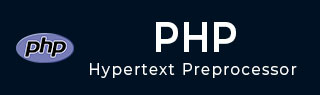
- PHP 教程
- PHP - 首页
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混合
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc 和 Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期和时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 比较符
- PHP 控制语句
- PHP - 决策
- PHP - If...Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do...While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - Final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET 和 POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 过滤输入
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try...Catch
- PHP - Bug 调试
- PHP - 适用于 C 开发人员
- PHP - 适用于 Perl 开发人员
- PHP - 框架
- PHP - Core PHP 与框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望
- PHP - Use 语句
- PHP - 整数除法
- PHP - 已弃用的功能
- PHP - 已移除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
PHP - 完整表单
本章将表单验证和提取 HTML 表单数据到 PHP 代码中的所有概念都整合在一起。下面给出的完整表单处理代码分为三个部分:开头是一个 PHP 代码部分,用于在提交表单时查找任何验证错误,HTML 表单包含各种元素,如文本字段、单选按钮、选择控件、复选框等。第三部分又是 PHP 代码,用于呈现用户输入的数据。
PHP 错误跟踪
捕获错误的代码位于整个脚本的开头。显然,每次加载页面时都会执行此代码。如果在提交表单后加载页面,则以下部分检查每个元素是否为空、电子邮件字段格式是否正确以及是否选中了复选框(表示用户同意条款)。
<?php // define variables and set to empty values $nameErr = $emailErr = $genderErr = $websiteErr = ""; $name = $email = $gender = $class = $course = $subject = ""; if ($_SERVER["REQUEST_METHOD"] == "POST") { if (empty($_POST["name"])) { $nameErr = "Name is required"; } else { $name = test_input($_POST["name"]); } if (empty($_POST["email"])) { $emailErr = "Email is required"; } else { $email = test_input($_POST["email"]); // check if e-mail address is well-formed if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { $emailErr = "Invalid email format"; } } if (empty($_POST["course"])) { $course = ""; } else { $course = test_input($_POST["course"]); } if (empty($_POST["class"])) { $class = ""; } else { $class = test_input($_POST["class"]); } if (empty($_POST["gender"])) { $genderErr = "Gender is required"; } else { $gender = test_input($_POST["gender"]); } if (empty($_POST["subject"])) { $subjectErr = "You must select one or more subjects"; } else { $subject = $_POST["subject"]; } } function test_input($data) { $data = trim($data); $data = stripslashes($data); $data = htmlspecialchars($data); return $data; } ?>
HTML 表单
呈现输入表单的 HTML 脚本位于错误捕获代码之后。表单设计中使用了各种表单元素。
