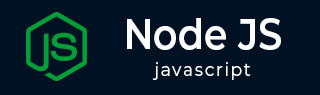
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 第一个应用
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 扩展应用
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTFul API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 从表中选择数据
- Node.js - MySQL where 条件
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 联接
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 限制结果数量
- Node.js - MongoDB 联接
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - 流
什么是流?
流是数据的集合。但是,与数组或字符串不同,流对象中的所有数据不会一次性存储在内存中。相反,一次只将流中的单个数据块加载到内存中。这使得流更加高效。Node.js 应用非常适合开发数据流应用。
流的类型
Node.js 处理四种基本的流类型:
可写流 - 可以写入数据的流。
可读流 - 可以从中读取数据的流。
双工流 - 既是可读流又是可写流的流。
转换流 - 可以在写入和读取数据时修改或转换数据的双工流。
每种类型的流都是 EventEmitter 实例,并在不同的时间实例中触发多个事件。例如,一些常用的事件包括:
data - 当有数据可供读取时触发此事件。
end - 当没有更多数据可供读取时触发此事件。
error - 当接收或写入数据时发生任何错误时触发此事件。
finish - 当所有数据都已刷新到底层系统时触发此事件。
本章中的示例使用名为 input.text 的文件,其中包含以下数据。
Tutorials Point is giving self learning content to teach the world in simple and easy way!!!!!
可读流
文件对象充当一个流,可以从中以特定大小的块读取数据。在下面的示例中,我们从 fs 模块调用 createReadStream() 函数以从给定文件读取数据。可读流的 on 事件收集文件内容,直到触发 end 事件。
var fs = require("fs"); var data = ''; // Create a readable stream var readerStream = fs.createReadStream('input.txt'); // Set the encoding to be utf8. readerStream.setEncoding('UTF8'); // Handle stream events --> data, end, and error readerStream.on('data', function(chunk) { data += chunk; }); readerStream.on('end',function() { console.log(data); }); readerStream.on('error', function(err) { console.log(err.stack); }); console.log("Program Ended");
将上述脚本保存为 main.js。运行上述 Node.js 应用。它将显示 input.text 文件的内容。
可写流
fs 模块中的 createWriteStream() 函数创建一个可写流对象。它的 write() 方法将数据存储在作为参数传递给 createWriteStream() 函数的文件中。
将以下代码保存为名为 main.js 的文件。
var fs = require("fs"); var data = `Tutorials Point is giving self learning content to teach the world in simple and easy way!!!!!`; // Create a writable stream var writerStream = fs.createWriteStream('output.txt'); // Write the data to stream with encoding to be utf8 writerStream.write(data,'UTF8'); // Mark the end of file writerStream.end(); // Handle stream events --> finish, and error writerStream.on('finish', function() { console.log("Write completed."); }); writerStream.on('error', function(err){ console.log(err.stack); }); console.log("Program Ended");
现在打开在当前目录中创建的 output.txt 文件,以检查它是否包含给定的数据。
管道流
管道是一种机制,我们将其提供一个流的输出作为另一个流的输入。它通常用于从一个流获取数据并将该流的输出传递给另一个流。管道操作没有限制。现在我们将展示一个从一个文件读取并将其写入另一个文件的管道示例。
创建一个名为 main.js 的 js 文件,其中包含以下代码:
var fs = require("fs"); // Create a readable stream var readerStream = fs.createReadStream('input.txt'); // Create a writable stream var writerStream = fs.createWriteStream('output.txt'); // Pipe the read and write operations // read input.txt and write data to output.txt readerStream.pipe(writerStream); console.log("Program Ended");
现在运行 main.js 以查看结果:
node main.js
验证输出。
Program Ended
打开在当前目录中创建的 output.txt 文件;它应该包含以下内容:
Tutorials Point is giving self learning content to teach the world in simple and easy way!!!!!
链接流
链接是一种将一个流的输出连接到另一个流并创建多个流操作链的机制。它通常与管道操作一起使用。现在我们将使用管道和链接首先压缩一个文件,然后解压缩同一个文件。
创建一个名为 main.js 的 js 文件,其中包含以下代码:
var fs = require("fs"); var zlib = require('zlib'); // Compress the file input.txt to input.txt.gz fs.createReadStream('input.txt') .pipe(zlib.createGzip()) .pipe(fs.createWriteStream('input.txt.gz')); console.log("File Compressed.");
现在运行 main.js 以查看结果:
node main.js
验证输出。
File Compressed.
您会发现 input.txt 已被压缩,并在当前目录中创建了一个名为 input.txt.gz 的文件。现在让我们尝试使用以下代码解压缩同一个文件:
var fs = require("fs"); var zlib = require('zlib'); // Decompress the file input.txt.gz to input.txt fs.createReadStream('input.txt.gz') .pipe(zlib.createGunzip()) .pipe(fs.createWriteStream('input.txt')); console.log("File Decompressed.");
现在运行 main.js 以查看结果:
node main.js
验证输出。
File Decompressed.