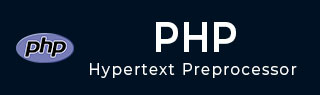
- PHP 教程
- PHP - 首页
- PHP - 路线图
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混合
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc 和 Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期和时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 比较运算符
- PHP 控制语句
- PHP - 决策制定
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - Final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET 和 POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 净化输入
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - Bug 调试
- PHP - 针对 C 开发人员
- PHP - 针对 PERL 开发人员
- PHP - 框架
- PHP - Core PHP 与框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望
- PHP - Use 语句
- PHP - 整数除法
- PHP - 已弃用功能
- PHP - 已移除扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP - 变量作用域
在 PHP 中,变量的作用域是指变量定义和可访问的上下文范围。通常,一个简单的顺序 PHP 脚本,没有循环或函数等,只有一个作用域。在“<?php”和“?>”标签内声明的任何变量,从定义点开始在整个程序中都可用。
根据作用域,PHP 变量可以是以下三种类型之一:
主脚本中的变量也可以提供给通过include或require语句包含的任何其他脚本。
示例
在以下示例中,“test.php”脚本包含在主脚本中。
main.php
<?php $var=100; include "test.php"; ?>
test.php
<?php echo "value of \$var in test.php : " . $var; ?>
当执行主脚本时,它将显示以下输出:
value of $var in test.php : 100
但是,当脚本具有用户定义的函数时,函数内部的任何变量都具有局部作用域。因此,在函数内部定义的变量无法在外部访问。在函数外部(上方)定义的变量具有全局作用域。
示例
请查看以下示例:
<?php $var=100; // global variable function myfunction() { $var1="Hello"; // local variable echo "var=$var var1=$var1" . PHP_EOL; } myfunction(); echo "var=$var var1=$var1" . PHP_EOL; ?>
它将产生以下输出:
var= var1=Hello var=100 var1= PHP Warning: Undefined variable $var in /home/cg/root/64504/main.php on line 5 PHP Warning: Undefined variable $var1 in /home/cg/root/64504/main.php on line 8
注意,全局变量不会自动在函数的局部作用域内可用。此外,函数内部的变量在外部不可访问。
“global”关键字
要启用在函数的局部作用域内访问全局变量,应使用“global”关键字显式执行此操作。
示例
PHP 脚本如下:
<?php $a=10; $b=20; echo "Global variables before function call: a = $a b = $b" . PHP_EOL; function myfunction() { global $a, $b; $c=($a+$b)/2; echo "inside function a = $a b = $b c = $c" . PHP_EOL; $a=$a+10; } myfunction(); echo "Variables after function call: a = $a b = $b c = $c"; ?>
它将产生以下输出:
Global variables before function call: a = 10 b = 20 inside function a = 10 b = 20 c = 15 Variables after function call: a = 20 b = 20 c = PHP Warning: Undefined variable $c in /home/cg/root/48499/main.php on line 12
现在可以在函数内部处理全局变量。此外,在函数内部对全局变量所做的任何更改都将反映在全局命名空间中。
$GLOBALS 数组
PHP 将所有全局变量存储在一个名为$GLOBALS的关联数组中。变量的名称和值构成键值对。
示例
在以下 PHP 脚本中,使用 $GLOBALS 数组访问全局变量:
<?php $a=10; $b=20; echo "Global variables before function call: a = $a b = $b" . PHP_EOL; function myfunction() { $c=($GLOBALS['a']+$GLOBALS['b'])/2; echo "c = $c" . PHP_EOL; $GLOBALS['a']+=10; } myfunction(); echo "Variables after function call: a = $a b = $b c = $c"; ?>
它将产生以下输出:
Global variables before function call: a = 10 b = 20 c = 15 PHP Warning: Undefined variable $c in C:\xampp\htdocs\hello.php on line 12 Variables after function call: a = 20 b = 20 c =
静态变量
使用static关键字定义的变量不会在每次调用函数时都初始化。此外,它保留了前一次调用的值。
示例
请查看以下示例:
<?php function myfunction() { static $x=0; echo "x = $x" . PHP_EOL; $x++; } for ($i=1; $i<=3; $i++) { echo "call to function :$i : "; myfunction(); } ?>
它将产生以下输出:
call to function :1 : x = 0 call to function :2 : x = 1 call to function :3 : x = 2
广告