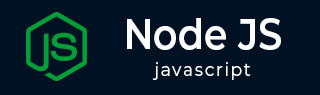
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 从表中选择数据
- Node.js - MySQL Where 条件
- Node.js - MySQL Order By 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 联接
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 限制数据
- Node.js - MongoDB 联接
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - Web 模块
什么是 Web 服务器?
Node.js 中的 http 模块支持服务器和客户端之间通过超文本传输协议 (HTTP) 传输数据。http 模块中的 createServer() 函数创建 Node.js http 服务器的实例。它监听来自其他 http 客户端在指定主机和端口上的传入请求。
Node.js 服务器是一个软件应用程序,它处理由 HTTP 客户端(如 Web 浏览器)发送的 HTTP 请求,并响应客户端的请求返回网页。Web 服务器通常提供 html 文档以及图像、样式表和脚本。
大多数 Web 服务器都支持服务器端脚本,使用脚本语言或将任务重定向到应用程序服务器,应用程序服务器从数据库中检索数据并执行复杂的逻辑,然后通过 Web 服务器将结果发送到 HTTP 客户端。
Web 应用程序架构
Web 应用程序通常分为四个层:
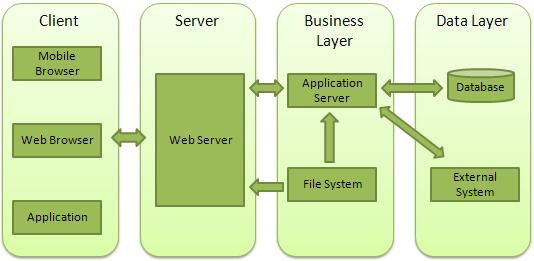
客户端 - 此层包含 Web 浏览器、移动浏览器或可以向 Web 服务器发出 HTTP 请求的应用程序。
服务器 - 此层包含 Web 服务器,它可以拦截客户端发出的请求并传递响应。
业务逻辑 - 此层包含应用程序服务器,Web 服务器利用它来执行所需的处理。此层通过数据库或某些外部程序与数据层交互。
数据 - 此层包含数据库或任何其他数据源。
使用 Node 创建 Web 服务器
Node.js 提供了一个 http 模块,可用于创建服务器的 HTTP 客户端。以下是监听 5000 端口的 HTTP 服务器的最小结构。
创建一个名为 server.js 的 js 文件:
var http = require('http'); var fs = require('fs'); var url = require('url'); // Create a server http.createServer( function (request, response) { // Parse the request containing file name var pathname = url.parse(request.url).pathname; // Print the name of the file for which request is made. console.log("Request for " + pathname + " received."); // Read the requested file content from file system fs.readFile(pathname.substr(1), function (err, data) { if (err) { console.log(err); } else { response.writeHead(200, {'Content-Type': 'text/html'}); // Write the content of the file to response body console.log(data.toString()); response.write(data.toString()); } // Send the response body response.end(); }); }).listen(5000); // Console will print the message console.log('Server running at http://127.0.0.1:5000/');
createServer() 函数开始监听 localhost 的 5000 端口上的客户端请求。Http 请求和服务器响应对象由 Nde.js 服务器内部提供。它获取 HTTP 客户端请求的 HTML 文件的 URL。createServer() 函数的回调函数读取请求的文件,并将它的内容作为服务器的响应写入。
您可以在服务器机器本身的浏览器上发送 HTTP 请求。运行上述 server.js 文件,并在浏览器窗口中输入 https://127.0.0.1:5000/index.html 作为 URL。index.html 页面的内容将被呈现。
发送对任何其他现有网页的请求,例如 https://127.0.0.1:5000/hello.html,请求的页面将被呈现。
使用 Node 创建 Web 客户端
可以使用 http 模块创建 Web 客户端。让我们检查以下示例。
创建一个名为 client.js 的 js 文件(将此文件保存在另一个文件夹中,不要保存在包含 server.js 脚本的文件夹中):
var http = require('http'); var fs = require('fs'); var path = require('path'); // Options to be used by request var options = { host: 'localhost', port: '5000', path: path.join('/',process.argv[2]) }; var body = ''; // Callback function is used to deal with response var callback = function(response) { // Continuously update stream with data response.on('data', function(data) { body += data; }); response.on('end', function() { // Data received completely. console.log(body); fs.writeFile(options.path.substr(1), body, function (err) { if (err) console.log(err); else console.log('Write operation complete.'); }); }); } // Make a request to the server var req = http.request(options, callback); req.end();
此客户端脚本发送对作为命令行参数给出的网页的 HTTP 请求。例如:
node main.js index.html
文件名称是参数列表中的 argv[2]。它用作要传递给 http.request() 方法的 options 参数中的路径参数。一旦服务器接受此请求,它的内容就会写入响应流。client.js 接收此响应并将接收到的数据作为新文件写入客户端的文件夹。
首先运行服务器代码。从另一个终端运行客户端代码。您将看到在客户端文件夹中创建的请求的 HTML 文件。