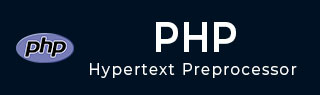
- PHP 教程
- PHP - 首页
- PHP - 路线图
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混用
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与I/O
- PHP - 数学函数
- PHP - Heredoc & Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期与时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 比较运算符(太空船运算符)
- PHP 控制语句
- PHP - 决策
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理CSV文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象的PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET & POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 过滤输入
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash 消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - Bug 调试
- PHP - 针对C开发人员
- PHP - 针对PERL开发人员
- PHP - 框架
- PHP - Core PHP vs. 框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望值
- PHP - use 语句
- PHP - 整数除法
- PHP - 已弃用的特性
- PHP - 已移除的扩展和SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP – Closure::call()
在PHP中,**闭包**是一个匿名函数,它可以访问其创建作用域中的变量,即使该作用域已关闭。需要在其中指定 use 关键字。
闭包是封装函数代码及其创建作用域的对象。在PHP 7中,引入了一个新的**Closure::call()**方法,用于将对象作用域绑定到闭包并调用它。
Closure 类中的方法
Closure 类包含以下方法,包括 call() 方法:
final class Closure { /* Methods */ private __construct() public static bind(Closure $closure, ?object $newThis, object|string|null $newScope = "static"): ?Closure public bindTo(?object $newThis, object|string|null $newScope = "static"): ?Closure public call(object $newThis, mixed ...$args): mixed public static fromCallable(callable $callback): Closure }
**call() 方法**是 Closure 类的静态方法。它作为 bind() 或 bindTo() 方法的快捷方式被引入。
**bind() 方法**复制一个具有特定绑定对象和类作用域的闭包,而 bindTo() 方法则复制一个具有新绑定对象和类作用域的闭包。
call() 方法具有以下**签名**:
public Closure::call(object $newThis, mixed ...$args): mixed
call() 方法会临时将闭包绑定到 newThis,并使用任何给定的参数调用它。
在PHP 7之前的版本中,可以使用 bindTo() 方法,如下所示:
<?php class A { private $x = 1; } // Define a closure Pre PHP 7 code $getValue = function() { return $this->x; }; // Bind a clousure $value = $getValue->bindTo(new A, 'A'); print($value()); ?>
该程序将闭包对象**$getValue**绑定到 A 类的对象,并打印其私有变量**$x**的值——它是1。
在PHP 7中,绑定是通过 call() 方法实现的,如下所示:
<?php class A { private $x = 1; } // PHP 7+ code, Define $value = function() { return $this->x; }; print($value->call(new A)); ?>
广告