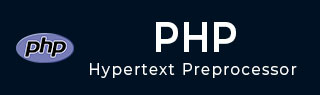
- PHP 教程
- PHP - 首页
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混淆
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc 和 Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期与时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 键值比较运算符
- PHP 控制语句
- PHP - 决策
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - Final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET 和 POST
- PHP - 文件上传
- PHP - Cookies
- PHP - Sessions
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 净化输入
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - Bug 调试
- PHP - 针对 C 开发人员
- PHP - 针对 PERL 开发人员
- PHP - 框架
- PHP - Core PHP 与框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP – 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 预期
- PHP - Use 语句
- PHP - 整数除法
- PHP - 已弃用的功能
- PHP - 已删除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP – 系统调用
PHP 的内置函数库包含一类用于在 PHP 代码中调用操作系统实用程序和外部程序的函数。在本章中,我们将讨论用于执行系统调用的 PHP 函数。
system() 函数
system() 函数类似于 C 中的 system() 函数,它执行给定的命令并输出结果。
system(string $command, int &$result_code = null): string|false
如果 PHP 作为服务器模块运行,则 system() 调用尝试在每行输出后自动刷新 Web 服务器的输出缓冲区。成功时返回命令输出的最后一行,失败时返回 false。
示例
以下 PHP 代码片段调用 Windows 操作系统的 DIR 命令,并显示当前目录中的文件列表。
<?php echo '<pre>'; // Outputs all the result of DOS command "dir", and returns // the last output line into $last_line. Stores the return value // of the shell command in $retval. $last_line = system('dir/w', $retval); // Printing additional info echo ' </pre> <hr />Last line of the output: ' . $last_line . ' <hr />Return value: ' . $retval; ?>
它将产生以下输出 -
Volume in drive C has no label. Volume Serial Number is 7EE4-E492 Directory of C:\xampp\htdocs [.] [..] applications.html bitnami.css [dashboard] employee.csv favicon.ico hello.csv hello.html hello.php homepage.php [img] index.php [Langi] menu.php myform.php myname.php new.png new.txt test.php test.zip [TPcodes] uploadfile.php [webalizer] welcome.png [xampp] 18 File(s) 123,694 bytes 8 Dir(s) 168,514,232,320 bytes free Last line of the output: 8 Dir(s) 168,514,232,320 bytes free Return value: 0
shell_exec() 函数
shell_exec() 函数与 PHP 的反引号运算符相同。它通过 shell 执行给定的命令,并将完整的输出作为字符串返回。
shell_exec(string $command): string|false|null
该函数返回一个包含已执行命令输出的字符串,如果管道无法建立则返回 false,如果发生错误或命令没有产生输出则返回 null。
示例
在以下代码中,我们使用 shell_exec() 函数在当前目录中获取扩展名为“.php”的文件列表 -
<?php $output = shell_exec('dir *.php'); echo "<pre>$output</pre>"; ?>
它将产生以下输出 -
Volume in drive C has no label. Volume Serial Number is 7EE4-E492 Directory of C:\xampp\htdocs 10/26/2023 08:27 PM 73 hello.php 10/12/2023 10:40 AM 61 homepage.php 07/16/2015 09:02 PM 260 index.php 10/12/2023 10:39 AM 49 menu.php 09/25/2023 01:43 PM 338 myform.php 10/12/2023 10:49 AM 51 myname.php 10/26/2023 02:00 PM 369 test.php 09/25/2023 01:42 PM 555 uploadfile.php 8 File(s) 1,756 bytes 0 Dir(s) 168,517,771,264 bytes free
exec() 函数
exec() 函数将给定的命令作为字符串参数执行。
exec(string $command, array &$output = null, int &$result_code = null):string|false
如果指定了$output 参数,则它是一个数组,其中将填充来自命令的每一行输出。
示例
在这种情况下,我们使用 exec() 函数从程序内部调用 whoami 命令。whoami 命令返回用户名。
<?php // outputs the username that owns the running php/httpd process // (on a system with the "whoami" executable in the path) $output=null; $retval=null; exec('whoami', $output, $retval); echo "Returned with status $retval and output:\n"; var_dump($output); ?>
它将产生以下输出 -
Returned with status 0 and output: array(1) { [0]=> string(13) "gnvbgl3\mlath" }
passthru() 函数
passthru() 函数执行外部程序并显示原始输出。尽管 passthru() 函数类似于 exec() 或 system() 函数,因为它执行命令,但当来自 OS 命令的输出是需要直接传递回浏览器的二进制数据时,应该使用它代替它们。
示例
一个使用 passthu() 函数显示系统 PATH 环境变量内容的 PHP 程序
passthru(string $command, int &$result_code = null): ?false <?php passthru ('PATH'); ?>
它将产生以下输出 -
PATH=C:\Python311\Scripts\;C:\Python311\;C:\WINDOWS\system32;C:\WINDOWS; C:\WINDOWS\System32\Wbem;C:\WINDOWS\System32\WindowsPowerShell\v1.0\; C:\WINDOWS\System32\OpenSSH\;C:\xampp\php;C:\Users\mlath\AppData\Local \Microsoft\WindowsApps;C:\VSCode\Microsoft VS Code\bin
反引号运算符
PHP 支持一个执行运算符:反引号 (``)。(它们不是单引号!)PHP 将尝试将反引号中的内容作为 shell 命令执行;将返回输出。反引号运算符的使用与 shell_exec() 相同。
示例
看看下面的例子 -
<?php $output = `dir *.php`; echo "<pre>$output</pre>"; ?>
它将产生以下输出 -
Volume in drive C has no label. Volume Serial Number is 7EE4-E492 Directory of C:\xampp\htdocs 10/26/2023 08:42 PM 61 hello.php 10/12/2023 10:40 AM 61 homepage.php 07/16/2015 09:02 PM 260 index.php 10/12/2023 10:39 AM 49 menu.php 09/25/2023 01:43 PM 338 myform.php 10/12/2023 10:49 AM 51 myname.php 10/26/2023 02:00 PM 369 test.php 09/25/2023 01:42 PM 555 uploadfile.php 8 File(s) 1,744 bytes 0 Dir(s) 168,471,289,856 bytes free
当shell_exec() 被禁用时,反引号运算符也被禁用。