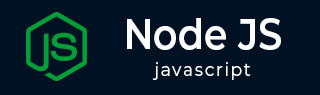
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTFul API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 从表中选择数据
- Node.js - MySQL 条件查询
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 联接
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 限制结果
- Node.js - MongoDB 联接
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - Buffer.lastIndexOf() 方法
NodeJS 的 Buffer.lastIndexOf() 方法用于在缓冲区内搜索某个值。它返回该值所在的位置,如果该值不存在则返回 -1。如果该值在缓冲区中出现多次,则返回最后出现的位置。
语法
以下是 NodeJS lastIndexOf() 方法的语法:
buffer.lastIndexOf(value, byteoffset, encoding);
参数
buffer.lastIndexOf() 方法接受三个参数。第一个参数是必填参数,其余两个参数是可选参数。
value − 这是必填参数。它是要在缓冲区内搜索的值。该值可以是字符串、数字或缓冲区。
byteOffset − 这是可选参数。它指定从哪里开始搜索。如果给出负偏移量,则将从缓冲区的末尾开始搜索。默认值为 buffer.length-1。
encoding − 如果要搜索的值是字符串,则可以使用此参数指定编码。这是一个可选参数。默认情况下使用的编码是 utf8。
返回值
Buffer.lastIndexOf() 方法将返回它遇到的最后一个搜索值的位置。如果该值不存在,则返回 -1。
示例
在此示例中,我们将尝试在创建的缓冲区内搜索某个值并测试结果。
const buffer = Buffer.from('Welcome to TutorialsPoint'); if (buffer.lastIndexOf('Welcome') != -1) { console.log("The string welcome is present in the buffer"); } else { console.log("The string welcome is not present"); }
输出
我们正在使用字符串“Welcome to Tutorialspoint”创建的缓冲区中搜索字符串 Welcome。由于给定的字符串存在,因此我们得到以下输出。我们检查该值是否不等于 -1。因为当我们在缓冲区中没有找到给定值的匹配项时,会返回 -1。
The string welcome is present in the buffer
示例
让我们尝试在下面的示例中给出 byteOffset 参数:
const buffer = Buffer.from('Welcome to TutorialsPoint'); if (buffer.lastIndexOf('Point', -1) != -1) { console.log("The string point is present in the buffer"); } else { console.log("The string point is not present"); }
输出
我们使用 -1 作为 byteOffset。它将从字符串“Welcome to TutorialsPoint”的末尾开始搜索。由于搜索字符串:Point 可用,您将获得输出打印为“字符串 point 存在于缓冲区中”。
The string point is present in the buffer
示例
在此示例中,我们将使用缓冲区作为值来检查它是否在给定的缓冲区内可用。
const buffer = Buffer.from('Welcome to TutorialsPoint'); const result = buffer.lastIndexOf(Buffer.from('TutorialsPoint')); if (result != -1) { console.log("The string TutorialsPoint is present in the buffer"); } else { console.log("The string TutorialsPoint is not present"); }
输出
在 Buffer.lastIndexOf() 方法内部,我们使用了一个包含字符串值:TutorialsPoint 的缓冲区。我们的主缓冲区包含一个字符串:Welcome to TutorialsPoint。因此,由于该字符串存在,返回的值是该字符串的起始位置。
The string TutorialsPoint is present in the buffer
示例
让我们尝试一个错误情况,即检查当字符串不存在时 Buffer.lastIndexOf() 的响应。
const buffer = Buffer.from('Welcome to TutorialsPoint'); if (buffer.lastIndexOf('Testing') != -1) { console.log("The string Testing is present in the buffer"); } else { console.log("The string Testing is not present"); }
输出
我们正在使用字符串:Welcome to TutorialsPoint 的缓冲区内搜索字符串:Testing。由于它不存在,它将返回 -1。因此,您将获得输出为:字符串 Testing 不存在。
The string Testing is not present
示例
让我们在 Buffer.lastIndexOf() 中测试 encoding 参数。
const buffer = Buffer.from('Hello World'); if (buffer.lastIndexOf('Hello','hex') != -1) { console.log("The string Hello is present in the buffer"); } else { console.log("The string Hello is not present"); }
输出
我们使用了“hex”编码。当使用编码进行检查且字符串存在时,它会返回字符串的起始位置。
The string Hello is present in the buffer
示例
在此示例中,我们将使用整数(即 utf-8 编码值)在缓冲区中进行搜索。
const buffer = Buffer.from('Hello World'); if (buffer.lastIndexOf(72) != -1) { console.log("The character H is present in the buffer"); } else { console.log("The string H is not present"); }
输出
这里 72 根据 utf-8 编码表示字符“H”。当在缓冲区中使用整数进行搜索时,它会返回字符 H 的位置,因为它存在于缓冲区中。
The string Hello is present in the buffer
示例
让我们检查一个示例,其中同一个字符串出现多次。
在示例中,我们使用 indexOf 和 lastIndexOf() 检查位置。使用 lastIndexOf(),它给出最后出现的字符串的位置,使用 indexOf(),它给出第一个出现的字符串的位置。
const buffer = Buffer.from('She sells sea shells on the sea shore'); console.log("The position of sea using lastIndexOf() is "+ buffer.lastIndexOf("sea")); console.log("The position of sea using indexOf() is "+ buffer.indexOf("sea"));
输出
The position of sea using lastIndexOf() is 28 The position of sea using indexOf() is 10