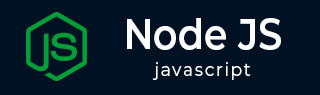
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL 条件查询
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 连接查询
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 数据限制
- Node.js - MongoDB 连接查询
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - assert.strictEqual() 函数
assert 模块提供了一组断言函数,用于验证不变式。Node.js 的 assert.strictEqual() 函数是 Node.js assert 模块的内置函数。
Node.js 的 strictEqual() 函数用于确定两个值是否相等,它使用比 == 运算符更严格的比较。此函数比较两个变量或对象的 数据类型和值,只有当它们完全相同 时才返回 true。此外,将 NaN(非数字)与任何其他值(包括自身)进行比较都将返回 false。
此函数将测试其两个参数 actual 和 expected 的相等性。当两个参数相同时,函数不会抛出 AssertionError。如果两个参数不相等,则它会将 AssertionError 抛出到输出。此函数是 Node.js assert.deepStrictEqual() 函数的别名。
语法
以下是 Node.js assert.strictEqual() 函数 的语法:
assert.strictEqual(actual, expected[, message]);
参数
此函数接受三个参数。如下所述。
actual − (必需)此参数中传递的值将被评估。值可以是任何类型。
expected − (必需)此参数中传递的值将与 actual 值进行比较。值可以是任何类型。
message − (可选)可以将字符串或 Error 类型作为输入传递到此参数。
返回值
如果 actual 和 expected 不匹配,此函数将在终端返回 AssertionError。
示例
在以下示例中,我们将两个相同的整数传递给 Node.js assert.strictEqual() 函数的 actual 和 expected 参数。
const assert = require('assert'); var num1 = 45; var num2 = 45; assert.strictEqual(num1, num2, 'Both the values are same');
输出
如果我们编译并运行代码,则不会将任何 AssertionError 抛出到输出。这是正确的,因为 45 == 45。
// Returns nothing
示例
在以下示例中,我们将两个不同的整数传递给函数的 actual 和 expected 参数。
const assert = require('assert'); var int1 = 34; var int2 = 10; assert.strictEqual(int1, int2, 'Both the values are not same');
输出
如果我们编译并运行代码,它将向输出抛出 AssertionError 以及 message 参数中的文本。这是错误的,因为 34 !== 10。
assert.js:79 throw new AssertionError(obj); ^ AssertionError [ERR_ASSERTION]: Both the values are not same at Object.<anonymous> (/home/cg/root/639c3f570123e/main.js:5:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
示例
在以下示例中,我们将两个相同的字符串传递给函数的 actual 和 expected 参数。
const assert = require('assert'); var text1 = 'Welcome to India'; var text2 = 'Welcome to India'; assert.strictEqual(text1, text2, 'Both the strings are identical');
输出
如果我们编译并运行代码,则不会将任何 AssertionError 抛出到输出。这是正确的,因为 text1 == text2。
// Returns nothing
示例
在以下示例中,我们传递两个字符串值并检查它们的类型。
const assert = require('assert'); var text1 = 'Rise'; var text2 = 'Rule'; assert.strictEqual(typeof text1 === 'string', typeof text2 === 'number', 'Both the strings are not identical');
输出
如果我们编译并运行代码,它将抛出一个 AssertionError 以及message中的文本到输出。这是错误的,因为'text2' == 'number'。
assert.js:79 throw new AssertionError(obj); ^ AssertionError [ERR_ASSERTION]: Both the strings are not identical at Object.<anonymous> (/home/cg/root/639c3f570123e/main.js:6:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
示例
在下面的示例中,我们传递两个不同的字符串值,并且没有向message参数传递任何文本。
const assert = require('assert'); assert.strictEqual('RRR', 'ZZZ');
输出
如果我们编译并运行代码,它将向输出抛出 AssertionError,并且函数将分配默认消息。
assert.js:79 throw new AssertionError(obj); ^ AssertionError [ERR_ASSERTION]: Input A expected to strictly equal input B: + expected - actual - 'RRR' + 'ZZZ' at Object.<anonymous> (/home/cg/root/639c3f570123e/main.js:3:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
示例
在下面的示例中,我们没有向函数的actual、expected和message参数传递任何输入值。
const assert = require('assert'); assert.strictEqual();
输出
// Returns Nothing
注意 - 有时在线编译器可能无法给出预期的结果,因此我们会在本地执行上述代码。
如果我们编译并运行代码,该函数将抛出 AssertionError,并显示默认消息“The 'actual' and 'expected' arguments must be specified”。
node:assert:568 throw new ERR_MISSING_ARGS('actual', 'expected'); ^ TypeError [ERR_MISSING_ARGS]: The "actual" and "expected" arguments must be specified at new NodeError (node:internal/errors:387:5) at Function.strictEqual (node:assert:568:11) at Object.<anonymous> (C:\Users\Lenovo\Desktop\JavaScript\nodefile.js:3:8) at Module._compile (node:internal/modules/cjs/loader:1126:14) at Object.Module._extensions..js (node:internal/modules/cjs/loader:1180:10) at Module.load (node:internal/modules/cjs/loader:1004:32) at Function.Module._load (node:internal/modules/cjs/loader:839:12) at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:81:12) at node:internal/main/run_main_module:17:47 { code: 'ERR_MISSING_ARGS' }