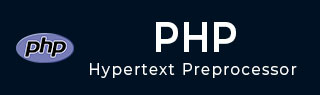
- PHP 教程
- PHP - 首页
- PHP - 路线图
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混淆
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc 和 Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期与时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 比较符
- PHP 控制语句
- PHP - 决策制定
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象的 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - Final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET 和 POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 对输入进行清理
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - Bug 调试
- PHP - 适用于 C 开发人员
- PHP - 适用于 PERL 开发人员
- PHP - 框架
- PHP - 核心 PHP 与框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望
- PHP - Use 语句
- PHP - 整数除法
- PHP - 已弃用的功能
- PHP - 已删除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP Try…Catch
在 PHP 中,try、catch、throw 和 finally 关键字用于处理异常。而错误是意外的程序结果,程序本身无法处理,程序必须使用 die() 或设置自定义错误处理程序来终止。
另一方面,异常是指意外情况,可以对其进行处理,以便程序在将其抛出其正常流程后继续运行。
异常可以被抛出,并使用 PHP 代码中的 catch 关键字捕获。可能发生异常的代码块用 try 块包围。每个 try 必须至少有一个对应的 catch 或 finally 块。
Try、Throw、Catch 和 Finally
这四个与异常相关的关键字具有以下作用:
Try - 将可能发生某些异常的代码块放在“try”块中。如果未触发异常,则代码继续执行。但是,如果确实发生了异常,则会“抛出”。执行将停止,PHP 将寻找匹配的“catch”块。如果未捕获异常,则 PHP 会发出致命错误。
Throw - 这是触发异常的方法。每个“throw”必须至少有一个“catch”或“finally”块。
Catch - 一个块,它检索异常并创建一个包含异常信息的
对象
。可以使用多个 catch 块来捕获不同的异常。Finally - finally 块中的代码始终在 throw 或 catch 块之后执行。
示例
这是一个异常处理技术的示例。代码在浏览器上呈现两个文本字段,并要求用户输入两个数字进行除法运算。如果第二个数字(分母)为 0,则会抛出异常,程序进入 catch 块并打印异常消息。否则,将显示除法的结果。
<html> <body> <form action="<?php echo $_SERVER['PHP_SELF'];?>" method="post"> <h3>First No: <input type="text" name="first"/></h3> <h3>Second No: <input type="text" name="second"/></h3> <input type="submit" value="Submit" /> </form> <?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $x = $_POST['first']; $y = $_POST['second']; echo "$x $y"; try { if ($y == 0) { throw new Exception("Division by Zero"); } $z = $x/$y; echo "<h3>x = $x y = $y Division = $z<br>"; } catch (Exception $e) { echo "<h3> Exception: " . $e->getMessage(); } } ?> </body> </html>
它将产生以下输出:
Case 1: x = 10 y = 5 Division = 2 Case 2: x = 10 y = 0 Exception: Division by Zero
异常类
PHP 抛出一个Exception 类的对象。在 PHP 中,Exception 类是用户异常的基础。它实现了 throwable 接口。
此类定义了以下方法:
getMessage()
此函数将异常消息作为字符串返回:
final public Exception::getMessage(): string
getCode()
此函数在 Exception 中将异常代码作为int返回:
final public Exception::getCode(): int
请查看以下示例:
try { throw new Exception("Some error message", 30); } catch(Exception $e) { echo "The exception code is: " . $e->getCode(); }
getFile()
此函数返回创建异常的文件名:
final public Exception::getFile(): string
请查看以下示例:
try { if ($y == 0) { throw new Exception("Division by Zero"); } $z = $x/$y; echo "<h3>x = $x y = $y Division = $z<br>"; } catch (Exception $e) { echo "<h3> Exception: " . $e->getMessage(). " in " . $e->getFile(); }
它将产生以下输出:
Exception: Division by Zero in C:\xampp\htdocs\hello.php
getLine()
此函数返回创建异常的行号:
