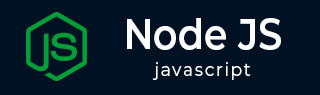
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL 数据库创建
- Node.js - MySQL 表创建
- Node.js - MySQL 数据插入
- Node.js - MySQL 数据查询
- Node.js - MySQL 条件查询 (Where)
- Node.js - MySQL 排序 (Order By)
- Node.js - MySQL 数据删除
- Node.js - MySQL 数据更新
- Node.js - MySQL 连接查询 (Join)
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 数据库创建
- Node.js - MongoDB 集合创建
- Node.js - MongoDB 数据插入
- Node.js - MongoDB 数据查找
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 数据删除
- Node.js - MongoDB 数据更新
- Node.js - MongoDB 数据限制 (Limit)
- Node.js - MongoDB 连接查询 (Join)
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - MongoDB 数据库创建
MongoDB 是一个开源的、跨平台的面向文档的数据库环境。MongoDB 使用类似 JSON 的、无模式的文档。多个文档构成一个集合,一个或多个集合可以存在于 MongoDB 数据库中。在本章中,我们将学习如何使用 Node.js 应用程序创建 MongoDB 数据库。
MongoDB 数据库管理软件提供以下三个版本:
MongoDB 社区版 - MongoDB 的源代码可用、免费使用和自管理版本,可用于本地安装,适用于 Windows、Linux 和 macOS。
MongoDB 企业版 - MongoDB 的商业版、订阅版、自管理版本,具有比社区版更多的高级功能。
MongoDB Atlas - MongoDB 部署的按需、完全托管的云服务。它运行在 AWS、Microsoft Azure 和 Google Cloud Platform 上。
连接字符串
Node.js 的 mongodb 驱动程序使用 require() 函数导入到代码中。MongoClient 类的对象表示数据库连接。您需要将连接字符串传递给其构造函数。
const { MongoClient } = require('mongodb'); const client = new MongoClient(ConnectionString);
MongoDB 连接字符串必须采用以下格式之一:
标准连接字符串格式 - 此格式用于连接到自托管的 MongoDB 单机部署、副本集或分片集群。标准 URI 连接方案具有以下形式:
mongodb://[username:password@]host1[:port1][,...hostN[:portN]][/[defaultauthdb][?options]]
默认连接字符串为:
mongodb://127.0.0.1:27017/
SRV 连接格式 - 连接字符串的主机名对应于 DNS SRV 记录。MongoDB Atlas 使用 SRV 连接格式。MongoDB 支持 DNS 构造的种子列表。它允许更大的部署灵活性以及在无需重新配置客户端的情况下轮换服务器的能力。SRV URI 连接方案具有以下形式:
mongodb+srv://[username:password@]host[/[defaultauthdb][?options]]
这是一个 SRV 连接字符串的示例:
const uri = "mongodb+srv://user:[email protected]/?retryWrites=true&w=majority";
在下面的示例中,我们使用标准连接字符串来获取数据库列表。
示例:数据库列表
MongoClient 对象的 connect() 方法返回一个 Promise。对 connect() 方法的调用是异步的。接下来调用 listdatabases() 函数。
const { MongoClient } = require('mongodb'); async function main() { const uri = "mongodb://127.0.0.1:27017/"; const client = new MongoClient(uri); try { // Connect to the MongoDB cluster await client.connect(); // Make the appropriate DB calls await listDatabases(client); } catch (e) { console.error(e); } finally { // Close the connection to the MongoDB cluster await client.close(); } } main().catch(console.error); async function listDatabases(client) { databasesList = await client.db().admin().listDatabases(); console.log("Databases:"); databasesList.databases.forEach(db => console.log(` - ${db.name}`)); };
输出
Databases: - admin - config - local - myProject - mydb
创建新的数据库
要在服务器上创建新的数据库,您需要调用 MongoClient 类的 db() 方法。
var dbobj = client.db(database_name, options);
db() 方法返回一个 db 实例,该实例代表服务器上的数据库。在下面的示例中,我们演示了如何在 MongoDB 中创建一个名为 mydatabase 的新数据库。
const {MongoClient} = require('mongodb'); async function main(){ const uri = "mongodb://127.0.0.1:27017/mydb"; const client = new MongoClient(uri); try { // Connect to the MongoDB cluster await client.connect(); await createdb(client, "mydatabase"); } finally { // Close the connection to the MongoDB cluster await client.close(); } } main().catch(console.error); async function createdb(client, dbname){ const dbobj = await client.db(dbname); console.log("Database created"); console.log(dbobj); }
但是,请注意,数据库不会在服务器上物理创建,除非至少创建了一个集合,并且其中至少包含一个文档。