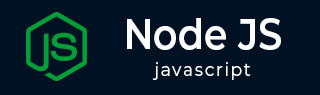
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL 条件查询
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 连接查询
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 数据限制
- Node.js - MongoDB 连接查询
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用工具模块
- Node.js - Web 模块
- Node.js 资源推荐
- Node.js - 快速指南
- Node.js - 资源推荐
- Node.js - 讨论
Node.js - 应用扩展
Node.js 应用的可扩展性是指它处理服务器上不断增加的工作负载的能力,即处理来自客户端的更多请求。Node.js 应用可以使用子进程来解决这个问题。子进程是 Node.js 运行时的单独实例,可以由主进程生成和管理。这样生成的子进程可以并行执行特定任务,从而提高应用程序的整体性能和可扩展性。
child_process 模块是 Node.js 运行时的核心模块之一。此模块提供各种方法来创建、管理和与子进程通信,尤其是在基于多核 CPU 的系统上。
子进程始终具有三个流:child.stdin、child.stdout 和 child.stderr。这些流可以与父进程的流共享。
child_process 模块有以下三种主要方法来创建子进程。
exec − child_process.exec 方法在 shell/控制台中运行命令并缓冲输出。
spawn − child_process.spawn 启动一个具有给定命令的新进程。
fork − child_process.fork 方法是 spawn() 的一个特例,用于创建子进程。
exec() 方法
child_process.exec 方法在 shell 中运行命令并缓冲输出。它具有以下签名:
child_process.exec(command[, options], callback)
参数
command − 要运行的命令,使用空格分隔的参数。
options − cwd- 子进程的当前工作目录,以及 env - 环境键值对
encoding − 编码字符串(默认值:'utf8')
shell − 用于执行命令的 shell(UNIX 上默认为 '/bin/sh',Windows 上默认为 'cmd.exe',shell 应该理解 UNIX 上的 -c 开关或 Windows 上的 /s /c。在 Windows 上,命令行解析应与 cmd.exe 兼容。)
timeout − 默认值:0
maxBuffer − 默认值:200*1024
killSignal − 默认值:'SIGTERM'
uid − 设置进程的用户标识。
gid − 设置进程的组标识。
callback − 一个具有三个参数 error、stdout 和 stderr 的函数,在进程终止时使用输出调用。
exec() 方法返回一个具有最大大小的缓冲区,等待进程结束并尝试一次返回所有缓冲数据。
示例
让我们创建两个名为 child.js 和 main.js 的 js 文件:
文件:child.js
console.log("Starting Child Process-" + process.argv[2]); console.log("Child Process-" + process.argv[2] + " executed." );
文件:main.js
const fs = require('fs'); const child_process = require('child_process'); for(var i=0; i<3; i++) { var childprocess = child_process.exec('node child.js '+i,function (error, stdout, stderr) { if (error) { console.log(error.stack); console.log('Error code: '+error.code); console.log('Signal received: '+error.signal); } console.log('stdout: ' + stdout); console.log('stderr: ' + stderr); }); childprocess.on('exit', function (code) { console.log('Child process exited with exit code '+code); }); }
现在运行 main.js 查看结果:
Child process exited with exit code 0 stdout: Starting Child Process-0 Child Process-0 executed. stderr: Child process exited with exit code 0 stdout: Starting Child Process-1 Child Process-1 executed. stderr: Child process exited with exit code 0 stdout: Starting Child Process-2 Child Process-2 executed. stderr:
spawn() 方法
child_process.spawn 方法使用给定的命令启动一个新进程。
child_process.spawn(command[, args][, options])
第一个参数是子进程要运行的命令。可以向其传递一个或多个参数。options 参数可能具有以下一个或多个属性:
cwd (字符串) 子进程的当前工作目录。
env (对象) 环境键值对。
stdio (数组) 字符串 子进程的 stdio 配置。
customFds (数组) 已弃用 子进程用于 stdio 的文件描述符。
detached (布尔值) 子进程将成为进程组领导者。
uid (数字) 设置进程的用户标识。
gid (数字) 设置进程的组标识。
spawn() 方法返回流 (stdout & stderr),当进程返回大量数据时应使用它。spawn() 在进程开始执行后立即开始接收响应。
示例
与之前的示例一样,创建 child.js 和 main.js 文件。
child.js
console.log("Starting Child Process-" + process.argv[2]); console.log("Child Process-" + process.argv[2] + " executed." );
main.js
const fs = require('fs'); const child_process = require('child_process'); for(var i=0; i<3; i++) { var childprocess = child_process.spawn('node', ['child.js', i]); childprocess.stdout.on('data', function (data) { console.log('stdout: ' + data); }); childprocess.on('exit', function (code) { console.log('Child process exited with exit code '+code); }); }
输出
stdout: Starting Child Process-0 stdout: Child Process-0 executed. Child process exited with exit code 0 stdout: Starting Child Process-1 stdout: Child Process-1 executed. stdout: Starting Child Process-2 stdout: Child Process-2 executed. Child process exited with exit code 0 Child process exited with exit code 0
fork() 方法
child_process.fork 方法是 spawn() 的一个特例,用于创建 Node 进程。它具有以下语法:
child_process.fork(modulePath[, args][, options])
参数
第一个参数是子进程要运行的模块。您可以向模块传递其他参数。选项与 spawn() 方法中的选项相同。
示例
与之前的示例一样,创建 child.js 和 main.js 文件。
child.js
console.log("Starting Child Process-" + process.argv[2]); console.log("Child Process-" + process.argv[2] + " executed." );
main.js
const fs = require('fs'); const child_process = require('child_process'); for(var i=0; i<3; i++) { var childprocess = child_process.fork("child.js", [i]); childprocess.on('close', function (code) { console.log('child process exited with code ' + code); }); }
输出
Starting Child Process-0 Child Process-0 executed. Starting Child Process-2 Child Process-2 executed. Starting Child Process-1 Child Process-1 executed. child process exited with code 0 child process exited with code 0 child process exited with code 0
execFile() 方法
child_process.execFile() 函数类似于 exec() 方法,不同之处在于它默认情况下不会生成 shell。它比 exec() 更有效率,因为它直接将指定的可执行文件作为新进程生成。
语法
child_process.execFile(command [, args][, options][, callback])
参数
command − 接受一个字符串,指定要运行的命令的名称或路径。
args − 字符串参数列表。
options − 一些可用的选项包括 cwd、env、encoding、shell、timeout 等
callback − 当进程终止时调用回调函数。此函数的参数分别是 error、stdout 和 stderr。