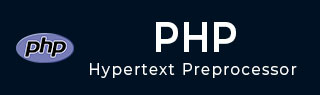
- PHP 教程
- PHP - 首页
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混淆
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc 和 Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期与时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 恒等比较运算符
- PHP 控制语句
- PHP - 决策制定
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象的 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - Final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET 和 POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 净化输入
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - Bug 调试
- PHP - 针对 C 开发人员
- PHP - 针对 PERL 开发人员
- PHP - 框架
- PHP - Core PHP 与框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望
- PHP - Use 语句
- PHP - 整数除法
- PHP - 已弃用的特性
- PHP - 已删除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
PHP - AJAX 自动完成搜索
自动完成功能是一种输入提示机制,当用户在提供的搜索框中输入数据时,它会显示输入建议。它也称为实时搜索,因为它会对用户的输入做出反应。在本例中,我们将使用 PHP 中的 AJAX 和 XML 解析器来演示自动完成文本框的使用。
此应用程序具有三个主要组成部分:
XML 文档
JavaScript 代码
PHP 中的 XML 解析器
现在让我们详细讨论这三个组成部分:
XML 文档
将以下 XML 脚本保存为“autocomplete.xml”到文档根目录文件夹
<?xml version = "1.0" encoding = "utf-8"?> <pages> <link> <title>android</title> <url>https://tutorialspoint.com/android/index.htm</url> </link> <link> <title>Java</title> <url>https://tutorialspoint.com/java/index.htm</url> </link> <link> <title>CSS </title> <url>https://tutorialspoint.com/css/index.htm</url> </link> <link> <title>angularjs</title> <url>https://tutorialspoint.com/angularjs/index.htm </url> </link> <link> <title>hadoop</title> <url>https://tutorialspoint.com/hadoop/index.htm </url> </link> <link> <title>swift</title> <url>https://tutorialspoint.com/swift/index.htm </url> </link> <link> <title>ruby</title> <url>https://tutorialspoint.com/ruby/index.htm </url> </link> <link> <title>nodejs</title> <url>https://tutorialspoint.com/nodejs/index.htm </url> </link> </pages>
JavaScript 代码
以下脚本呈现一个文本字段,供用户输入他选择的课程名称。每次按键都会调用一个 JavaScript 函数,并将输入值通过 GET 方法传递到服务器端的 PHP 脚本。服务器的响应会异步渲染。
将此代码保存为“index.php”。
<html> <head> <script> function showResult(str) { if (str.length == 0) { document.getElementById("livesearch").innerHTML = ""; document.getElementById("livesearch").style.border = "0px"; return; } if (window.XMLHttpRequest) { xmlhttp = new XMLHttpRequest(); } else { xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.onreadystatechange = function() { if (xmlhttp.readyState == 4 && xmlhttp.status == 200) { document.getElementById("livesearch").innerHTML = xmlhttp.responseText; document.getElementById("livesearch").style.border = "1px solid #A5ACB2"; } } xmlhttp.open("GET","livesearch.php?q="+str,true); xmlhttp.send(); } </script> </head> <body> <form> <h2>Enter Course Name</h2> <input type = "text" size = "30" onkeyup = "showResult(this.value)"> <div id = "livesearch"></div> <a href = "https://tutorialspoint.com">More Details</a> </form> </body> </html>
PHP 中的 XML 解析器
这是服务器上的 PHP 脚本。它解析给定的 XML 源文档,读取输入字段中输入的字符,在解析的 XNL 对象中搜索它,并发送回响应。
将以下代码保存为“livesearch.php”。
<?php $xml_doc = new DOMDocument(); $xml_doc->load('autocomplete.xml'); $x=$xml_doc->getElementsByTagName('link'); $q = $_GET['q']; $result = ''; foreach($x as $node) { if (stripos("{$node->nodeValue}", $q) !== false) { $result .= "{$node->nodeValue}"; } } // Set $response to "No records found." in case no hint was found // or the values of the matching values if ($result == '') $result = 'No records found.'; // show the results or "No records found." echo $result; ?>
在 XAMPP 服务器运行的情况下,访问“https://127.0.0.1/index.php”,浏览器会显示一个输入文本字段。对于在其中键入的每个字符,相关的建议都会显示在其下方。
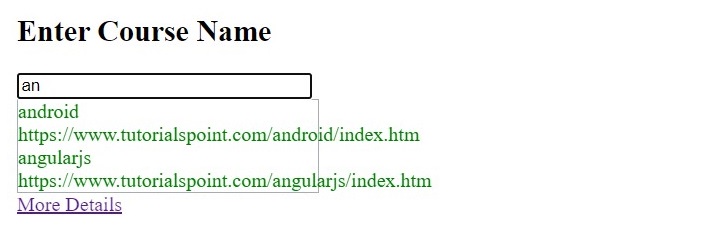
广告