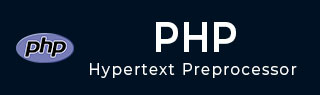
- PHP 教程
- PHP - 首页
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔法常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混合
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc 和 Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期和时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 比较符
- PHP 控制语句
- PHP - 决策制定
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - Final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET 和 POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 过滤输入
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - Bug 调试
- PHP - 针对 C 开发人员
- PHP - 针对 PERL 开发人员
- PHP - 框架
- PHP - Core PHP 与框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望
- PHP - Use 语句
- PHP - 整数除法
- PHP - 已弃用的特性
- PHP - 已移除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP – 抽象类
PHP 的保留字列表包括“abstract”关键字。当一个类用“abstract”关键字定义时,它不能被实例化,即你不能声明此类的新对象。抽象类可以被另一个类扩展。
abstract class myclass { // class body }
如上所述,你**不能声明此类的对象**。因此,以下语句 -
$obj = new myclass;
将导致如下所示的**错误**消息 -
PHP Fatal error: Uncaught Error: Cannot instantiate abstract class myclass
抽象类可能包含属性、常量或方法。类成员可以是公有、私有或受保护类型。类中的一种或多种方法也可以定义为抽象方法。
如果类中的任何方法是抽象的,那么该类本身必须是抽象类。换句话说,普通类不能在其内部定义抽象方法。
这将引发**错误** -
class myclass { abstract function myabsmethod($arg1, $arg2); function mymethod() #this is a normal method { echo "Hello"; } }
**错误消息**将显示为 -
PHP Fatal error: Class myclass contains 1 abstract method and must therefore be declared abstract
你可以使用抽象类作为父类并用子类扩展它。但是,子类必须为父类中的每个抽象方法提供具体的实现,否则将遇到错误。
示例
在以下代码中,**myclass** 是一个**抽象类**,**myabsmethod()** 是一个**抽象方法**。它的派生类是**mynewclass**,但它没有在其父类中实现抽象方法。
<?php abstract class myclass { abstract function myabsmethod($arg1, $arg2); function mymethod() { echo "Hello"; } } class newclass extends myclass { function newmethod() { echo "World"; } } $m1 = new newclass; $m1->mymethod(); ?>
在这种情况下,**错误消息**为 -
PHP Fatal error: Class newclass contains 1 abstract method and must therefore be declared abstract or implement the remaining methods (myclass::myabsmethod)
它表明 newclass 应该实现抽象方法,或者它应该被声明为抽象类。
示例
在以下 PHP 脚本中,我们有**marks** 作为抽象类,其中 percent() 是一个抽象方法。另一个**student** 类扩展了**marks** 类并实现了它的 percent() 方法。
<?php abstract class marks { protected int $m1, $m2, $m3; abstract public function percent(): float; } class student extends marks { public function __construct($x, $y, $z) { $this->m1 = $x; $this->m2 = $y; $this->m3 = $z; } public function percent(): float { return ($this->m1+$this->m2+$this->m3)*100/300; } } $s1 = new student(50, 60, 70); echo "Percentage of marks: ". $s1->percent() . PHP_EOL; ?>
它将产生以下**输出** -
Percentage of marks: 60
PHP 中接口和抽象类的区别
PHP 中抽象类的概念与接口非常相似。但是,接口和抽象类之间存在一些区别。
抽象类 |
接口 |
---|---|
使用 abstract 关键字定义抽象类 |
使用 interface 关键字定义接口 |
抽象类不能被实例化 |
接口不能被实例化。 |
抽象类可以具有普通方法和抽象方法 |
接口必须仅声明带参数和返回类型的方法,而不能带任何主体。 |
抽象类被子类扩展,子类必须实现所有抽象方法 |
接口必须由另一个类实现,该类必须提供接口中所有方法的功能。 |
可以具有公有、私有或受保护的属性 |
接口中不能声明属性 |