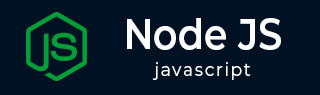
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL WHERE 条件
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL JOIN 连接
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 数据限制
- Node.js - MongoDB 连接
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - Buffer.writeInt16LE() 方法
Buffer.writeInt16LE() 方法用于以小端序的形式将一个带符号的 16 位整数写入缓冲区中的给定偏移量。写入缓冲区的整数表示为二进制补码带符号值。
16 位带符号整数的取值范围是 -32768 到 32767。
**二进制补码**是二进制数的一种数学运算。要获得任何给定二进制数的二进制补码,步骤如下:
二进制数: 101011
**步骤 1** - 首先找到二进制数 101011 的反码:要获得反码,你需要将 1 变成 0,将 0 变成 1。所以上面二进制数的反码是:010100。
**步骤 2** - 现在,对于步骤 1 中的反码,加上 1。
I.e. 010100 + 1 = 010101. So 010101 is the two's complement of binary number 101011.
语法
以下是 **Node.JS Buffer.writeInt16LE() 方法** 的语法:
buf.writeInt16LE(value[, offset])
参数
此方法接受两个参数。解释如下。
**value** - (必需)要写入缓冲区的带符号 16 位整数。
**offset** - 指示开始读取位置的偏移量。偏移量大于或等于 0,小于或等于 buffer.length-2。默认值为 0。
返回值
方法 **buffer.writeInt16LE()** 写入给定值并返回偏移量加上写入的字节数。
示例
要创建缓冲区,我们将使用 NodeJS Buffer.alloc() 方法:
const buffer = Buffer.alloc(10); buffer.writeInt16LE(0x0910, 0); console.log(buffer);
输出
我们使用的偏移量是 0。执行时,从第 0 位开始的值将被写入创建的缓冲区。上面创建的缓冲区长度为 10。因此,我们只能使用 0 到 8 的偏移量值。如果任何值 > 8,它将给出错误 **ERR_OUT_OF_RANGE**。
<Buffer 10 09 00 00 00 00 00 00 00 00>
示例
在这个例子中,我们将使用一个大于 buffer.length - 2 的偏移量。它应该抛出如下所示的错误。
const buffer = Buffer.alloc(10); buffer.writeInt16LE(0x0910, 9); console.log(buffer);
输出
internal/buffer.js:83 throw new ERR_OUT_OF_RANGE(type || 'offset', ^ RangeError [ERR_OUT_OF_RANGE]: The value of "offset" is out of range. It must be >= 0 and <= 8. Received 9 at boundsError (internal/buffer.js:83:9) at checkBounds (internal/buffer.js:52:5) at checkInt (internal/buffer.js:71:3) at writeU_Int16LE (internal/buffer.js:719:3) at Buffer.writeInt16LE (internal/buffer.js:862:10) at Object.<anonymous> (C:\nodejsProject\src\testbuffer.js:2:8) at Module._compile (internal/modules/cjs/loader.js:1063:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:1092:10) at Module.load (internal/modules/cjs/loader.js:928:32) at Function.Module._load (internal/modules/cjs/loader.js:769:14) { code: 'ERR_OUT_OF_RANGE' }
示例
要创建缓冲区,我们将使用 Buffer.allocUnsafe() 方法:
const buffer = Buffer.allocUnsafe(10); buffer.writeInt16LE(0x0910); console.log(buffer);
输出
未使用偏移量,默认情况下将取为 0。因此,上面的输出如下所示:
<Buffer 00 00 11 45 01 00 00 00 00 00>
nodejs_buffer_module.htm
广告