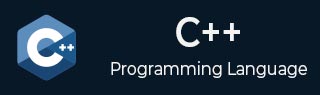
- C++ 基础
- C++ 首页
- C++ 概述
- C++ 环境搭建
- C++ 基本语法
- C++ 注释
- C++ Hello World
- C++ 省略命名空间
- C++ 常量/字面量
- C++ 关键字
- C++ 标识符
- C++ 数据类型
- C++ 数值数据类型
- C++ 字符数据类型
- C++ 布尔数据类型
- C++ 变量类型
- C++ 变量作用域
- C++ 多个变量
- C++ 基本输入/输出
- C++ 修饰符类型
- C++ 存储类
- C++ 运算符
- C++ 数字
- C++ 枚举
- C++ 引用
- C++ 日期和时间
- C++ 控制语句
- C++ 决策
- C++ if 语句
- C++ if else 语句
- C++ 嵌套 if 语句
- C++ switch 语句
- C++ 嵌套 switch 语句
- C++ 循环类型
- C++ while 循环
- C++ for 循环
- C++ do while 循环
- C++ foreach 循环
- C++ 嵌套循环
- C++ break 语句
- C++ continue 语句
- C++ goto 语句
- C++ 构造函数
- C++ 构造函数和析构函数
- C++ 复制构造函数
C++ 运算符
运算符是告诉编译器执行特定数学或逻辑操作的符号。C++ 拥有丰富的内置运算符,并提供以下类型的运算符:
- 算术运算符
- 关系运算符
- 逻辑运算符
- 位运算符
- 赋值运算符
- 其他运算符
本章将逐一介绍算术、关系、逻辑、位、赋值和其他运算符。
算术运算符
C++ 中的算术运算符 是基本的运算符,用于对操作数执行基本的数学或算术运算。这些运算符对于执行计算和在程序中操作数据至关重要。
C++ 语言支持以下算术运算符:
假设变量 A 持有 10,变量 B 持有 20,则:
运算符 | 描述 | 示例 |
---|---|---|
+ | 加两个操作数 | A + B 将得到 30 |
- | 从第一个操作数中减去第二个操作数 | A - B 将得到 -10 |
* | 将两个操作数相乘 | A * B 将得到 200 |
/ | 将分子除以分母 | B / A 将得到 2 |
% | 模运算符,整数除法后的余数 | B % A 将得到 0 |
++ | 递增运算符,将整数值增加 1 | A++ 将得到 11 |
-- | 递减运算符,将整数值减少 1 | A-- 将得到 9 |
示例
以下是算术运算符的示例:
#include <iostream> using namespace std; main() { int a = 21; int b = 10; int c ; c = a + b; cout << "Line 1 - Value of c is :" << c << endl ; c = a - b; cout << "Line 2 - Value of c is :" << c << endl; c = a * b; cout << "Line 3 - Value of c is :" << c << endl ; c = a / b; cout << "Line 4 - Value of c is :" << c << endl ; c = a % b; cout << "Line 5 - Value of c is :" << c << endl ; c = a++; cout << "Line 6 - Value of c is :" << c << endl ; c = a--; cout << "Line 7 - Value of c is :" << c << endl ; return 0; }
输出
Line 1 - Value of c is :31 Line 2 - Value of c is :11 Line 3 - Value of c is :210 Line 4 - Value of c is :2 Line 5 - Value of c is :1 Line 6 - Value of c is :21 Line 7 - Value of c is :22
关系运算符
关系运算符 用于比较两个值或表达式。这些运算符返回布尔值 - 如果比较正确则为 **true**,否则为 **false**。
它们对于根据条件做出决策和控制程序流程至关重要。
C++ 语言支持以下关系运算符
假设变量 A 持有 10,变量 B 持有 20,则:
运算符 | 描述 | 示例 |
---|---|---|
== | 检查两个操作数的值是否相等,如果相等则条件为真。 | (A == B) 为假。 |
!= | 检查两个操作数的值是否不相等,如果不相等则条件为真。 | (A != B) 为真。 |
> | 检查左侧操作数的值是否大于右侧操作数的值,如果是则条件为真。 | (A > B) 为假。 |
< | 检查左侧操作数的值是否小于右侧操作数的值,如果是则条件为真。 | (A < B) 为真。 |
>= | 检查左侧操作数的值是否大于或等于右侧操作数的值,如果是则条件为真。 | (A >= B) 为假。 |
<= | 检查左侧操作数的值是否小于或等于右侧操作数的值,如果是则条件为真。 | (A <= B) 为真。 |
示例
以下是关系运算符的示例:
