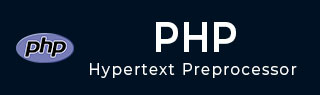
- PHP 教程
- PHP - 首页
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混合
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc 和 Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期和时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 比较运算符(太空船运算符)
- PHP 控制语句
- PHP - 决策
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - Final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET 和 POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 过滤输入
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - Bug 调试
- PHP - 针对 C 开发人员
- PHP - 针对 PERL 开发人员
- PHP - 框架
- PHP - Core PHP 与框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望
- PHP - Use 语句
- PHP - 整数除法
- PHP - 已弃用的特性
- PHP - 已删除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP - 登录示例
一个典型的 PHP web 应用程序在登录前会对用户进行身份验证,方法是询问用户的凭据,例如用户名和密码。然后将这些凭据与服务器上可用的用户数据进行核对。在本示例中,用户数据以关联数组的形式提供。以下 PHP 登录脚本进行了说明 -
HTML 表单
代码的 HTML 部分显示了一个简单的 HTML 表单,该表单接受用户名和密码,并将数据发布到自身。
<form action = "<?php echo htmlspecialchars($_SERVER['PHP_SELF']); ?>" method="post"> <div> <label for="username">Username:</label> <input type="text" name="username" id="name"> </div> <div> <label for="password">Password:</label> <input type="password" name="password" id="password"> </div> <section style="margin-left:2rem;"> <button type="submit" name="login">Login</button> </section> </form>
PHP 身份验证
PHP 脚本解析 POST 数据,并检查 users 数组中是否存在用户名。如果找到,它将进一步检查密码是否与数组中注册的用户相对应。
<?php if (array_key_exists($user, $users)) { if ($users[$_POST['username']]==$_POST['password']) { $_SESSION['valid'] = true; $_SESSION['timeout'] = time(); $_SESSION['username'] = $_POST['username']; $msg = "You have entered correct username and password"; } else { $msg = "You have entered wrong Password"; } } else { $msg = "You have entered wrong user name"; } ?>
用户名和相应的消息将添加到 $_SESSION 数组中。系统会提示用户输入相应的邮件,告知他输入的凭据是否正确。
完整代码
以下是完整代码 -
Login.php
<?php ob_start(); session_start(); ?> <html lang = "en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="loginstyle.css"> <title>Login</title> </head> <body> <h2 style="margin-left:10rem; margin-top:5rem;">Enter Username and Password</h2> <?php $msg = ''; $users = ['user'=>"test", "manager"=>"secret", "guest"=>"abc123"]; if (isset($_POST['login']) && !empty($_POST['username']) && !empty($_POST['password'])) { $user=$_POST['username']; if (array_key_exists($user, $users)){ if ($users[$_POST['username']]==$_POST['password']){ $_SESSION['valid'] = true; $_SESSION['timeout'] = time(); $_SESSION['username'] = $_POST['username']; $msg = "You have entered correct username and password"; } else { $msg = "You have entered wrong Password"; } } else { $msg = "You have entered wrong user name"; } } ?> <h4 style="margin-left:10rem; color:red;"><?php echo $msg; ?></h4> <br/><br/> <form action = "<?php echo htmlspecialchars($_SERVER['PHP_SELF']); ?>" method="post"> <div> <label for="username">Username:</label> <input type="text" name="username" id="name"> </div> <div> <label for="password">Password:</label> <input type="password" name="password" id="password"> </div> <section style="margin-left:2rem;"> <button type="submit" name="login">Login</button> </section> </form> <p style="margin-left: 2rem;"> <a href = "logout.php" tite = "Logout">Click here to clean Session.</a> </p> </div> </body> </html>
Logout.php
要注销,用户可以点击链接到logout.php
<?php session_start(); unset($_SESSION["username"]); unset($_SESSION["password"]); echo '<h4>You have cleaned session</h4>'; header('Refresh: 2; URL = login.php'); ?>
输入“https://127.0.0.1/login.php”启动应用程序。以下是不同的场景 -
正确的用户名和密码
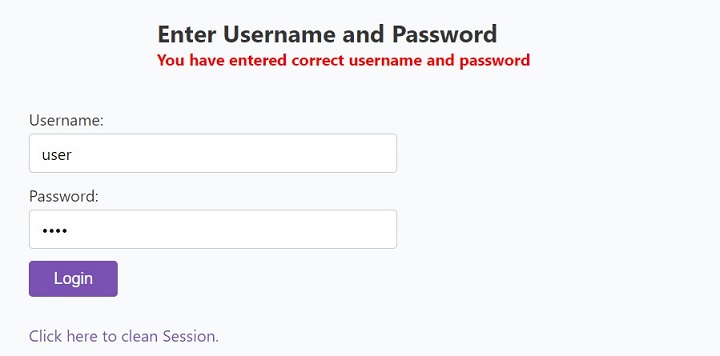
密码错误
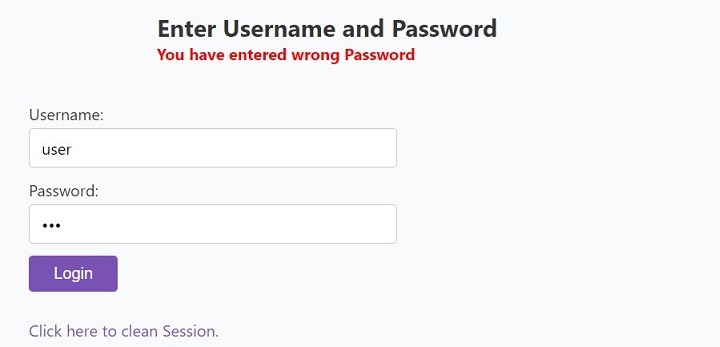
用户名错误
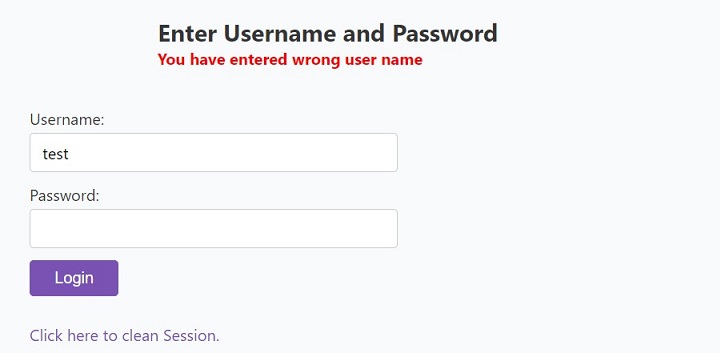
当用户点击底部的链接时,会话变量将被删除,并重新显示登录屏幕。
广告