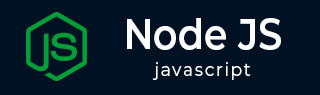
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTFul API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 从表中选择数据
- Node.js - MySQL Where 条件
- Node.js - MySQL Order By 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 联接
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 限制结果数量
- Node.js - MongoDB 联接
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - MongoDB 创建集合
MongoDB 数据库由一个或多个集合组成。集合是一组文档对象。在 MongoDB 服务器(独立服务器或 MongoDB Atlas 中的共享集群)上创建数据库后,您可以在其中创建集合。Node.js 的 mongodb 驱动程序有一个 cerateCollection() 方法,它返回一个 Collection 对象。
MongoDB 中的集合类似于关系数据库中的表。但是,它没有预定义的模式。集合中的每个文档可能包含可变数量的键值对,并且每个文档中的键不一定相同。
要创建集合,请从数据库连接获取数据库对象并调用 createCollection() 方法。
db.createCollection(name: string, options)
要创建的集合的名称作为参数传递。该方法返回一个 Promise。集合命名空间验证在服务器端执行。
const dbobj = await client.db(dbname); const collection = await dbobj.createCollection("MyCollection");
请注意,即使在插入之前没有创建集合,当您向其中插入文档时,也会隐式创建该集合。
const result = await client.db("mydatabase").collection("newcollection").insertOne({k1:v1, k2:v2});
示例
以下 Node.js 代码在名为 mydatabase 的 MongoDB 数据库中创建一个名为 MyCollection 的集合。
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://127.0.0.1:27017/"; const client = new MongoClient(uri); try { // Connect to the MongoDB cluster await client.connect(); await newcollection(client, "mydatabase"); } finally { // Close the connection to the MongoDB cluster await client.close(); } } main().catch(console.error); async function newcollection (client, dbname){ const dbobj = await client.db(dbname); const collection = await dbobj.createCollection("MyCollection"); console.log("Collection created"); console.log(collection); }
MongoDB Compass 显示 MyCollection 已在 mydatabase 中创建。
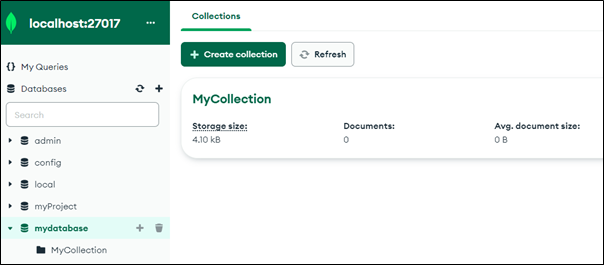
您也可以在 MongoDB shell 中验证相同的内容。
> use mydatabase < switched to db mydatabase > show collections < MyCollection
广告