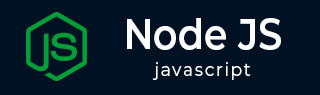
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTFul API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL 条件查询
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 连接查询
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 限制结果
- Node.js - MongoDB 连接查询
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - 打包
一个大型的 Node.js 项目通常包含许多依赖项和源文件,以及其他资源,例如图像、网页、设置文件等。分发 Node.js 项目并在任何其他环境中部署它变得很困难,因此需要对其进行打包,以便可以轻松地移植到其他机器。NPM 存储库中提供了许多打包工具。本章讨论 nexe 打包工具,并概述其他一些打包库。
Nexe
为了演示 Nexe 实用程序的工作原理,我们将使用以下脚本创建一个 ExpressJs 应用程序:
Index.js
var express = require('express'); var app = express(); var path = require('path'); var bodyParser = require('body-parser'); // Create application/x-www-form-urlencoded parser var urlencodedParser = bodyParser.urlencoded({ extended: false }) app.use(express.static('public')); app.get('/', function (req, res) { res.sendFile(path.join(__dirname,"index.html")); }) app.get('/process_get', function (req, res) { // Prepare output in JSON format response = { first_name:req.query.first_name, last_name:req.query.last_name }; console.log(response); res.end(JSON.stringify(response)); }) app.post("/process_post", ) var server = app.listen(5000, function () { console.log("Express App running at http://127.0.0.1:5000/"); })
“/” 路由从以下脚本呈现 HTML 表单:
Index.html
<html> <body> <form action = "/process_POST" method = "POST"> First Name: <input type = "text" name = "first_name"> <br> Last Name: <input type = "text" name = "last_name"> <br> <input type = "submit" value = "Submit"> </form> </body> </html>
上述 Node.js 服务器显示以下表单:
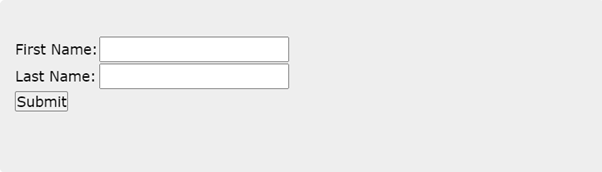
上面的 Node.js 应用程序还注册了静态中间件,因此它显示了放置在 static/images 文件夹中的图像。
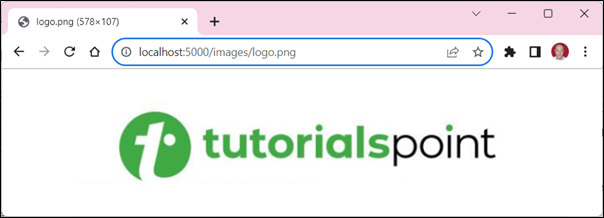
现在,我们希望为这个 Node.js 应用程序构建一个自执行文件,该文件将代码、子目录以及资源打包到一个文件中。Nexe 是一种命令行实用程序,可将您的 Node.js 应用程序编译成单个可执行文件。
Nexe 的特性
自包含应用程序
能够使用不同的 Node.js 运行时运行多个应用程序。
分发二进制文件,无需 Node/npm。
更快地启动和部署。
灵活的构建管道
跨平台构建
在 Windows 上安装 Nexe
执行以下操作以全局安装 Nexe:
npm install nexe -g
您还需要 Netwide Assembler (NASM) 工具。从 www.nasm.us 下载并安装。
nexe 实用程序需要系统上安装 Python。确保 Python 版本为 3.11 到 3.7 之间。
假设您正在将 nexe 用于 64 位 Windows 操作系统,您还需要从 Visual Studio 2022 安装“使用 C++ 的桌面开发”工作负载。可以从 aka.ms 安装。
(对于其他操作系统平台,请按照 github.com/nodejs 上的说明操作)
安装完所有先决条件后,在 CMD 终端中运行以下命令:
D:\expressApp>nexe index.js --build windows-x64-20.9.0 –verbose
编译可能需要一段时间,但最终它将在应用程序文件夹中创建 expressApp.exe。
D:\expressApp │ expressApp.exe │ index.html │ index.js │ package-lock.json │ package.json │ users.json │ ├───node_modules │ │ .package-lock.json │ ├───body-parser │ │ │ HISTORY.md │ │ │ index.js │ │ │ LICENSE │ │ │ package.json │ │ │ README.md │ │ │ SECURITY.md │ │ │ . . . │ │ │ . . . │ │ │ . . . │ ├───express │ │ │ History.md │ │ │ index.js │ │ │ LICENSE │ │ │ package.json │ │ │ Readme.md │ │ │ . . . │ │ │ . . . │ │ │ . . . └───public └───images logo.png
从命令行运行它,Node.js 服务器将启动。
D:\expressApp>expressapp Express App running at http://127.0.0.1:5000/
pkg 工具
pkg 工具是一个命令行界面,使开发人员能够从 Node.JS 项目创建可执行文件;允许您即使在未安装 Node.JS 的环境中运行应用程序。
要安装 pkg,请使用以下命令:
npm install -g pkg
然后使用 pkg 构建可执行文件
pkg myapp.js
运行上述命令将生成三个程序;即 Windows、macOS 和 Linux 的可执行文件。更多详细信息可以在 www.npmjs.com 上找到。
JXCore
JXcore 是一个开源项目,它为将源文件和其他资源打包和加密到 JX 包中引入了独特的功能。
根据您的操作系统和机器架构,从 https://github.com/jxcore 下载并安装 JXcore 包。
要打包上述项目,您只需进入此目录并发出以下 jx 命令。假设 index.js 是 Node.js 项目的入口文件:
jx package index.js index
上述命令将打包所有内容,并创建以下两个文件:
index.jxp - 这是一个中间文件,包含编译项目所需完整的项目详细信息。
index.jx - 这是一个包含完整包的二进制文件,已准备好交付给您的客户或生产环境。
假设您的原始 Node.js 项目正在按以下方式运行:
node index.js command_line_arguments
使用 JXcore 编译您的包后,可以按以下方式启动它:
jx index.jx command_line_arguments