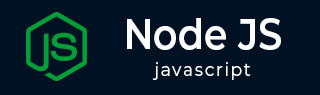
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL WHERE 条件
- Node.js - MySQL ORDER BY 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL JOIN 连接
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查询数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 限制结果
- Node.js - MongoDB 连接
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - 回调函数概念
什么是回调函数?
在 Node.js 中,回调函数是函数的异步等效项。它是一种特殊类型的函数,作为参数传递给另一个函数。Node.js 广泛使用回调函数。回调函数帮助我们进行异步调用。Node 的所有 API 都是以支持回调函数的方式编写的。
默认情况下,编程指令是同步执行的。如果程序中的某个指令需要执行冗长的过程,则主执行线程会被阻塞。只有在当前 I/O 完成后才能执行后续指令。这就是回调函数发挥作用的地方。
当包含回调函数作为参数的函数完成执行时,就会调用回调函数,并允许代码在此期间运行。这使得 Node.js 具有高度的可扩展性,因为它可以处理大量请求,而无需等待任何函数返回结果。
在 Node.js 中实现回调函数的语法如下:
function function_name(argument, function (callback_argument){ // callback body })
Node.js 中的 `setTimeout()` 函数是回调函数的典型示例。以下代码调用异步 `setTimeout()` 方法,等待 1000 毫秒,但不会阻塞线程。相反,后续的“Hello World”消息会在计时消息之前显示。
示例
setTimeout(function () { console.log('This prints after 1000 ms'); }, 1000); console.log("Hello World");
输出
Hello World This prints after 1000 ms
阻塞代码示例
要理解回调函数的功能,请将以下文本保存为 input.txt 文件。
TutorialsPoint is the largest free online tutorials Library Master any technology. From programming languages and web development to data science and cybersecurity
以下代码使用 fs 模块中的 `readFileSync()` 函数同步读取文件。由于操作是同步的,它会阻塞其余代码的执行。
var fs = require("fs"); var data = fs.readFileSync('input.txt'); console.log(data.toString()); let i = 1; while (i <=5) { console.log("The number is " + i); i++; }
输出显示 Node.js 读取文件并显示其内容。只有在此之后,才会执行打印数字 1 到 5 的循环。
TutorialsPoint is the largest free online tutorials Library Master any technology. From programming languages and web development to data science and cybersecurity The number is 1 The number is 2 The number is 3 The number is 4 The number is 5
非阻塞代码示例
我们在以下代码中使用相同的 input.txt 文件来演示回调函数的使用。
TutorialsPoint is the largest free online tutorials Library Master any technology. From programming languages and web development to data science and cybersecurity
fs 模块中的 `ReadFile()` 函数提供了一个回调函数。传递给回调函数的两个参数是错误和 `ReadFile()` 函数本身的返回值。当 `ReadFile()` 完成执行时,通过返回错误或文件内容来调用回调函数。在文件读取操作进行时,Node.js 会异步运行后续循环。
var fs = require("fs"); fs.readFile('input.txt', function (err, data) { if (err) return console.error(err); console.log(data.toString()); }); let i = 1; while (i <=5) { console.log("The number is " + i); i++; }
输出
The number is 1 The number is 2 The number is 3 The number is 4 The number is 5 TutorialsPoint is the largest free online tutorials Library Master any technology. From programming languages and web development to data science and cybersecurity
箭头函数作为回调函数
您还可以将箭头函数赋值为回调函数参数。JavaScript 中的箭头函数是匿名函数。它也称为 lambda 函数。在 Node.js 中使用箭头函数作为回调函数的语法如下:
function function_name(argument, (callback_argument) => { // callback body })
它是在 JavaScript ES6 版本中引入的。让我们用箭头函数替换上述示例中的回调函数。
var fs = require("fs"); fs.readFile('input.txt', (err, data) => { if (err) return console.error(err); console.log(data.toString()); }); let i = 1; while (i <=5) { console.log("The number is " + i); i++; }
上述代码产生的输出与之前的示例类似。