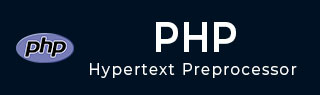
- PHP 教程
- PHP - 首页
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混杂
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc 和 Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期和时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 比较符
- PHP 控制语句
- PHP - 决策
- PHP - if…else 语句
- PHP - switch 语句
- PHP - 循环类型
- PHP - for 循环
- PHP - foreach 循环
- PHP - while 循环
- PHP - do…while 循环
- PHP - break 语句
- PHP - continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - Traits
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET 和 POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 净化输入
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 代码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - try…catch
- PHP - 调试
- PHP - 针对 C 开发人员
- PHP - 针对 PERL 开发人员
- PHP - 框架
- PHP - 核心 PHP 与框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望
- PHP - use 语句
- PHP - 整数除法
- PHP - 已弃用的特性
- PHP - 已移除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP - 文件是否存在
在对文件进行任何处理之前,先检查你尝试打开的文件是否存在通常非常有用。否则,程序可能会引发运行时异常。
PHP 的内置库在这方面提供了一些实用函数。本章将讨论的一些函数包括:
file_exists() - 测试文件是否存在
is_file() - 如果 fopen() 返回的句柄指向文件或目录。
is_readable() - 测试你打开的文件是否允许读取数据
is_writable() - 测试是否允许向文件写入数据
file_exists() 函数
此函数可用于文件和目录。它检查给定的文件或目录是否存在。
file_exists(string $filename): bool
此函数唯一的参数是表示文件/目录完整路径的字符串。函数根据文件是否存在返回 true 或 false。
示例
以下程序检查文件“hello.txt”是否存在。
<?php $filename = 'hello.txt'; if (file_exists($filename)) { $message = "The file $filename exists"; } else { $message = "The file $filename does not exist"; } echo $message; ?>
如果文件存在于当前目录中,则消息为:
The file hello.txt exists
如果不存在,则消息为:
The file hello.txt does not exist
示例
指向文件的字符串可以具有相对路径或绝对路径。假设“hello.txt”文件位于当前目录内的“hello”子目录中。
<?php $filename = 'hello/hello.txt'; if (file_exists($filename)) { $message = "The file $filename exists"; } else { $message = "The file $filename does not exist"; } echo $message; ?>
它将产生以下输出:
The file hello/hello.txt exists
示例
尝试给出如下所示的绝对路径:
<?php $filename = 'c:/xampp/htdocs/hello.txt'; if (file_exists($filename)) { $message = "The file $filename exists"; } else { $message = "The file $filename does not exist"; } echo $message; ?>
它将产生以下输出:
The file c:/xampp/htdocs/hello.txt exists
is_file() 函数
file_exists() 函数对现有文件和目录都返回true。is_file() 函数可帮助你确定它是否是文件。
is_file ( string $filename ) : bool
以下示例显示了is_file() 函数的工作方式:
<?php $filename = 'hello.txt'; if (is_file($filename)) { $message = "$filename is a file"; } else { $message = "$filename is a not a file"; } echo $message; ?>
输出表明它是一个文件:
hello.txt is a file
现在,将“$filename”更改为目录,然后查看结果:
<?php $filename = hello; if (is_file($filename)) { $message = "$filename is a file"; } else { $message = "$filename is a not a file"; } echo $message; ?>
现在你会被告知“hello”不是文件。
请注意,is_file() 函数接受一个$filename,并且仅当$filename 是文件且存在时才返回true。
is_readable() 函数
有时,你可能想事先检查是否可以读取文件。is_readable() 函数可以确定这一事实。
is_readable ( string $filename ) : bool
示例
以下是is_readable() 函数工作方式的示例:
<?php $filename = 'hello.txt'; if (is_readable($filename)) { $message = "$filename is readable"; } else { $message = "$filename is not readable"; } echo $message; ?>
它将产生以下输出:
hello.txt is readable
is_writable() 函数
你可以使用 is_writable() 函数来检查文件是否存在以及是否可以对给定文件执行写入操作。
is_writable ( string $filename ) : bool
示例
以下示例显示了is_writable() 函数的工作方式:
<?php $filename = 'hello.txt'; if (is_writable($filename)) { $message = "$filename is writable"; } else { $message = "$filename is not writable"; } echo $message; ?>
对于普通的存档文件,程序会指出它是可写的。但是,将其属性更改为“只读”并运行程序。现在你得到:
hello.txt is writable