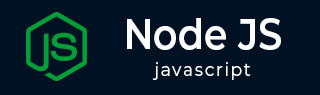
- Node.js 教程
- Node.js - 首页
- Node.js - 简介
- Node.js - 环境搭建
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调函数概念
- Node.js - 上传文件
- Node.js - 发送邮件
- Node.js - 事件
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 进程
- Node.js - 应用程序扩展
- Node.js - 打包
- Node.js - Express 框架
- Node.js - RESTFul API
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 入门
- Node.js - MySQL 创建数据库
- Node.js - MySQL 创建表
- Node.js - MySQL 插入数据
- Node.js - MySQL 从表中选择数据
- Node.js - MySQL Where 条件
- Node.js - MySQL 排序
- Node.js - MySQL 删除数据
- Node.js - MySQL 更新数据
- Node.js - MySQL 连接
- Node.js MongoDB
- Node.js - MongoDB 入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB 插入数据
- Node.js - MongoDB 查找数据
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB 删除数据
- Node.js - MongoDB 更新数据
- Node.js - MongoDB 限制结果数量
- Node.js - MongoDB 连接
- Node.js 模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - 实用程序模块
- Node.js - Web 模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用资源
- Node.js - 讨论
Node.js - os.userInfo() 方法
Node.js 的 os.userInfo() 方法属于 os 模块,它返回一个包含当前有效用户信息的对象,例如 'username'、'uid'、'gid'、'shell' 和 'homedir'。
如果用户没有 username 或 homedir,则会抛出 SystemError。os.userInfo() 方法返回的对象中名为 'homedir' 的属性值由操作系统提供。
以下是返回对象属性的描述。
uid − 用户标识符,用于指定当前有效用户。
gid − 组 ID;它是将用户链接到其他具有某些共同点的用户的标识符。
username − 指定当前有效用户的用户名。
homedir − 指定当前有效用户的 home 目录。
shell − 当前有效用户正在使用的命令行解释器。
语法
以下是 Node.js os.userInfo() 方法 的语法 −
os.userInfo([options])
参数
此方法仅接受一个参数 options,它是可选参数。
encoding 指定返回数据的字符编码。如果 encoding 设置为 'buffer',则 username、shell 和 homedir 的值将是 'Buffer' 的实例。如果 encoding 设置为 'utf8',则值将是 'utf8' 的实例。默认情况下,实例为 'utf8'。
返回值
此方法返回的对象包含名为 'username'、'uid'、'gid'、'shell' 和 'homedir' 的属性。而在 Windows 上,'uid' 和 'gid' 为 −1,'shell' 为 null。
示例
当没有参数传递给方法时,它将采用默认值 'utf8',返回对象属性的值将是 'Buffer' 实例。
在以下示例中,我们尝试通过将 Node.js userInfo() 方法 记录到 控制台 来打印当前有效用户信息。
const os = require('os'); console.log(os.userInfo());
输出
{ uid: 1000, gid: 1000, username: 'webmaster',homedir: '/home/webmaster', shell: '/bin/bash' }
注意 − 为了获得准确的结果,最好在本地执行上述代码。
执行上述程序后,os.userInfo() 方法 返回一个对象,其中包含有关当前有效用户的的信息,如下面的输出所示。
{ uid: -1, gid: -1, username: 'Lenovo', homedir: 'C:\\Users\\Lenovo', shell: null }
示例
如果 os.userInfo() 方法 的 'encoding' 设置为 'utf8',则返回对象属性的值将是 'Buffer' 实例。
在以下示例中,我们尝试将 encoding 值指定为 'utf8'。然后我们打印当前有效用户信息。
const os = require('os'); var option = { encoding: 'utf8' }; console.log(os.userInfo(option));
输出
{ uid: 1000, gid: 1000, username: 'webmaster', homedir: '/home/webmaster', shell: '/bin/bash' }
注意 − 为了获得准确的结果,最好在本地执行上述代码。
执行上述程序后,os.userInfo() 方法 返回的对象打印了 'username'、'uid'、'gid'、'shell' 和 'homedir' 的值作为 'utf8' 实例。
{ uid: -1, gid: -1, username: 'Lenovo', homedir: 'C:\\Users\\Lenovo', shell: null }
示例
在以下示例中,我们尝试将 encoding 值指定为 'buffer'。然后我们通过将 userInfo() 方法记录到控制台来返回当前有效用户信息。
const os = require('os'); var option = { encoding: 'buffer' }; console.log(os.userInfo(option));
输出
{ uid: 1000, gid: 1000, username: <Buffer 77 65 62 6d 61 73 74 65 72>, homedir: <Buffer 2f 68 6f 6d 65 2f 77 65 62 6d 61 73 74 65 72>, shell: <Buffer 2f 62 69 6e 2f 62 61 73 68> }
注意 − 为了获得准确的结果,最好在本地执行上述代码。
执行上述程序后,os.userInfo() 方法 返回的对象打印了 'username'、'uid'、'gid'、'shell' 和 'homedir' 的值作为 'buffer' 实例。
{ uid: -1, gid: -1, username: <Buffer 4c 65 6e 6f 76 6f>, homedir: <Buffer 43 3a 5c 55 73 65 72 73 5c 4c 65 6e 6f 76 6f>, shell: null }