final public Exception::getLine(): int
示例
请查看以下示例:
<?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $x = $_POST['first']; $y = $_POST['second']; echo "$x $y"; try { if ($y == 0) { throw new Exception("Division by Zero"); } $z = $x/$y; echo "<h3>x = $x y = $y Division = $z<br>"; } catch (Exception $e) { echo "<h3> Exception: " . $e->getMessage(). " in " . $e->getLine() . " of " . $e->getFile(); } } ?>
它将产生以下输出:
Exception: Division by Zero in 21 of C:\xampp\htdocs\hello.php
多个 Catch 块
PHP 允许在 try 块之后使用一系列 catch 块来处理不同的异常情况。可以使用多个catch块来处理预定义的异常和错误以及用户定义的异常。
示例
以下示例使用catch块来处理 DivisioByZeroError、TypeError、ArgumentCountError 和 InvalidArgumentException 条件。还有一个catch块用于处理通用 Exception。
<?php declare(strict_types=1); function divide(int $a, int $b) : int { return $a / $b; } $a=10; $b=0; try { if (!$b) { throw new DivisionByZeroError('Division by zero.'); if (is_int($a)==FALSE || is_int($b)==FALSE) throw new InvalidArgumentException("Invalid type of arguments"); $result=divide($a, $b); echo $result; } // if argument types not matching catch (TypeError $x) { echo $x->getMessage(); } // if denominator is 0 catch (DivisionByZeroError $y) { echo $y->getMessage(); } // if number of arguments not equal to 2 catch (ArgumentCountError $z) { echo $z->getMessage(); } // if argument types not matching catch (InvalidArgumentException $i) { echo $i->getMessage(); } // any uncaught exception catch (Exception $ex) { echo $ex->getMessage(); } ?>
首先,由于分母为 0,因此将显示“除以 0”错误:
Division by 0
设置$b=3,这将导致 TypeError,因为 divide 函数预期返回整数,但除法结果为float。
divide(): Return value must be of type int, float returned
如果只将一个变量传递给 divide 函数,通过更改$res=divide($a); 这将导致ArgumentCountError:
Too few arguments to function divide(), 1 passed in C:\xampp\htdocs\hello.php on line 16 and exactly 2 expected
如果其中一个参数不是整数,则为InvalidArgumentException的情况。将$b更改为字符串:
Invalid type of arguments
Finally 块
除了catch块之外,还可以指定finally块,或者用finally块代替catch块。无论是否抛出异常,finally块中的代码都将在try和catch块之后执行,并在正常执行恢复之前执行。
try { if ($y == 0) { throw new Exception("Division by Zero"); } $z = $x/$y; echo "<h3>x = $x y = $y Division = $z </h3><br>"; } catch (Exception $e) { echo "<h3> Exception: " . $e->getMessage(). "</h3>"; } finally { echo "<h3>End of try - catch - finally</h3>"; }
它将产生以下输出:
情况1:
x = 10 y = 5 Division = 2 End of try - catch – finally
情况2:
X=10 y=0 Exception: Division by Zero End of try - catch – finally
带有返回语句的Finally
当try块或catch块(或两者)包含return语句时,finally块的行为比较特殊。通常,return语句会导致程序控制权返回到调用位置。但是,在包含try/catch块且带有return语句的函数中,finally块中的语句将在返回之前首先执行。
示例
在下面的示例中,div() 函数具有“try-catch-finally”结构。try块在没有异常的情况下返回除法的结果。如果发生异常,catch块将返回错误消息。但是,在这两种情况下,finally块中的语句都会首先执行。
<?php function div($x, $y) { try { if ($y==0) throw new Exception("Division by 0"); else $res=$x/$y;; return $res; } catch (Exception $e) { return $e->getMessage(); } finally { echo "This block is always executed\n"; } } $x=10; $y=0; echo div($x,$y); ?>
它将产生以下输出:
This block is always executed Division by 0