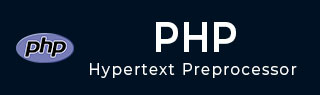
- PHP 教程
- PHP - 首页
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混合
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc & Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期与时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 键值比较运算符
- PHP 控制语句
- PHP - 决策
- PHP - If...Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do...While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象 PHP
- PHP - 面向对象编程
- PHP - 类与对象
- PHP - 构造函数与析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP – 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - Final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET & POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 净化输入
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try...Catch
- PHP - Bug 调试
- PHP - 针对 C 开发人员
- PHP - 针对 PERL 开发人员
- PHP - 框架
- PHP - Core PHP 与框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望
- PHP - Use 语句
- PHP - 整数除法
- PHP - 已弃用的特性
- PHP - 已删除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP – 特性
在 PHP 中,一个类只能继承自一个父类,PHP 中未定义多重继承。PHP 中的特性是为了克服此限制而引入的。您可以在特性中定义一个或多个方法,这些方法可以在各种独立类中自由重用。
语法
“trait”关键字按以下语法使用:
trait mytrait { function method1() { /*function body*/ } function method2() { /*function body*/ } }
为了能够调用特性中的方法,需要使用 use 关键字将其提供给另一个类。
示例
特性类似于类,但仅用于以细粒度和一致的方式对功能进行分组。无法单独实例化特性。
<?php trait mytrait { public function hello() { echo "Hello World from " . __TRAIT__ . ""; } } class myclass { use mytrait; } $obj = new myclass(); $obj->hello(); ?>
它将产生以下输出:
Hello World from mytrait
示例
特性可以在多个类中使用。以下示例具有一个包含 avg() 函数的 mytrait。它用于 marks 类中。percent() 方法在内部调用特性中的 avg() 函数。
请查看以下示例:
<?php trait mytrait { function avg($x, $y) { return ($x+$y)/2; } } class marks { use mytrait; private int $m1, $m2; function __construct($x, $y) { $this->m1 = $x; $this->m2 = $y; } function percent():float { return $this->avg($this->m1, $this->m2); } } $obj = new marks(50, 60); echo "percentage: " . $obj->percent(); ?>
它将产生以下输出:
percentage: 55
使用多个特性
一个类可以使用多个特性。这里我们有两个特性,每个特性都有一个函数,分别执行两个数字的加法和乘法。两者都用于第三个类中。
<?php trait addition { function add($x, $y) { return $x+$y; } } trait multiplication { function multiply($x, $y) { return $x*$y; } } class numbers { use addition, multiplication; private int $m1, $m2; function __construct($x, $y) { $this->m1 = $x; $this->m2 = $y; } function calculate():array { $arr = [$this->add($this->m1, $this->m2), $this->multiply($this->m1, $this->m2)]; return $arr; } } $obj = new numbers(50, 60); $res = $obj->calculate(); echo "Addition: " . $res[0] . PHP_EOL; echo "Multiplication: " . $res[1] . PHP_EOL; ?>
它将产生以下输出:
Addition: 110 Multiplication: 3000
覆盖特性函数
当一个类使用某个特性时,它的函数就像子类继承父方法一样对它可用。也可以覆盖特性函数。
<?php trait mytrait { public function sayHello() { echo 'Hello World!'; } } class myclass { use mytrait; public function sayHello() { echo 'Hello PHP!'; } } $o = new myclass(); $o->sayHello(); ?>
它将产生以下输出:
Hello PHP!
“insteadof”关键字
有时,两个或更多特性可能具有相同名称的函数。因此,在类中使用它们会产生模棱两可的情况。PHP 提供 insteadof 关键字来告诉解析器您打算使用哪个特性的函数。
<?php trait mytrait { public function sayHello() { echo 'Hello World!'; } } trait newtrait { public function sayHello() { echo 'Hello PHP!'; } } class myclass { use mytrait, newtrait{ newtrait::sayHello insteadof mytrait; } } $o = new myclass(); $o->sayHello(); ?>
它将产生以下输出:
Hello PHP!
特性函数的别名
如果希望能够调用来自两个特性的函数,即使它们具有相同名称的函数,也可以通过为其中一个指定别名作为解决方法。
示例
在以下示例中,我们将 mytrait 中的 sayHello() 称为 hello():
<?php trait mytrait { public function sayHello() { echo 'Hello World!' . PHP_EOL; } } trait newtrait { public function sayHello() { echo 'Hello PHP!' . PHP_EOL; } } class myclass { use mytrait, newtrait{ mytrait::sayHello as hello; newtrait::sayHello insteadof mytrait; } } $o = new myclass(); $o->hello(); $o->sayHello(); ?>
它将产生以下输出:
Hello World! Hello PHP!
广告