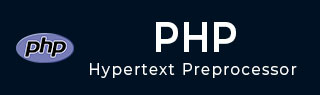
- PHP 教程
- PHP - 首页
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混杂
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc & Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期与时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 宇宙飞船运算符
- PHP 控制语句
- PHP - 决策制定
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象的 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP – 接口
- PHP - 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET & POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 净化输入
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 代码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - bug 调试
- PHP - 针对 C 开发者
- PHP - 针对 PERL 开发者
- PHP - 框架
- PHP - Core PHP vs. 框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望值
- PHP - Use 语句
- PHP - 整数除法
- PHP - 已弃用的特性
- PHP - 已移除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP – 接口
正如类是其对象的模板一样,PHP 中的接口可以被称为类的模板。我们知道,当实例化一个类时,类中定义的属性和方法都可用。同样,PHP 中的接口声明了方法及其参数和返回值。这些方法没有任何主体,即接口中没有定义任何功能。
一个具体类必须实现接口中的方法。换句话说,当一个类实现一个接口时,它必须为接口中的所有方法提供功能。
接口的定义方式与类的定义方式相同,只是用关键字“interface”代替了 class。
interface myinterface { public function myfunction(int $arg1, int $arg2); public function mymethod(string $arg1, int $arg2); }
请注意,接口内部的方法没有提供任何功能。这些方法的定义必须由实现此接口的类提供。
当我们定义子类时,我们使用关键字“extends”。在这种情况下,该类必须使用关键字“implements”。
接口中声明的所有方法都必须定义,并且参数数量和类型以及返回值必须相同。
class myclass implements myinterface { public function myfunction(int $arg1, int $arg2) { ## implementation of myfunction; } public function mymethod(string $arg1, int $arg2) { # implementation of mymethod; } }
注意,接口中声明的所有方法都必须是公共的。
示例
让我们定义一个名为shape的接口。形状有一定的面积。您有不同几何形状的形状,例如矩形、圆形等,每个形状都有一个面积,用不同的公式计算。因此,shape 接口声明了一个返回浮点值的方法 area()。
interface shape { public function area(): float; }
接下来,我们将定义一个实现 shape 接口的 circle 类,为了实现,该类必须提供接口中函数的具体实现。在这里,circle 类中的 area() 函数计算给定半径的圆的面积。
class circle implements shape { var $radius; public function __construct($arg1) { $this->radius = $arg1; } public function area(): float { return pow($this->radius,2)*pi(); } }
我们现在可以声明一个 circle 类的对象,并调用 area() 方法。
$cir = new circle(5); echo "Radius : " . $cir->radius . " Area of Circle: " . $cir->area(). PHP_EOL;
只要实现类提供了接口中每个方法的功能,任何数量的类(可能彼此无关)都可以实现一个接口。
这是一个实现 shape 的 Square 类。area() 方法返回 side 属性的平方。
class square implements shape { var $side; public function __construct($arg1) { $this->side = $arg1; } public function area(): float { return pow($this->side, 2); } }
同样,创建一个 Square 对象并调用 area() 方法。
示例
以下是 shape 接口的完整代码,由 circle 和 Square 类实现:
<?php interface shape { public function area(): float; } class square implements shape { var $side; public function __construct($arg1) { $this->side = $arg1; } public function area(): float { return pow($this->side, 2); } } class circle implements shape { var $radius; public function __construct($arg1) { $this->radius = $arg1; } public function area(): float { return pow($this->radius,2)*pi(); } } $sq = new square(5); echo "Side: " . $sq->side . " Area of Square: ". $sq->area() . PHP_EOL; $cir = new circle(5); echo "Radius: " . $cir->radius . " Area of Circle: " . $cir->area(). PHP_EOL; ?>
它将产生以下输出:
Side: 5 Area of Square: 25 Radius: 5 Area of Circle: 78.539816339745
PHP 中的多重继承
PHP 没有构建扩展两个父类的子类的规定。换句话说,语句:
class child extends parent1, parent2
不被接受。但是,PHP 确实支持具有扩展一个父类并实现一个或多个接口的子类。
让我们来看下面的例子,它展示了一个扩展另一个类并实现一个接口的类。
首先,父类 marks。它有三个实例变量或属性 $m1、$m2、$m3,分别代表三门科目的分数。提供了一个构造函数来初始化对象。
class marks { protected int $m1, $m2, $m3; public function __construct($x, $y, $z) { $this->m1 = $x; $this->m2 = $y; $this->m3 = $z; } }
现在我们提供一个名为 percent 的接口,它声明一个方法 percent(),该方法应该返回一个浮点数,但没有函数体。
interface percent { public function percent(): float; }
现在我们开发一个扩展 marks 类并为接口中的 percent() 方法提供实现的类。
class student extends marks implements percent { public function percent(): float { return ($this->m1+$this->m2+$this->m3)*100/300; } }
student 类继承了父构造函数,但提供了 parent() 方法的实现,该方法返回分数的百分比。
示例
完整的代码如下:
<?php class marks { protected int $m1, $m2, $m3; public function __construct($x, $y, $z) { $this->m1 = $x; $this->m2 = $y; $this->m3 = $z; } } interface percent { public function percent(): float; } class student extends marks implements percent { public function percent(): float { return ($this->m1+$this->m2+$this->m3)*100/300; } } $s1 = new student(50, 60, 70); echo "Percentage of marks: ". $s1->percent() . PHP_EOL; ?>
它将产生以下输出:
Percentage of marks: 60
PHP 中的接口定义了一个方法框架,类使用该框架提供他们自己不同的具体实现。