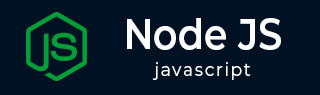
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区 (Buffers)
- Node.js - 流 (Streams)
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL 条件查询 (Where)
- Node.js - MySQL 排序 (Order By)
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 连接查询 (Join)
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 数据限制 (Limit)
- Node.js - MongoDB 连接查询 (Join)
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - Buffer.includes() 方法
NodeJS 的 `buffer.includes()` 方法检查给定值是否在缓冲区内。如果存在则返回 true,否则返回 false。
语法
以下是 NodeJS `includes()` 方法的语法:
buf.includes(value[, byteOffset][, encoding])
参数
`buffer.includes()` 方法接受三个参数。第一个参数是必需的,其余参数都是可选的。
value − 此参数是必需的。这是要在缓冲区内搜索的值。
byteOffset − 此参数是可选的。它指定从哪里开始搜索。如果给出负偏移值,则将从缓冲区的末尾开始搜索。默认值为 0。
encoding − 如果要搜索的值是字符串,则可以使用此参数指定编码。此参数是可选的。默认编码为 utf8。
返回值
如果在缓冲区中找到该值,则 `buffer.includes()` 方法返回 true;否则返回 false。
示例
在此示例中,我们将尝试在创建的缓冲区内搜索一个值并测试结果。
const buffer = Buffer.from('Welcome to TutorialsPoint'); if (buffer.includes('Welcome')) { console.log("The string welcome is present in the buffer"); } else { console.log("The string welcome is not present"); }
输出
我们正在搜索在包含字符串 "Welcome to Tutorialspoint" 的缓冲区中搜索字符串 Welcome。由于给定的字符串存在,因此我们得到以下输出。
The string welcome is present in the buffer
示例
让我们尝试在下面的示例中使用 `byteOffset` 参数:
const buffer = Buffer.from('Welcome to TutorialsPoint'); if (buffer.includes('Point', 10)) { console.log("The string point is present in the buffer"); } else { console.log("The string point is not present"); }
输出
我们使用了 10 作为 `byteOffset`。它将在字符串 "Welcome to TutorialsPoint" 中从偏移量 10 开始搜索。由于搜索字符串 "Point" 可用,您将得到输出 "字符串 Point 存在于缓冲区中"。
The string point is present in the buffer
示例
在此示例中,我们将使用一个缓冲区作为值来检查它是否在给定的缓冲区内。
const buffer = Buffer.from('Welcome to TutorialsPoint'); const result = buffer.includes(Buffer.from('TutorialsPoint')); if (result) { console.log("The string TutorialsPoint is present in the buffer"); } else { console.log("The string TutorialsPoint is not present"); }
输出
在 `Buffer.includes()` 方法中,我们使用了一个包含字符串值 TutorialsPoint 的缓冲区。我们的主缓冲区包含字符串 Welcome to TutorialsPoint。因此,由于字符串存在,返回的值为 true。
The string TutorialsPoint is present in the buffer
示例
让我们尝试一个 false 的情况,即检查当字符串不存在时 `Buffer.includes()` 的响应。
const buffer = Buffer.from('Welcome to TutorialsPoint'); if (buffer.includes('Testing')) { console.log("The string Testing is present in the buffer"); } else { console.log("The string Testing is not present"); }
输出
我们正在搜索字符串 Testing,它在包含字符串 "Welcome to TutorialsPoint" 的缓冲区中。由于它不存在,它将返回 false。因此,您将得到输出:字符串 Testing 不存在。
The string Testing is not present
示例
让我们测试 `Buffer.includes()` 中的 `encoding` 参数。
const buffer = Buffer.from('Hello World'); if (buffer.includes('Hello','hex')) { console.log("The string Hello is present in the buffer"); } else { console.log("The string Hello is not present"); }
输出
我们使用了 "hex" 编码。当使用编码检查并且字符串存在时,它返回 true。
The string Hello is present in the buffer
示例
在此示例中,我们将使用整数值,即 utf-8 编码值,在缓冲区中进行搜索。
const buffer = Buffer.from('Hello World'); if (buffer.includes(72)) { console.log("The character H is present in the buffer"); } else { console.log("The string H is not present"); }
输出
这里 72 代表根据 utf-8 编码的字符 'H'。当在缓冲区中使用整数值进行搜索时,它返回 true,因为字符 H 存在。
The string Hello is present in the buffer