<h2>Absolute Classes Registration Form</h2> <p><span class = "error">* required field.</span></p> <form method = "POST" action = "<?php echo htmlspecialchars($_SERVER["PHP_SELF"]);?>"> <table> <tr> <td>Name:</td> <td> <input type = "text" name = "name"> <span class = "error">* <?php echo $nameErr;?></span> </td> </tr> <tr> <td>E-mail: </td> <td> <input type = "text" name = "email"> <span class = "error">* <?php echo $emailErr;?></span> </td> </tr> <tr> <td>Time:</td> <td> <input type = "text" name = "course"> <span class = "error"><?php echo $websiteErr;?></span> </td> </tr> <tr> <td>Classes:</td> <td><textarea name = "class" rows = "5" cols = "40"></textarea></td> </tr> <tr> <td>Gender:</td> <td> <input type = "radio" name = "gender" value = "female">Female <input type = "radio" name = "gender" value = "male">Male <span class = "error">* <?php echo $genderErr;?></span> </td> </tr> <tr> <td>Select:</td> <td> <select name = "subject[]" size = "4" multiple> <option value = "Android">C</option> <option value = "Java">Java</option> <option value = "C#">C#</option> <option value = "Data Base">C++</option> <option value = "Hadoop">PHP</option> <option value = "VB script">Python</option> </select> </td> </tr> <tr> <td>Agree</td> <td><input type = "checkbox" name = "checked" value = "1"></td> <?php if(!isset($_POST['checked'])){ ?> <span class = "error">* <?php echo "You must agree to terms";?></span> <?php } ?> </tr> <tr> <td> <input type = "submit" name = "submit" value = "Submit"> </td> </tr> </table> </form>
请注意,表单数据会提交回同一个脚本,因此表单的 action 属性设置为 $_SERVER["PHP_SELF"] 超全局变量。此部分还包含某些内联 PHP 代码,这些代码会在相应的表单控件旁边显示错误消息,例如,如果在提交表单时名称字段为空,则在名称文本框旁边显示“名称必填”消息。
显示表单数据
脚本的第三部分又是 PHP 代码,用于回显用户提交的每个表单字段的值。
<?php if ($_SERVER["REQUEST_METHOD"] == "POST") { echo "<h2>Your given values are as :</h2>"; echo ("<p><b>Name</b> : $name</p>"); echo ("<p><b>Email address</b> : $email</p>"); echo ("<p><b>Preffered class time</b> : $course</p>"); echo ("<p><b>Class info</b> : $class </p>"); echo ("<p><b>Gender</b> : $gender</p>"); echo "<p><b>Subjcts Chosen:</b><p>"; if (!empty($subject)) { echo "<ul>"; for($i = 0; $i < count($subject); $i++) { echo "<li>$subject[$i]</u/li>"; } echo "</ul>"; } } ?>
以下是当从服务器的文档根文件夹运行脚本时,在表单中填写的一些示例数据:
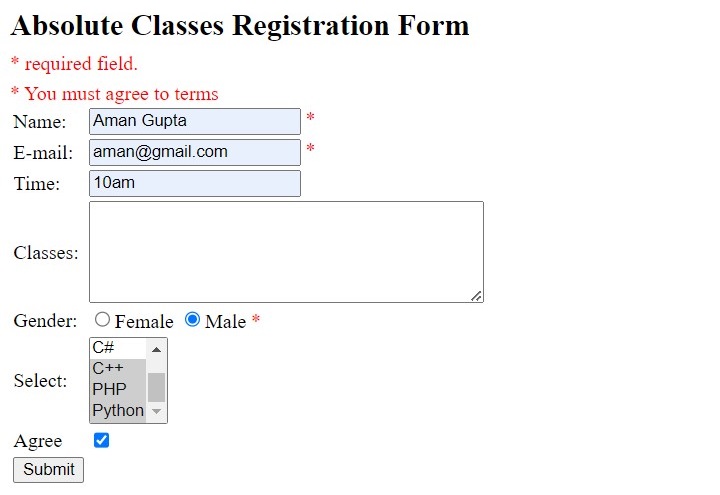
提交后,输出如下所示:
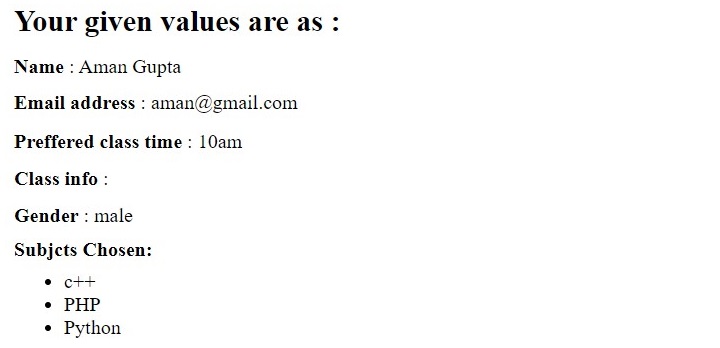
示例
PHP 处理 HTML 表单的完整代码如下:
<html> <head> <style> .error {color: #FF0000;} </style> </head> <body> <?php // define variables and set to empty values $nameErr = $emailErr = $genderErr = $websiteErr = ""; $name = $email = $gender = $class = $course = $subject = ""; if ($_SERVER["REQUEST_METHOD"] == "POST") { if (empty($_POST["name"])) { $nameErr = "Name is required"; }else { $name = test_input($_POST["name"]); } if (empty($_POST["email"])) { $emailErr = "Email is required"; } else { $email = test_input($_POST["email"]); // check if e-mail address is well-formed if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { $emailErr = "Invalid