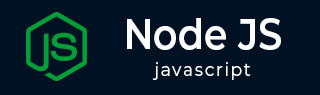
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区 (Buffers)
- Node.js - 流 (Streams)
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL WHERE 条件
- Node.js - MySQL ORDER BY 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL JOIN 连接
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 限制结果
- Node.js - MongoDB 连接
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用工具模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - Buffer.copy() 方法
NodeJS 的 Buffer.copy() 方法将源缓冲区对象的内容复制到目标缓冲区。即使两个缓冲区之间存在内存重叠,内容也会被复制。
语法
以下是 NodeJS copy() 方法的语法:
buf.copy( target, targetStart, sourceStart, sourceEnd )
参数
buffer.copy() 方法接受四个参数。第一个参数 target 是必需的,其余参数是可选的。
target −这是必需参数。它是目标缓冲区对象,源缓冲区对象将被复制到其中。
targetStart −它指的是目标缓冲区中开始写入的偏移量。默认为 0。
sourceStart −它指的是源缓冲区中开始复制的偏移量。默认为 0。
sourceEnd −它指的是源缓冲区中停止复制的偏移量。默认值为源缓冲区的长度。
返回值
buffer.copy() 方法返回已复制的字节数。
示例
使用 NodeJS buffer.copy() 方法将内容从一个缓冲区复制到另一个缓冲区。
var buffer1 = Buffer.from('testing'); var buffer2 = Buffer.from('HELLO'); buffer2.copy(buffer1); console.log("After copying the string in buffer1 is "+ buffer1.toString());
输出
在这个例子中,我们将缓冲区 buffer2 中的字符串 HELLO 复制到缓冲区 buffer1。当您打印 buffer1 的内容时,您将得到以下输出:
After copying the string in buf1 is HELLOng
示例
在这个例子中,我们将使用 targetStart 参数进行复制。
var buffer1 = Buffer.from('testing'); var buffer2 = Buffer.from('HELLO'); buffer2.copy(buffer1, 3); console.log("After copying the string in buffer1 is "+ buffer1.toString());
输出
在上面的例子中,我们使用了 targetStart 为 3。因此在 buffer1 中字符串是 testing,从偏移量 3 到字符串的末尾将复制 buffer2 的内容。
After copying the string in buffer1 is tesHELL
示例
在这个例子中,我们将使用 targetStart 和 sourceStart 来使用 buffer.copy() 复制内容。
var buffer1 = Buffer.from('testing'); var buffer2 = Buffer.from('HELLO'); buffer2.copy(buffer1, 2, 2); console.log("After copying the string in buffer1 is "+ buffer1.toString());
输出
在上面的例子中,我们使用了 targetStart 为 2。因此在 buffer1 中字符串是 testing,从偏移量 2 到字符串的末尾将复制 buffer2 的内容。我们使用了 sourceStart,所以 buffer2 中的字符串是 HELLO,要复制的内容将从偏移量 2 开始,即 LLO 将被复制到 buffer1。
After copying the string in buffer1 is teLLOng
示例
现在让我们使用 Buffer.copy() 方法的所有参数。
var buffer1 = Buffer.from('testing'); var buffer2 = Buffer.from('HELLO'); buffer2.copy(buffer1, 2, 0, 3); console.log("After copying the string in buffer1 is "+ buffer1.toString());
输出
在上面的例子中,我们使用了 tartgetStart、sourceStart 和 sourceEnd 参数。因此,从源缓冲区中选择的字符串是 HEL,它将从 buffer1 的偏移量 2 开始复制。
After copying the string in buffer1 is teHELng
示例
在这个例子中,我们将使用 Buffer.allocUnsafe() 方法创建一个缓冲区,然后使用 Unicode 值向其中添加字符。
var buffer1 = Buffer.allocUnsafe(10); var buffer2 = Buffer.from('HELLO'); for (let i = 0; i < 10; i++) { //109 is for character m, it will increment and n,o,p..will get added. buffer1[i] = i + 109; } console.log("The string in buffer1 is "+buffer1.toString()); buffer2.copy(buffer1, 2); console.log("The string in buffer1 is "+buffer1.toString());
输出
我们使用 Buffer.allocUnsafe() 在 buffer1 中分配了 10 个字节。之后,我们使用 for 循环从 0 到 10 循环,并将字符添加到 buffer1。buffer2 的内容将从 buffer1 中偏移量 2 开始复制到 buffer1 中。
当您执行上述代码时,输出如下所示:
The string in buffer1 is mnopqrstuv The string in buffer1 is mnHELLOtuv
示例
当您尝试使用 targetStart 和 sourceStart 参数的值小于零时,它将抛出错误。
让我们看看当我们使用 targetStart 和 sourceStart 小于零时的例子。
var buffer1 = Buffer.from('testing'); var buffer2 = Buffer.from('HELLO'); buffer2.copy(buffer1, -1, -1, 3); console.log("After copying the string in buffer1 is "+ buffer1.toString());
输出
由于我们使用了 targetStart 和 sourceStart 为 -1,您将得到上面的错误。默认值为 0,小于 0 将抛出错误,如下所示:
RangeError: Index out of range