#include <iostream> using namespace std; main() { int a = 21; int b = 10; int c ; if( a == b ) { cout << "Line 1 - a is equal to b" << endl ; } else { cout << "Line 1 - a is not equal to b" << endl ; } if( a < b ) { cout << "Line 2 - a is less than b" << endl ; } else { cout << "Line 2 - a is not less than b" << endl ; } if( a > b ) { cout << "Line 3 - a is greater than b" << endl ; } else { cout << "Line 3 - a is not greater than b" << endl ; } /* Let's change the values of a and b */ a = 5; b = 20; if( a <= b ) { cout << "Line 4 - a is either less than \ or equal to b" << endl ; } if( b >= a ) { cout << "Line 5 - b is either greater than \ or equal to b" << endl ; } return 0; }
输出
Line 1 - a is not equal to b Line 2 - a is not less than b Line 3 - a is greater than b Line 4 - a is either less than or equal to b Line 5 - b is either greater than or equal to b
逻辑运算符
逻辑运算符 用于对布尔值(**true** 或 **false**)执行逻辑运算。这些运算符对于根据条件控制程序流程至关重要。C++ 中有三个主要的逻辑运算符,如下所示:
C++ 语言支持以下逻辑运算符。
假设变量 A 持有 1,变量 B 持有 0,则:
运算符 | 描述 | 示例 |
---|---|---|
&& | 称为逻辑与运算符。如果两个操作数均非零,则条件为真。 | (A && B) 为假。 |
|| | 称为逻辑或运算符。如果两个操作数中任何一个非零,则条件为真。 | (A || B) 为真。 |
! | 称为逻辑非运算符。用于反转其操作数的逻辑状态。如果条件为真,则逻辑非运算符将使其为假。 | !(A && B) 为真。 |
示例
以下是逻辑运算符的示例:
#include <iostream> using namespace std; main() { int a = 5; int b = 20; int c ; if(a && b) { cout << "Line 1 - Condition is true"<< endl ; } if(a || b) { cout << "Line 2 - Condition is true"<< endl ; } /* Let's change the values of a and b */ a = 0; b = 10; if(a && b) { cout << "Line 3 - Condition is true"<< endl ; } else { cout << "Line 4 - Condition is not true"<< endl ; } if(!(a && b)) { cout << "Line 5 - Condition is true"<< endl ; } return 0; }
输出
Line 1 - Condition is true Line 2 - Condition is true Line 4 - Condition is not true Line 5 - Condition is true
位运算符
位运算符 用于对整数数据类型执行位级运算。这些运算对位进行直接操作,例如低级编程、图形和密码学。
位运算符在位上工作并执行逐位运算。&、| 和 ^ 的真值表如下所示:
p | q | p & q | p | q | p ^ q |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
1 | 0 | 0 | 1 | 1 |
假设 A = 60;B = 13;现在以二进制格式,它们将如下所示:
A = 0011 1100
B = 0000 1101
-----------------
A&B = 0000 1100
A|B = 0011 1101
A^B = 0011 0001
~A = 1100 0011
C++ 语言支持的位运算符列在下表中。假设变量 A 持有 60,变量 B 持有 13,则:
运算符 | 描述 | 示例 |
---|---|---|
& | 二进制与运算符将位复制到结果中,如果它存在于两个操作数中。 | (A & B) 将得到 12,即 0000 1100 |
| | 二进制或运算符将位复制,如果它存在于任何一个操作数中。 | (A | B) 将得到 61,即 0011 1101 |
^ | 二进制异或运算符将位复制,如果它在一个操作数中设置,而不是在两个操作数中都设置。 | (A ^ B) 将得到 49,即 0011 0001 |
~ | 二进制非运算符是一元运算符,其作用是“翻转”位。 | (~A ) 将得到 -61,由于带符号二进制数,在 2 的补码形式中为 1100 0011。 |
<< | 二进制左移运算符。左侧操作数的值向左移动右侧操作数指定的位数。 | A << 2 将得到 240,即 1111 0000 |
>> | 二进制右移运算符。左侧操作数的值向右移动右侧操作数指定的位数。 | A >> 2 将得到 15,即 0000 1111 |
示例
以下是位运算符的示例:
