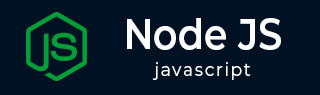
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 查询数据
- Node.js - MySQL 条件查询
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 连接查询
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 数据限制
- Node.js - MongoDB 连接查询
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
NodeJS - console.assert() 方法
Node.js 的 console.assert() 方法会在断言为假时将错误消息打印到控制台,如果断言为真,则什么也不会发生。
在此方法中,如果我们提供 假值 (falsy) 作为参数,它将停止程序执行并告诉我们我们做出的断言是 错误的 (false)。如果我们提供 真值 (truthy) 作为参数,则不会返回或打印任何内容。
为了更好地理解,让我们了解 Node.js 的 console.assert() 方法的语法和用法。
语法
以下是 Node.js console.assert() 方法 的语法:
console.assert(value[,…message]);
参数
此方法接受两个参数,如下所述。
value − 如果给定值为 真值 (truthy),则测试此值。
message − 此参数中传递的任何 消息 或 参数 将被视为错误消息。
返回值
如果我们做出的断言为 真 (true),则此方法不返回任何内容。如果断言失败,则它将抛出错误以及错误消息到 stderr。
以下是 真值 (truthy):
true
{}
[]
30
"0"
"false"
-32
12n
3.14
-3.14
Infinity
-Infinity
示例
在下面的示例中,我们通过将 真值 (truthy) 传递给 value 参数,并将消息传递给该方法的 message 参数来调用 Node.js console.assert() 方法。
const console = require('console'); console.assert(true, 'This asserttion is true');
输出
从下图的输出中可以看到,我们传递的值是 真值 (truthy)。因此,此方法不会返回任何内容。
// Returns nothing
示例
在下面的示例中,我们通过传递不同类型的 真值 (truthy) 值以及消息到该方法的 message 参数来调用 Node.js console.assert() 方法。
const console = require('console'); const emp = []; const num = 30; const inf = Infinity; console.assert(emp, '"${emp}" is a truthy value'); console.assert(num, '"${num}" is a truthy value'); console.assert(inf, '"${inf}" is a truthy value')
输出
如果我们编译并运行上面的代码,我们将得到如下图所示的输出。我们传入的值是 真值 (truthy)。因此,此方法不会返回任何内容。
// Returns nothing
以下是 假值 (falsy):
false
0
-0
0n
"", '', ``
null
undefined
NaN
示例
在下面的示例中,我们通过将 假值 (falsy) 传递给 value 参数,并将错误消息传递给该方法的 message 参数来调用 console.assert() 方法。
const console = require('console'); console.assert(false, 'This is a falsy value');
输出
从下面的输出中可以看到,我们传递的值是 假值 (falsy)。因此,我们做出的断言失败,该方法返回“断言失败”的输出以及其错误消息。
Assertion failed: This is a falsy value
示例
在下面的示例中,我们通过传递不同类型的 假值 (falsy) 值以及错误消息到该方法的 message 参数来调用 console.assert() 方法。
const console = require('console'); const zero = 0; const negzero = -0; const emp = ""; const NULL = null; const und = undefined; const nan = NaN; console.assert(zero, '"${zero}" is a falsy value'); console.assert(negzero, '"${negzero}" is a falsy value'); console.assert(emp, '"${emp}" is a falsy value'); console.assert(NULL, '"${NULL}" is a falsy value'); console.assert(und, '"${und}" is a falsy value'); console.assert(nan, '"${nan}" is a falsy value');
输出
从下面的输出中可以看到,我们做出的断言失败,该方法返回“断言失败”的输出以及其指定的错误消息。
Assertion failed: "${zero}" is a falsy value Assertion failed: "${negzero}" is a falsy value Assertion failed: "${emp}" is a falsy value Assertion failed: "${NULL}" is a falsy value Assertion failed: "${und}" is a falsy value Assertion failed: "${nan}" is a falsy value