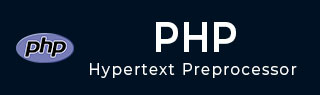
- PHP 教程
- PHP - 首页
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混合
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc 和 Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期与时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 比较符
- PHP 控制语句
- PHP - 决策制定
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象的 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - Final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET 和 POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 清理输入
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - Bug 调试
- PHP - 针对 C 开发人员
- PHP - 针对 PERL 开发人员
- PHP - 框架
- PHP - Core PHP 与框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望
- PHP - Use 语句
- PHP - 整数除法
- PHP - 已弃用的特性
- PHP - 已移除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP - 表单验证
术语“表单验证”指的是确定用户在各种表单元素中输入的数据是否可以用于进一步处理的过程。在后续处理之前验证数据可以避免可能的异常和运行时错误。
验证可以在客户端和服务器端进行。当客户端提交表单时,表单数据会被服务器上运行的 PHP 脚本拦截。使用 PHP 中提供的各种函数,可以完成服务器端的表单验证。
客户端验证
根据 HTML5 规范,新的输入控件具有内置验证功能。例如,类型为“email”的输入元素,即使是文本字段,也经过定制以接受符合电子邮件地址协议的字符串。
验证发生在数据提交到服务器之前。对于其他输入类型,如 URL、数字等,也是如此。
示例
下面是一个 HTML 表单,其中包含数字类型、电子邮件类型和 URL 类型的输入元素。如果您输入与所需格式不符的数据,则在尝试提交表单时会显示相应的错误消息。
<h1>Input Validation</h1> <form> <p><Label for "name">Enter your name</label> <input type = "text" id="name" name="name"></p> <p><label for="age">Enter age</label> <input type = "text" id = "age" name="age"></p> <p><label for="email">Enter your email:</label> <input type="text" id="email" name="email"></p> <p><label for="URL">Enter your website<label> <input type = "text" id="URL" name="url"></p> <input type="submit"> </form>
数字类型的文本字段在右侧显示上下计数箭头。只接受数字,并且可以递增或递减。
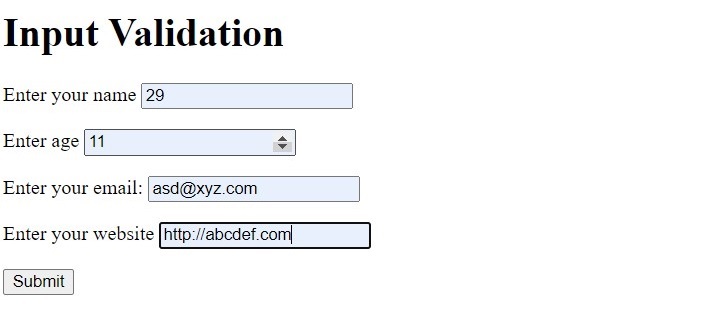
如果电子邮件字段中的数据无效,您会看到如下所示的错误消息。
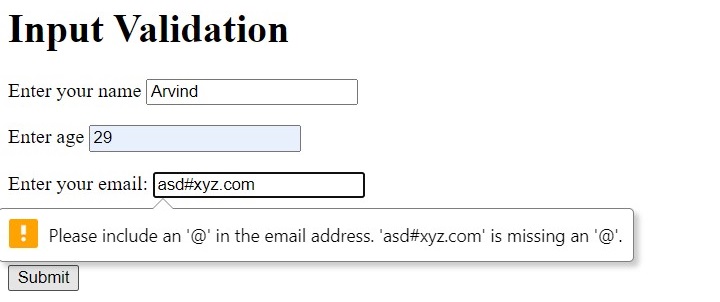
类似地,URL 的任何不正确格式也会显示如下所示的错误 -
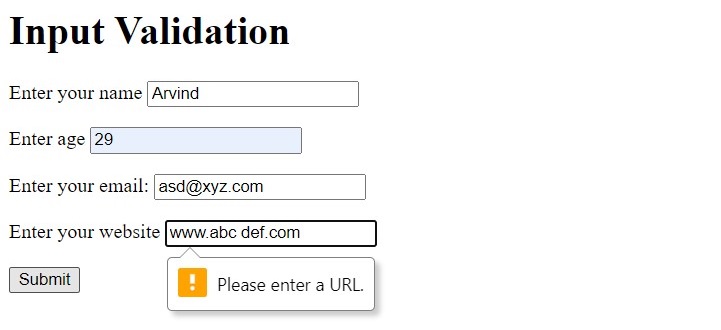
验证函数
使用 PHP 进行服务器端验证的情况出现在表单数据通过客户端验证时,或者根本没有客户端验证时。
在上例中使用的 HTML 表单中,让我们删除所有特殊输入类型,并使用所有文本类型的文本字段。表单使用 POST 方法提交到服务器上的 hello.php。
<form action="hello.php" method="POST"> <p><Label for "name">Enter your name</label> <input type = "text" id="name" name="name"></p> <p><label for="age">Enter age</label> <input type = "text" id = "age" name="age"></p> <p><label for="email">Enter your email:</label> <input type="text" id="email" name="email"></p> <p><label for="URL">Enter your website<label> <input type = "text" id="URL" name="url"></p> <input type="submit"> </form>
表单为空
如果用户(可能是无意地)点击提交按钮,您可以要求 PHP 再次显示表单。您需要检查 $_POST 数组是否已使用 isset() 函数初始化。如果没有,header() 函数会将控制权重新定向回表单。
<?php if ($_SERVER["REQUEST_METHOD"] == "POST") { if (isset($_POST)) { header("Location: hello.html", true, 301); exit(); } // form processing if the form is not empty } ?>
示例
您还可以检查在提交表单时是否有任何字段为空。
<?php if ($_SERVER["REQUEST_METHOD"] == "POST") { foreach($_POST as $k=>$v) { if (empty($v)==true) { echo "One or more fields are empty \n"; echo "<a href = 'hello.html'>Click here to go back </a>"; exit; } else echo "$k => $v \n"; } } ?>
年龄字段不是数字
在 HTML 表单中,名称的输入字段为文本类型,因此它可以接受任何字符。但是,我们希望它为数字。这可以通过 is_numeric() 函数来确保。
<?php if (is_numeric($_POST["age"])==false) { echo "Age cannot be non-numeric \n"; echo "<a href = 'hello.html'>Click here to go back</a>"; } ?>
PHP 还有 is_string() 函数来检查字段是否包含字符串。另外两个函数 trim() 和 htmlspecialchars() 也对表单验证很有用。
trim() - 从字符串开头和结尾删除空白字符
htmlspecialchars() - 将特殊字符转换为 HTML 实体,以防止跨站点脚本 (XSS) 攻击。