#include <iostream> using namespace std; main() { unsigned int a = 60; // 60 = 0011 1100 unsigned int b = 13; // 13 = 0000 1101 int c = 0; c = a & b; // 12 = 0000 1100 cout << "Line 1 - Value of c is : " << c << endl ; c = a | b; // 61 = 0011 1101 cout << "Line 2 - Value of c is: " << c << endl ; c = a ^ b; // 49 = 0011 0001 cout << "Line 3 - Value of c is: " << c << endl ; c = ~a; // -61 = 1100 0011 cout << "Line 4 - Value of c is: " << c << endl ; c = a << 2; // 240 = 1111 0000 cout << "Line 5 - Value of c is: " << c << endl ; c = a >> 2; // 15 = 0000 1111 cout << "Line 6 - Value of c is: " << c << endl ; return 0; }
输出
Line 1 - Value of c is : 12 Line 2 - Value of c is: 61 Line 3 - Value of c is: 49 Line 4 - Value of c is: -61 Line 5 - Value of c is: 240 Line 6 - Value of c is: 15
赋值运算符
赋值运算符 用于为变量赋值。这些运算符允许您设置或更新存储在变量中的值。
C++ 语言支持以下赋值运算符:
运算符 | 描述 | 示例 |
---|---|---|
= | 简单赋值运算符,将右侧操作数的值赋给左侧操作数。 | C = A + B 将 A + B 的值赋给 C |
+= | 加法赋值运算符,它将右侧操作数加到左侧操作数上,并将结果赋给左侧操作数。 | C += A 等价于 C = C + A |
-= | 减法赋值运算符,它从左侧操作数中减去右侧操作数,并将结果赋给左侧操作数。 | C -= A 等价于 C = C - A |
*= | 乘法赋值运算符,它将右侧操作数乘以左侧操作数,并将结果赋给左侧操作数。 | C *= A 等价于 C = C * A |
/= | 除法赋值运算符,它将左侧操作数除以右侧操作数,并将结果赋给左侧操作数。 | C /= A 等价于 C = C / A |
%= | 模赋值运算符,它使用两个操作数取模,并将结果赋给左侧操作数。 | C %= A 等价于 C = C % A |
<<= | 左移赋值运算符。 | C <<= 2 等价于 C = C << 2 |
>>= | 右移赋值运算符。 | C >>= 2 等价于 C = C >> 2 |
&= | 按位与赋值运算符。 | C &= 2 等价于 C = C & 2 |
^= | 按位异或赋值运算符。 | C ^= 2 等价于 C = C ^ 2 |
|= | 按位或赋值运算符。 | C |= 2 等价于 C = C | 2 |
示例
下面是一个赋值运算符的示例:
#include <iostream> using namespace std; main() { int a = 21; int c ; c = a; cout << "Line 1 - = Operator, Value of c = : " <<c<< endl ; c += a; cout << "Line 2 - += Operator, Value of c = : " <<c<< endl ; c -= a; cout << "Line 3 - -= Operator, Value of c = : " <<c<< endl ; c *= a; cout << "Line 4 - *= Operator, Value of c = : " <<c<< endl ; c /= a; cout << "Line 5 - /= Operator, Value of c = : " <<c<< endl ; c = 200; c %= a; cout << "Line 6 - %= Operator, Value of c = : " <<c<< endl ; c <<= 2; cout << "Line 7 - <<= Operator, Value of c = : " <<c<< endl ; c >>= 2; cout << "Line 8 - >>= Operator, Value of c = : " <<c<< endl ; c &= 2; cout << "Line 9 - &= Operator, Value of c = : " <<c<< endl ; c ^= 2; cout << "Line 10 - ^= Operator, Value of c = : " <<c<< endl ; c |= 2; cout << "Line 11 - |= Operator, Value of c = : " <<c<< endl ; return 0; }
输出
Line 1 - = Operator, Value of c = : 21 Line 2 - += Operator, Value of c = : 42 Line 3 - -= Operator, Value of c = : 21 Line 4 - *= Operator, Value of c = : 441 Line 5 - /= Operator, Value of c = : 21 Line 6 - %= Operator, Value of c = : 11 Line 7 - <<= Operator, Value of c = : 44 Line 8 - >>= Operator, Value of c = : 11 Line 9 - &= Operator, Value of c = : 2 Line 10 - ^= Operator, Value of c = : 0 Line 11 - |= Operator, Value of c = : 2
其他运算符
杂项运算符(通常缩写为“misc operators”)包括各种不适合归入算术运算符或逻辑运算符等其他类别的运算符。以下是几种常见的杂项运算符及其定义:
