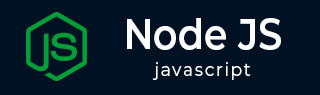
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTFul API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 从表中选择数据
- Node.js - MySQL Where 条件
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 连接
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 限制结果
- Node.js - MongoDB 连接
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - assert.doesNotReject() 函数
Node.js assert.doesNotReject() 函数是 Node.js 的 assert 模块的内置函数。它用于检查给定的 Promise 是否没有被拒绝。
在 assert.doesNotReject() 函数中,如果提供的参数是 Promise,则等待 Promise 完成。如果提供的参数是函数,则立即调用该函数并等待返回的 Promise 完成。此函数将检查提供的 Promise 是否被拒绝。此函数的行为与 assert.doesNotThrow() 相同。
assert.doesNotReject() 的实用性不大,因为捕获错误并再次拒绝错误的好处很少。相反,如果我们为不应该被拒绝的特定代码路径添加适当的注释,并尽可能使错误消息具有表达性,会更好。
语法
以下是 Node.js assert.doesNotReject() 函数 的语法:
assert.doesNotReject(asyncFn[, error][, message]);
参数
此函数接受三个参数。如下所述。
asyncFn − (必需) 此参数包含一个异步函数,该函数将同步抛出错误。
error − (可选) 此参数可以包含类、正则表达式、验证函数或对象,其中每个属性都将被测试。
message − (可选) 此参数可以包含类、正则表达式、验证函数或对象,其中每个属性都将被测试。
返回值
函数 assert.doesNotReject() 将把被拒绝的 Promise 返回到输出。
示例
以下是 Node.js assert.doesNotReject() 函数的使用方法。
const assert = require('assert'); (async () => { await assert.doesNotReject( async () => { throw new TypeError('Wrong value'); }, TypeError ); } )();
输出
以下是上述代码的输出:
(node:40094) UnhandledPromiseRejectionWarning: AssertionError [ERR_ASSERTION]: Got unwanted rejection. Actual message: "Wrong value" at process._tickCallback (internal/process/next_tick.js:68:7) at Function.Module.runMain (internal/modules/cjs/loader.js:746:11) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3) (node:40094) UnhandledPromiseRejectionWarning: Unhandled promise rejection. This error originated either by throwing inside of an async function without a catch block, or by rejecting a promise which was not handled with .catch(). (rejection id: 2) (node:40094) [DEP0018] DeprecationWarning: Unhandled promise rejections are deprecated. In the future, promise rejections that are not handled will terminate the Node.js process with a non-zero exit code.
示例
以下是 Node.js assert.doesNotReject() 函数在另一种场景下的使用方法。
const assert = require('assert'); (async () => { await assert.doesNotReject( async () => { throw new TypeError('Error occured!!!'); }, SyntaxError ); } )();
输出
以下是上述代码的输出:
(node:43403) UnhandledPromiseRejectionWarning: TypeError: Error occured!!! at assert.doesNotReject (/home/cg/root/639c2bf348ea8/main.js:6:23) at waitForActual (assert.js:518:21) at Function.doesNotReject (assert.js:620:39)at /home/cg/root/639c2bf348ea8/main.js:4:22 at Object.<anonymous> (/home/cg/root/639c2bf348ea8/main.js:11:2) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) (node:43403) UnhandledPromiseRejectionWarning: Unhandled promise rejection. This error originated either by throwing inside of an async function without a catch block, or by rejecting a promise which was not handled with .catch(). (rejection id: 2) (node:43403) [DEP0018] DeprecationWarning: Unhandled promise rejections are deprecated. In the future, promise rejections that are not handled will terminate the Node.js process with a non-zero exit code.
nodejs_assert_module.htm
广告