数。我们还在下面的示例中使用了SRV连接字符串:
示例
const {MongoClient} = require('mongodb'); async function main(){ //const uri = "mongodb://127.0.0.1:27017"; const uri = "mongodb+srv://user:[email protected]/?retryWrites=true&w=majority"; const client = new MongoClient(uri); try { // Connect to the MongoDB cluster await client.connect(); // Make the appropriate DB calls // insert documents await createdocs(client, [ {'ProductID':1, 'Name':'Laptop', 'price':25000}, {'ProductID':2, 'Name':'TV', 'price':40000}, {'ProductID':3, 'Name':'Router', 'price':2000}, {'ProductID':4, 'Name':'Scanner', 'price':5000}, {'ProductID':5, 'Name':'Printer', 'price':9000} ]); } finally { // Close the connection to the MongoDB cluster await client.close(); } } main().catch(console.error); async function createdocs(client, docs){ const result = await client.db("mydb").collection("products").insertMany(docs); console.log(`${result.insertedCount} new document(s) created with the following id(s):`); console.log(result.insertedIds); }
输出
5 new listing(s) created with the following id(s): { '0': new ObjectId('6580964f20f979d2e9a72ae7'), '1': new ObjectId('6580964f20f979d2e9a72ae8'), '2': new ObjectId('6580964f20f979d2e9a72ae9'), '3': new ObjectId('6580964f20f979d2e9a72aea'), '4': new ObjectId('6580964f20f979d2e9a72aeb') }
您可以将文档集合导出为CSV格式。
_id,ProductID,Name,Price,price 65809214693bd4622484dce3,1,Laptop,25000, 6580964f20f979d2e9a72ae8,2,TV,40000 6580964f20f979d2e9a72ae9,3,Router,2000 6580964f20f979d2e9a72aea,4,Scanner,5000 6580964f20f979d2e9a72aeb,5,Printer,9000
广告