下表列出了 C++ 支持的其他一些运算符。
序号 | 运算符 & 描述 |
---|---|
1 | sizeof sizeof 运算符 返回变量的大小。例如,sizeof(a),其中 'a' 为整数,将返回 4。 |
2 | Condition ? X : Y 条件运算符 (?)。如果 Condition 为真,则返回 X 的值,否则返回 Y 的值。 |
3 | , 逗号运算符 用于执行一系列操作。整个逗号表达式的值是逗号分隔列表中最后一个表达式的值。 |
4 | .(点)和 ->(箭头) 成员运算符 用于引用类、结构体和联合体的各个成员。 |
5 | 强制转换 强制转换运算符 将一种数据类型转换为另一种数据类型。例如,int(2.2000) 将返回 2。 |
6 | & 指针运算符 & 返回变量的地址。例如 &a; 将给出变量的实际地址。 |
7 | * 指针运算符 * 是指向变量的指针。例如 *var; 将指向变量 var。 |
条件(三元)运算符 (?:)
它是 C++ 中执行条件评估的一种简写方式。
int a = 10, b = 20; int result = (a > b) ? a : b; // result will be 20 because a is not greater than b cout << "The greater value is: " << result << endl;
输出
The greater value is: 20
sizeof 运算符
sizeof 运算符返回变量或数据类型的大小(以字节为单位)。
int x = 5; cout << "Size of int: " << sizeof(x) << " bytes" << endl; cout << "Size of double: " << sizeof(double) << " bytes" << endl;
输出
4 8
按位取反运算符 (~)
按位取反运算符反转其操作数的位。
unsigned int num = 5; // Binary: 00000000 00000000 00000000 00000101 unsigned int result = ~num; // Binary: 11111111 11111111 11111111 11111010 cout << "Bitwise complement of 5: " << result << endl; // Outputs a large positive number due to unsigned
范围解析运算符 (::)
此运算符用于定义函数或变量的范围,尤其是在类和命名空间的上下文中。
class MyClass { public: static int value; }; int MyClass::value = 10; // Define static member outside the class cout << "Static value: " << MyClass::value << endl;
输出
10
C++ 中的运算符优先级
运算符优先级 决定了表达式中项的组合方式。这会影响表达式的计算方式。某些运算符的优先级高于其他运算符;例如,乘法运算符的优先级高于加法运算符:
例如 x = 7 + 3 * 2; 在这里,x 被赋值为 13,而不是 20,因为运算符 * 的优先级高于 +,所以它首先与 3*2 相乘,然后加到 7 中。
这里,优先级最高的运算符出现在表格顶部,优先级最低的出现在底部。在表达式中,优先级较高的运算符将首先被计算。
类别 | 运算符 | 结合性 |
---|---|---|
后缀 | () [] -> . ++ -- | 从左到右 |
一元 | + - ! ~ ++ -- (type)* & sizeof | 从右到左 |
乘法 | * / % | 从左到右 |
加法 | + - | 从左到右 |
移位 | << >> | 从左到右 |
关系 | < <= > >= | 从左到右 |
相等 | == != | 从左到右 |
按位与 | & | 从左到右 |
按位异或 | ^ | 从左到右 |
按位或 | | | 从左到右 |
逻辑与 | && | 从左到右 |
逻辑或 | || | 从左到右 |
条件 | ?: | 从右到左 |
赋值 | = += -= *= /= %= >>= <<= &= ^= |= | 从右到左 |
逗号 | , | 从左到右 |
示例
下面是一个演示运算符优先级的示例:
#include <iostream> using namespace std; main() { int a = 20; int b = 10; int c = 15; int d = 5; int e; e = (a + b) * c / d; // ( 30 * 15 ) / 5 cout << "Value of (a + b) * c / d is :" << e << endl ; e = ((a + b) * c) / d; // (30 * 15 ) / 5 cout << "Value of ((a + b) * c) / d is :" << e << endl ; e = (a + b) * (c / d); // (30) * (15/5) cout << "Value of (a + b) * (c / d) is :" << e << endl ; e = a + (b * c) / d; // 20 + (150/5) cout << "Value of a + (b * c) / d is :" << e << endl ; return 0; }
输出
Value of (a + b) * c / d is :90 Value of ((a + b) * c) / d is :90 Value of (a + b) * (c / d) is :90 Value of a + (b * c) / d is :50