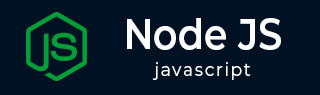
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL 条件查询
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 连接查询
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 数据限制
- Node.js - MongoDB 连接查询
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - doesNotMatch() 函数
Node.js 的 `assert.doesNotMatch()` 函数预期作为参数传入的 *字符串* 不匹配作为第二个参数传入的正则表达式。
这是 Node.js 的 `assert` 模块的内置函数。
语法
以下是 **Node.js `assert.doesNotMatch()` 函数** 的语法:
assert.doesNotMatch(string, regexp[, message]);
参数
此函数接受三个参数。具体描述如下。
**字符串** - (必填) 此参数仅接受字符串输入,否则将向输出抛出 AssertionError。
**正则表达式** - (必填) 在此参数中,需要传入一个正则表达式,该表达式不应与 **字符串** 匹配。如果两个输入(字符串和正则表达式)相同,则会向输出抛出 AssertionError。
**消息** - (可选) 可以将字符串或 Error 类型作为输入传递到此参数。
返回值
当两个输入(字符串和正则表达式)相同时,此方法将向输出抛出 AssertionError。
示例
在下面的示例中,我们将 **字符串** 和 **正则表达式** 值以及 **消息** 传递给 Node.js `assert.doesNotMatch()` 函数。
const assert = require('assert'); var str = 'Tutorialspoint'; var reg = /E-learning platform/; assert.doesNotMatch(str, reg, 'Both are not matching');
输出
当我们编译并运行代码时,该函数不会向输出抛出任何 AssertionError,因为字符串和正则表达式不相等。
// Returns nothing
示例
在下面的示例中,我们将 **字符串** 和 **正则表达式** 值以及 **消息** 传递给 *Node.js `assert.doesNotMatch()`* 函数。但是我们传递给 **字符串** 的值是一个整数。
const assert = require('assert'); var str = 96864; var reg = /E-learning platform/; assert.doesNotMatch(str, reg, 'Integer is passes instead of string');
输出
当我们编译并运行代码时,该函数将抛出 *AssertionError* 以及 *消息* 到输出,因为 **字符串** 输入值必须只能是 *字符串*。
/home/cg/root/639c2bf348ea8/main.js:6 assert.doesNotMatch(str, reg, 'Integer is passes instead of string'); ^ TypeError: assert.doesNotMatch is not a function at Object.<anonymous> (/home/cg/root/639c2bf348ea8/main.js:6:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
示例
在下面的示例中,我们将 **字符串** 和 **正则表达式** 传递给 *Node.js `assert.doesNotMatch()`* 函数进行比较。但是我们没有在 *消息* 参数中传递任何文本。
const assert = require('assert'); var str = 436; var reg = /Number/; assert.doesNotMatch(str, reg);
输出
当我们编译并运行代码时,该函数将分配一个默认错误消息。
/home/cg/root/639c2bf348ea8/main.js:6 assert.doesNotMatch(str, reg); ^ TypeError: assert.doesNotMatch is not a function at Object.<anonymous> (/home/cg/root/639c2bf348ea8/main.js:6:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12)at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
示例
在下面的示例中,我们将 **字符串** 和 **正则表达式** 值以及 **消息** 传递给 *Node.js `assert.doesNotMatch()`* 函数。
const assert = require('assert'); var str = 'Bahubali'; var reg = /Bahubali/; assert.doesNotMatch(str, reg, 'Both the values are exactly same');
输出
当我们编译并运行代码时,该函数将向输出抛出 *AssertionError*,因为 *字符串* 和 *正则表达式* 相同。
/home/cg/root/639c2bf348ea8/main.js:6 assert.doesNotMatch(str, reg, 'Both the values are exactly same'); ^ TypeError: assert.doesNotMatch is not a function at Object.<anonymous> (/home/cg/root/639c2bf348ea8/main.js:6:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
示例
在下面的示例中,我们将 **字符串** 和 **正则表达式** 值以及 **消息** 传递给 *Node.js `assert.doesNotMatch()`* 函数。
const assert = require('assert'); var str = 'Good to see you back officer'; var reg = /see/; assert.doesNotMatch(str, reg, 'Both the values are partially same');
输出
当我们编译并运行代码时,该函数将向输出抛出 *AssertionError*,因为 *字符串* 和 *正则表达式* 部分相同。
/home/cg/root/639c2bf348ea8/main.js:6 assert.doesNotMatch(str, reg, 'Both the values are partially same'); ^ TypeError: assert.doesNotMatch is not a function at Object.<anonymous> (/home/cg/root/639c2bf348ea8/main.js:6:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)