email format"; } } if (empty($_POST["course"])) { $course = ""; } else { $course = test_input($_POST["course"]); } if (empty($_POST["class"])) { $class = ""; } else { $class = test_input($_POST["class"]); } if (empty($_POST["gender"])) { $genderErr = "Gender is required"; } else { $gender = test_input($_POST["gender"]); } if (empty($_POST["subject"])) { $subjectErr = "You must select one or more subjects"; } else { $subject = $_POST["subject"]; } } function test_input($data) { $data = trim($data); $data = stripslashes($data); $data = htmlspecialchars($data); return $data; } ?> <h2>Absolute Classes Registration Form</h2> <p><span class = "error">* required field.</span></p> <form method = "POST" action = "<?php echo htmlspecialchars($_SERVER["PHP_SELF"]);?>"> <table> <tr> <td>Name:</td> <td> <input type = "text" name = "name"> <span class = "error">* <?php echo $nameErr;?></span> </td> </tr> <tr> <td>E-mail: </td> <td> <input type = "text" name = "email"> <span class = "error">* <?php echo $emailErr;?></span> </td> </tr> <tr> <td>Time:</td> <td> <input type = "text" name = "course"> <span class = "error"><?php echo $websiteErr;?></span> </td> </tr> <tr> <td>Classes:</td> <td><textarea name = "class" rows = "5" cols = "40"></textarea></td> </tr> <tr> <td>Gender:</td> <td> <input type = "radio" name = "gender" value = "female">Female <input type = "radio" name = "gender" value = "male">Male <span class = "error">* <?php echo $genderErr;?></span> </td> </tr> <tr> <td>Select:</td> <td> <select name = "subject[]" size = "4" multiple> <option value = "C">C</option> <option value = "Java">Java</option> <option value = "C#">C#</option> <option value = "c++">C++</option> <option value = "PHP">PHP</option> <option value = "Python">Python</option> </select> </td> </tr> <tr> <td>Agree</td> <td><input type = "checkbox" name = "checked" value = "1"></td> <?php if(!isset($_POST['checked'])){ ?> <span class = "error">* <?php echo "You must agree to terms";?></span> <?php } ?> </tr> <tr> <td> <input type = "submit" name = "submit" value = "Submit"> </td> </tr> </table> </form> <?php if ($_SERVER["REQUEST_METHOD"] == "POST") { echo "<h2>Your given values are as :</h2>"; echo ("<p><b>Name</b> : $name</p>"); echo ("<p><b>Email address</b> : $email</p>"); echo ("<p><b>Preffered class time</b> : $course</p>"); echo ("<p><b>Class info</b> : $class </p>"); echo ("<p><b>Gender</b> : $gender</p>"); echo "<p><b>Subjcts Chosen:</b><p>"; if (!empty($subject)) { echo "<ul>"; for($i = 0; $i < count($subject); $i++) { echo "<li>$subject[$i]</u/li>"; } echo "</ul>"; } } ?> </body> </html>
它将产生以下输出:
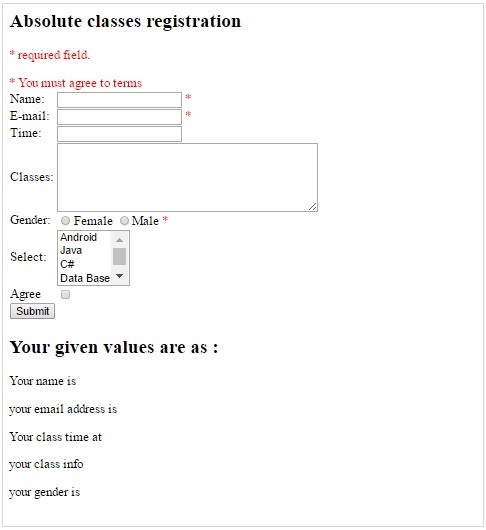
广告