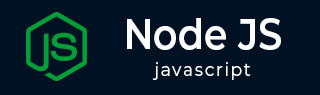
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL 条件查询
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 连接查询
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 数据限制
- Node.js - MongoDB 连接查询
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用工具模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - assert.notDeepEqual() 函数
assert 模块提供了一组断言函数,用于验证不变式。Node.js assert.notDeepEqual() 函数是 Node.js assert 模块的内置函数。
Node.js assert.notDeepEqual() 函数 将比较传入的值是否深度相等。如果值深度相等,则函数将抛出一个包含消息参数中文本的 AssertionError。否则,如果值不相等,则不会抛出任何 AssertionError,也不会返回任何输出。
此方法与 assert.notDeepStrictEqual() 函数几乎相同,并且是 assert.deepEqual() 函数的反义。
语法
以下是 Node.js assert.notDeepEqual() 函数 的语法:
assert.notDeepEqual(actual, expected[, message]);
参数
此方法接受三个参数。具体说明如下。
actual − (必需) 此参数中传递的值将被评估。值可以是任何类型。
expected − (必需) 此参数中传递的值将与 actual 值 进行比较。值可以是任何类型。
message − (可选) 可以将字符串或错误类型作为输入传递到此参数中。
返回值
如果 actual 和 expected 匹配,则此函数将在终端返回一个 AssertionError。
示例
在下面的示例中,我们将两个不同的整数值作为 actual 和 expected 传递给 Node.js assert.notDeepEqual() 函数,并将文本传递给 message 参数。
const assert = require('assert'); var num1 = 34; var num2 = 45; assert.notDeepEqual(num1, num2, "Both the numbers are NOT EQUAL");
输出
当我们编译并运行代码时,该函数不会向输出抛出 AssertionError,因为 actual 和 expected 不匹配。
// Returns nothing
示例
在下面的示例中,我们将两个输入传递给 Node.js assert.notDeepEqual() 函数,一个整数传递给 actual 参数,一个字符串传递给 expected 参数。
const assert = require('assert'); var string1 = '7'; var num1 = 7; assert.notDeepEqual(string1, num1, "Both the values are EQUAL");
输出
当我们编译并运行代码时,该函数会向输出抛出 AssertionError,因为 7 == '7'。
assert.js:79 throw new AssertionError(obj); ^ AssertionError [ERR_ASSERTION]: Both the values are EQUAL at Object.<anonymous> (/home/cg/root/639c30a0a5fb2/main.js:6:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19)at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
示例
在下面的示例中,我们创建了两个具有不同属性的嵌套对象。然后,我们将这两个对象作为 actual 和 expected 传递给函数,并将文本传递给 message 参数。
const assert = require('assert'); var object1 = { Name : 'Mike', Id : 17, Salary : { "2020-2021" : '45000INR', "2021-2022" : '50000INR', }, address: { Area: { address1: "White field road", address2: "Brown wheat field", }, } }; var object2 = { Name : 'Mike', Id : 17, Salary : { "2020-2021" : '45000INR', "2021-2022" : '50000INR', }, address: { Area: { address1: "White field road", address2: "Brown wheat field", }, } }; assert.notDeepEqual(object1, object2, "Both the objects are EQUAL")
输出
当我们编译并运行上述程序时,它会抛出 AssertionError 和message 到输出,因为actual 和expected 是相同的。
assert.js:79 throw new AssertionError(obj); ^ AssertionError [ERR_ASSERTION]: Both the objects are EQUAL at Object.<anonymous> (/home/cg/root/639c30a0a5fb2/main.js:33:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
示例
在下面的示例中,我们创建了两个具有相似属性的嵌套对象。然后,我们将这两个对象作为 actual 和 expected 传递给函数,并将文本传递给 message 参数。
const assert = require('assert'); var object1 = { Name : 'John', Id : 27, Salary : { "2020-2021" : '55000INR', "2021-2022" : '70000INR', }, }; var object2 = { Name : 'Mike', Id : 17, Salary : { "2020-2021" : '45000INR', "2021-2022" : '50000INR', }, }; assert.notDeepEqual(object1, object2, "Both the objects are NOT EQUAL");
输出
因此,当我们编译并运行代码时,该函数不会向输出抛出 AssertionError,因为 actual 和 expected 不相同。
// Returns nothing